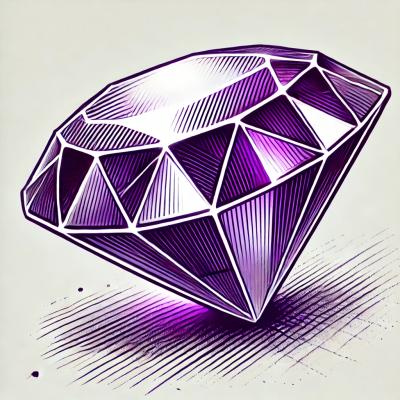
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
postman-collection-transformer
Advanced tools
Perform rapid conversation and validation of JSON structure between Postman Collection Format v1 and v2
The postman-collection-transformer npm package is a utility library for transforming Postman collections between different versions and formats. It allows users to convert collections to and from various Postman schema versions, making it easier to manage and maintain API collections.
Convert Collection to Latest Version
This feature allows you to convert a Postman collection from an older version (e.g., 1.0.0) to the latest version (e.g., 2.1.0). This is useful for updating collections to be compatible with the latest Postman features.
const transformer = require('postman-collection-transformer');
const collectionV1 = { /* V1 collection JSON */ };
transformer.convert(collectionV1, { inputVersion: '1.0.0', outputVersion: '2.1.0' }, (err, collectionV2) => {
if (err) { console.error(err); }
else { console.log(collectionV2); }
});
Convert Collection to Specific Version
This feature allows you to convert a Postman collection from a newer version (e.g., 2.1.0) to an older version (e.g., 1.0.0). This can be useful for backward compatibility with tools or environments that only support older versions.
const transformer = require('postman-collection-transformer');
const collectionV2 = { /* V2 collection JSON */ };
transformer.convert(collectionV2, { inputVersion: '2.1.0', outputVersion: '1.0.0' }, (err, collectionV1) => {
if (err) { console.error(err); }
else { console.log(collectionV1); }
});
Validate Collection
This feature allows you to validate a Postman collection against a specific version schema. It helps ensure that the collection conforms to the expected structure and format.
const transformer = require('postman-collection-transformer');
const collection = { /* collection JSON */ };
transformer.validate(collection, { version: '2.1.0' }, (err, result) => {
if (err) { console.error(err); }
else { console.log(result); }
});
The postman-collection package provides a set of utilities for working with Postman collections, including creating, manipulating, and validating collections. While it offers some transformation capabilities, it is more focused on general collection management compared to the specialized transformation features of postman-collection-transformer.
The swagger-jsdoc package allows you to generate Swagger (OpenAPI) documentation from JSDoc comments in your code. While it is not specifically for Postman collections, it serves a similar purpose of transforming API documentation formats. It is more focused on generating documentation rather than transforming between different collection versions.
The openapi-to-postmanv2 package converts OpenAPI (Swagger) definitions to Postman collections. It is similar to postman-collection-transformer in that it deals with transforming API documentation formats, but it specifically focuses on converting OpenAPI specs to Postman collections rather than transforming between different Postman collection versions.
Perform rapid conversion of JSON structure between Postman Collection Format v1 and v2.
The formats are documented at https://schema.getpostman.com
For CLI usage:
$ npm install -g postman-collection-transformer
As a library:
$ npm install --save postman-collection-transformer
The transformer provides a Command line API to convert collections.
Example:
$ transform-collection convert \
--input ./v1-collection.json \
--input-version 2.0.0 \
--output ./v2-collection.json \
--output-version 1.0.0 \
--pretty \
--overwrite
All options:
$ transform-collection convert -h
Usage: convert [options]
Convert Postman Collection from one format to another
Options:
-h, --help output usage information
-i, --input <path> path to the input postman collection file
-j, --input-version [version] the version of the input collection format standard (v1 or v2)
-o, --output <path> target file path where the converted collection will be written
-p, --output-version [version] required version to which the collection is needed to be converted to
-P, --pretty Pretty print the output
--retain-ids Retain the request and folder IDs during conversion (collection ID is always retained)
-w, --overwrite Overwrite the output file if it exists
If you'd rather use the transformer as a library:
var transformer = require('postman-collection-transformer'),
collection = require('./path/to/collection.json'),
inspect = require('util').inspect,
options = {
inputVersion: '1.0.0',
outputVersion: '2.0.0',
retainIds: true // the transformer strips request-ids etc by default.
};
transformer.convert(collection, options, function (error, result) {
if (error) {
return console.error(error);
}
// result <== the converted collection as a raw Javascript object
console.log(inspect(result, {colors: true, depth: 10000}));
});
The transformer also allows you to convert individual requests (only supported when used as a library):
var transformer = require('postman-collection-transformer'),
objectToConvert = { /* A valid collection v1 Request or a collection v2 Item */ },
options = {
inputVersion: '1.0.0',
outputVersion: '2.0.0',
retainIds: true // the transformer strips request-ids etc by default.
};
transformer.convertSingle(objectToConvert, options, function (err, converted) {
console.log(converted);
});
You can convert individual responses too if needed:
var transformer = require('postman-collection-transformer'),
objectToConvert = { /* A v1 Response or a v2 Response */ },
options = {
inputVersion: '1.0.0',
outputVersion: '2.0.0',
retainIds: true // the transformer strips request-ids etc by default.
};
transformer.convertResponse(objectToConvert, options, function (err, converted) {
console.log(converted);
});
The transformer also provides a Command line API to normalize collections for full forward compatibility.
Example:
$ transform-collection normalize \
--input ./v1-collection.json \
--normalize-version 1.0.0 \
--output ./v1-norm-collection.json \
--pretty \
--overwrite
All options:
$ transform-collection normalize -h
Usage: normalize [options]
Normalizes a postman collection according to the provided version
Options:
-i, --input <path> Path to the collection JSON file to be normalized
-n, --normalize-version <version> The version to normalizers the provided collection on
-o, --output <path> Path to the target file, where the normalized collection will be written
-P, --pretty Pretty print the output
--retain-ids Retain the request and folder IDs during conversion (collection ID is always retained)
-w, --overwrite Overwrite the output file if it exists
-h, --help Output usage information
If you'd rather use the transformer as a library:
var transformer = require('postman-collection-transformer'),
collection = require('./path/to/collection.json'),
inspect = require('util').inspect,
options = {
normalizeVersion: '1.0.0',
mutate: false, // performs in-place normalization, false by default.
noDefaults: false, // when set to true, sensible defaults for missing properties are skipped. Default: false
prioritizeV2: false, // when set to true, v2 attributes are used as the source of truth for normalization.
retainEmptyValues: false, // when set to true, empty values are set to '', not removed. False by default.
retainIds: true // the transformer strips request-ids etc by default.
};
transformer.normalize(collection, options, function (error, result) {
if (error) {
return console.error(error);
}
// result <== the converted collection as a raw Javascript object
console.log(inspect(result, {colors: true, depth: 10000}));
});
FAQs
Perform rapid conversation and validation of JSON structure between Postman Collection Format v1 and v2
The npm package postman-collection-transformer receives a total of 114,866 weekly downloads. As such, postman-collection-transformer popularity was classified as popular.
We found that postman-collection-transformer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.