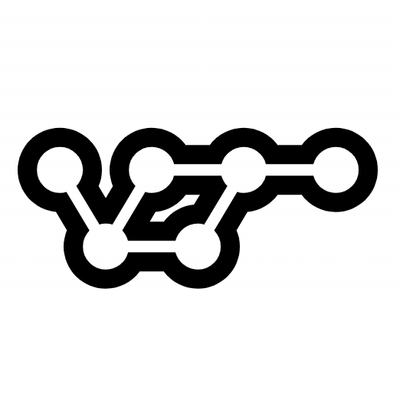
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
react-native-svg-transformer
Advanced tools
The react-native-svg-transformer package is a transformer for React Native that allows you to import and use SVG files as React components. This package simplifies the process of working with SVGs in a React Native project by transforming SVG files into components that can be easily styled and manipulated.
Importing SVG files
This feature allows you to import SVG files directly into your React Native components. The SVG file is transformed into a React component that can be used like any other component.
import Logo from './logo.svg';
const App = () => (
<View>
<Logo width={120} height={40} />
</View>
);
Styling SVG components
You can style the imported SVG components using standard React Native styles. This example demonstrates how to change the fill color of an SVG component.
import Logo from './logo.svg';
const App = () => (
<View>
<Logo width={120} height={40} style={{ fill: 'blue' }} />
</View>
);
Using SVG components with props
SVG components can accept props, allowing you to dynamically change their attributes. This example shows how to set the fill color of an SVG component using a prop.
import Logo from './logo.svg';
const App = () => (
<View>
<Logo width={120} height={40} fill='red' />
</View>
);
react-native-svg is a popular library for rendering SVG images in React Native. It provides a set of SVG elements that can be used to create complex graphics. Unlike react-native-svg-transformer, which focuses on transforming SVG files into components, react-native-svg provides a more comprehensive set of tools for working with SVGs directly in your code.
react-native-vector-icons is a library that provides a set of customizable icons for React Native. While it does not specifically handle SVG files, it offers a wide range of vector icons that can be used in place of SVGs. This package is useful if you need a variety of icons without the need to manage SVG files directly.
svgr is a tool that transforms SVGs into React components. It can be used in both web and React Native projects. While svgr is not specifically designed for React Native, it can be integrated into a React Native project to achieve similar functionality to react-native-svg-transformer.
Load SVG files in React Native.
Limitations: React Native's packager does not currently support running the transformer for .svg
file extension. That is why a .svgx
file extension should be used instead for your SVG files. This will hopefully be fixed in the future versions of React Native. Read more: facebook/metro/issues/176#issuecomment-393202582
Demo app: react-native-svg-example
Make sure that you have installed and linked react-native-svg
library:
https://github.com/react-native-community/react-native-svg#installation
yarn add --dev react-native-svg-transformer
Add this to your rn-cli.config.js
(make one if you don't have one already):
module.exports = {
getTransformModulePath() {
return require.resolve("react-native-svg-transformer");
},
getSourceExts() {
return ["js", "jsx", "svgx"];
}
};
...or if you are using Expo, in app.json
:
{
"expo": {
"packagerOpts": {
"sourceExts": ["js", "jsx", "svgx"],
"transformer": "node_modules/react-native-svg-transformer/index.js"
}
}
}
First make sure that you rename your .svg
file to .svgx
.
Import your .svgx
file inside a React component:
import Logo from "./logo.svgx";
You can then use your image as a component:
<Logo width={120} height={40} />
In addition to React Native, this transfomer depends on the following libraries:
v0.11.0
.svg
extension as React Native v0.57+ should support it.FAQs
SVG transformer for react-native
The npm package react-native-svg-transformer receives a total of 447,803 weekly downloads. As such, react-native-svg-transformer popularity was classified as popular.
We found that react-native-svg-transformer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.