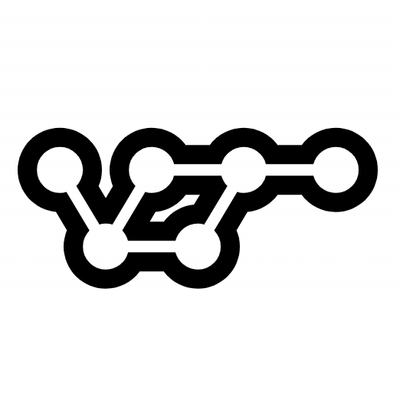
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
react-native-critizr
Advanced tools
React Native wrapper for Critizr
npm install react-native-critizr
This module does not require any extra step after rebuilding your app.
pod "Critizr-ios", :git => "https://github.com/critizr/critizr-ios-pod.git", :tag => "1.2.8"
pod install
<key>CritizrAPIKey</key>
<string>Critizr API Key</string>
<key>CritizrEnvironement</key>
<string>PreProduction</string>
or
<key>CritizrEnvironement</key>
<string>Production</string>
Importing the library:
import Critizr from 'react-native-critizr';
Initiliaze (Only works on Android):
Critizr.init({
apiKey: 'my-secret-api-key',
languageCode: 'en',
});
Manage language:
const language = await Critizr.getLanguage();
Critizr.setLanguage('fr');
Set user for current instance:
Critizr.setUser({
firstname: 'Michael',
lastname: 'Scott',
email: 'michael.scott@dundermifflin.com',
phone: '0123456789',
crmId: '123ABC',
});
Open feedback display:
// you don't have to give any parameter
Critizr.openFeedbackDisplay();
// show a targeted place
Critizr.openFeedbackDisplay({ placeId: 'your-place-id' });
//
// show a targeted place with different modes
//
Critizr.openFeedbackDisplay({
placeId: 'your-place-id',
mode: Critizr.FEEDBACK_MODES.START_WITH_FEEDBACK, // default
});
Critizr.openFeedbackDisplay({
placeId: 'your-place-id',
mode: Critizr.FEEDBACK_MODES.START_WITH_QUIZ,
});
Critizr.openFeedbackDisplay({
placeId: 'your-place-id',
mode: Critizr.FEEDBACK_MODES.ONLY_QUIZ,
});
Open store display:
Critizr.openStoreDisplay('your-place-id');
Get ratings using event listener:
useEffect(() => {
const listener = Critizr.addEventListener(
Critizr.EVENTS.RATING_RESULT,
(e) => {
if (e?.customerRelationship) {
setResult(
`Customer Relationship: ${e.customerRelationship}, Satisfaction: ${e.satisfaction}`
);
} else {
setResult('Event Rating Error!');
}
}
);
return () => listener.remove();
}, []);
You can perceive whether the user gives feedback (Only works on Android):
useEffect(() => {
const listener = Critizr.addEventListener(
Critizr.EVENTS.FEEDBACK_SENT,
(_) => {
console.log('User given a feedback!');
}
);
return () => listener.remove();
}, []);
See the contributing guide to learn how to contribute to the repository and the development workflow.
MIT
FAQs
React Native wrapper for Critizr
The npm package react-native-critizr receives a total of 1 weekly downloads. As such, react-native-critizr popularity was classified as not popular.
We found that react-native-critizr demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.