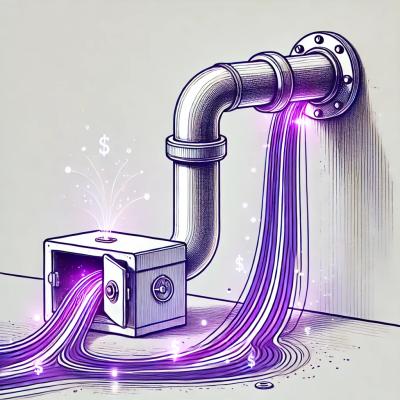
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
read-vinyl-file-stream
Advanced tools
Turns out that reading all the files in a vinyl stream is cumbersome, and supporting all of the options is a little bit annoying. I decided that I don't want to write that code more than once. So here is a library that does that. This is most useful for gulp plugins that need to transform all the files in a stream, though I am sure you can figure out other ways to use it too.
npm install read-vinyl-file-stream
The module is a function that creates a transform stream. It will read the vinyl file, whether it is a buffer or a stream internally. It takes the following parameters, in order:
'utf8'
- provide the content in a UTF-8 string.'buffer'
- provide the content in a raw buffer. This is useful if you are processing binary files, for example.The function that you provide to it has the following parameters, in order:
This is a function that will allow you to execute some code after all the files have been read but before the stream ends. It has the following parameters, in order:
Observe all of the files:
var readFiles = require('read-vinyl-file-stream');
var input = getVinylStream();
var hashOfFiles = {};
input.pipe(readFiles(function (content, file, stream, cb) {
hashOfFiles[file.path] = content;
cb();
}));
Transform the content of the file and output it back to the stream:
var readFiles = require('read-vinyl-file-stream');
var input = getVinylStream();
input.pipe(readFiles(function (content, file, stream, cb) {
var newContent = doWorkToTheContent(content);
cb(null, newContent);
}));
Split the file into multiple files and output all of them to the stream:
var readFiles = require('read-vinyl-file-stream');
var File = require('vinyl');
var input = getVinylStream();
input.pipe(readFiles(function (content, file, stream, cb) {
var lines = content.split('\n');
lines.forEach(function (line, idx) {
stream.push(new File({
contents: new Buffer(line),
path: file.path + 'line' + idx
}));
});
cb();
}));
Use inside gulp
(to create a filter):
var gulp = require('gulp');
var readFiles = require('read-vinyl-file-stream');
gulp.task('mytask', function() {
return gulp.src('*.ext')
.pipe(readFiles(function (content, file, stream, cb) {
if (/^n/.test(content)) {
return cb(null, content);
}
cb();
}))
.pipe(gulp.dest('filesThatStartWithN'));
});
FAQs
process a vinyl file stream with minimal code
The npm package read-vinyl-file-stream receives a total of 11,173 weekly downloads. As such, read-vinyl-file-stream popularity was classified as popular.
We found that read-vinyl-file-stream demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.