Polyglot i18n provider for react-admin
Polyglot i18n provider for react-admin, the frontend framework for building admin applications on top of REST/GraphQL services. It relies on polyglot.js, which uses JSON files for translations.
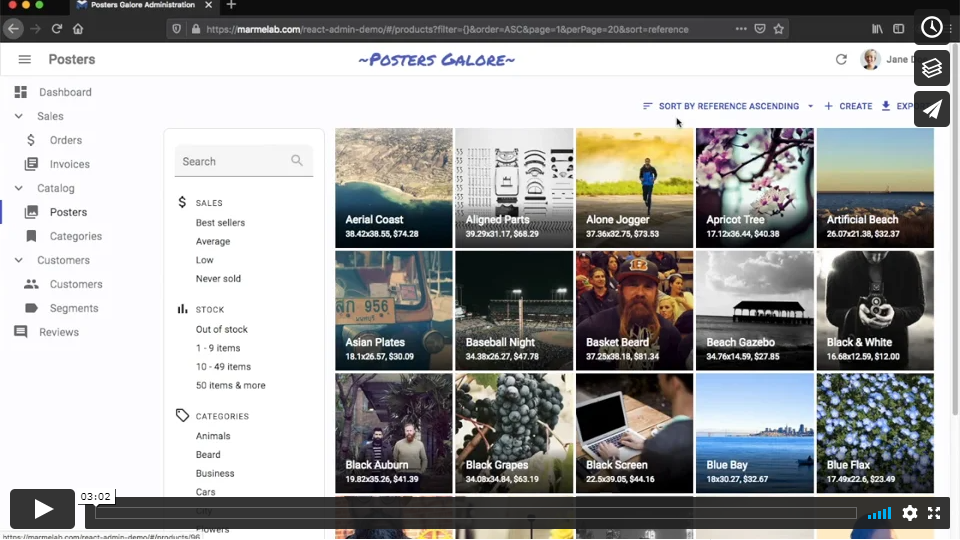
Installation
npm install --save ra-i18n-polyglot
Usage
Wrap the function exported by this package around a function returning translation messages based on a locale to produce a valid i18nProvider
.
import * as React from "react";
import { Admin, Resource } from 'react-admin';
import polyglotI18nProvider from 'ra-i18n-polyglot';
import englishMessages from 'ra-language-english';
import frenchMessages from 'ra-language-french';
const messages = {
fr: frenchMessages,
en: englishMessages,
};
const i18nProvider = polyglotI18nProvider(locale => messages[locale]);
const App = () => (
<Admin locale="en" i18nProvider={i18nProvider}>
...
</Admin>
);
export default App;
Translation Messages
The message
returned by the function argument should be a dictionary where the keys identify interface components, and values are the translated string. This dictionary is a simple JavaScript object looking like the following:
{
ra: {
action: {
delete: 'Delete',
show: 'Show',
list: 'List',
save: 'Save',
create: 'Create',
edit: 'Edit',
cancel: 'Cancel',
},
...
},
}
All core translations are in the ra
namespace, in order to prevent collisions with your own custom translations. The root key used at runtime is determined by the value of the locale
prop.
The default messages are available here.
Asynchronous Locale Change
The function passed as parameter of polyglotI18nProvider
can return a Promise for messages instead of a messages object. This lets you lazy load messages upon language change.
Note that the messages for the default locale (used by react-admin for the initial render) must be returned in a synchronous way.
import polyglotI18nProvider from 'ra-i18n-polyglot';
import englishMessages from 'ra-language-english';
const asyncMessages = {
fr: () => import('ra-language-french').then(messages => messages.default),
it: () => import('ra-language-italian').then(messages => messages.default),
};
const messagesResolver = locale => {
if (locale === 'en') {
return englishMessages;
}
return asyncMessages[params.locale]();
}
const i18nProvider = polyglotI18nProvider(messagesResolver);
Using Specific Polyglot Features
Polyglot.js is a fantastic library: in addition to being small, fully maintained, and totally framework agnostic, it provides some nice features such as interpolation and pluralization, that you can use in react-admin.
const messages = {
'hello_name': 'Hello, %{name}',
'count_beer': 'One beer |||| %{smart_count} beers',
};
translate('hello_name', { name: 'John Doe' });
=> 'Hello, John Doe.'
translate('count_beer', { smart_count: 1 });
=> 'One beer'
translate('count_beer', { smart_count: 2 });
=> '2 beers'
translate('not_yet_translated', { _: 'Default translation' });
=> 'Default translation'
To find more detailed examples, please refer to http://airbnb.io/polyglot.js/
v5.0.0
This major release introduces new features and some breaking changes. Here are the highlights:
UI Improvements
- Apps now have a theme switcher and a dark theme by default (#9479)
- Inputs now default to full width (#9704)
- Links are now underlined (#9483)
- List pages restore scroll position when coming back from Edit and Create views (#9774)
- Errors in the Layout code now trigger the Error Boundary (#9799)
- Button size can be set via props (#9735)
App Initialization
- Simpler custom layout components just need to render their children (#9591)
- No more props drilling for Layout, AppBar, Menu, etc (#9591)
- useDefaultTitle() hook returns the application title from anywhere in the app (#9591)
Data Providers
- Data providers can now cancel queries for unmounted components (opt-in) (#9612)
- GraphQL data providers are easier to initialize (they are now synchronous) (#9820)
- GraphQL-Simple data provider supports Sparse Fields in queries (#9392)
- GraphQL-Simple data provider supports updateMany and deleteMany mutations (#9393)
- withLifecycleCallbacks now supports for wildcard and array of callbacks (#9577)
- Middlewares are more powerful and handle errors better (#9875)
List pages
- Datagrid has rowClick enabled by default, it links to the edit or show view depending on the resource definition (#9466)
- List bulkActionButtons is now a Datagrid prop (#9707)
- setFilters doesn't debounce by default, so custom filters work as expected (#9682)
- List parameters persistence in the store can be disabled (#9742)
Forms & Inputs
- Inputs no longer require to be touched to display a validation error (#9781)
- ReferenceInputs are now smarter by default as they use the recordRepresentation (#9902)
- Server-Side validation is now more robust (#9848)
- warnWhenUnsavedChanges works again (#9657)
- Smart input components like TranslatableInputs, ArrayInput, or ReferenceManyInput now compose more seamlessly thanks to a new SourceContext. There is no need for getSource in FormDataConsumer. (#9533)
- All inputs now have a unique ID - no more duplicate ID warnings (#9788)
- Learning Forms is facilitated by a new Form chapter in the doc (#9864)
DX Improvements
- The default Record Representation for resources is now smarter (#9650)
- Data provider hooks like useGetOne have a smart return type based on the request state. This will force you to plan for the error case. (#9743)
- Stricter TypeScript types will detect more errors at compile time (#9741)
- PropTypes are gone, so there is no conflict with TypeScript types (#9851)
- create-react-admin can run in non-interactive mode (#9544)
- ra-data-fakerest accepts a delay parameter to simulate network delays (#9908)
- data-generator-retail now exposes types for the generated data (#9764)
Bump dependencies
- React-admin requires React 18 to leverage Concurrent React (#9827)
- React-admin uses the latest version of react-router, react-query, date-fns, fakerest, etc. (#9657, #9473, #9812, #9801, #9908)
- Internet Explorer is no longer supported (#9530)
Upgrading to v5
We've written a migration guide to help you upgrade your apps to v5. It covers all the breaking changes and how to adapt your code to the new APIs.
We estimate that a react-admin app with 50,000 lines of code will require about 2 days of work to upgrade to v5.
Changelog
For a detailed changelog, see the release notes for the following pre-releases: