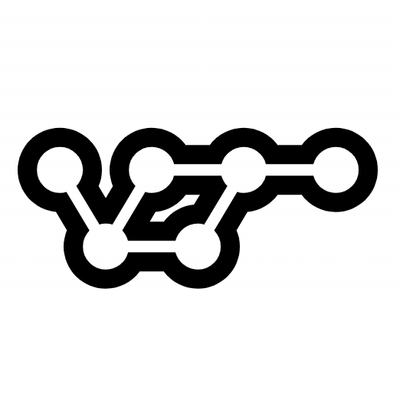
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
refire-client
Advanced tools
A lightweight TypeScript client for sending transactional emails through the Refire Email API.
Before using the client, you'll need an API key:
Keep your API key secure and never share it publicly or commit it to version control.
npm install refire-client
Initialize the Client
import { Refire } from "refire-client";
const refire = new Refire({
apiKey: "your-api-key",
});
You can optionally specify a custom API URL during initialization:
const refire = new Refire({
apiKey: "your-api-key",
apiUrl: "https://api.refire.email/v1",
});
To send a basic email without a template:
refire.sendEmail({
to: "recipient@example.com",
subject: "Hello",
name: "John Doe",
message: "Your email content here",
});
To send an email using a pre-defined template:
refire.sendEmailFromTemplate({
to: "recipient@example.com",
templateId: "<your_template_id>",
});
You can also use method chaining if preferred:
Sending a basic email:
new Refire("<your_api_key>").sendEmail({
to: "recipient@example.com",
subject: "Hello",
name: "John Doe",
message: "Your email content here",
});
Sending a template-based email:
new Refire("<your_api_key>").sendEmailFromTemplate({
to: "recipient@example.com",
templateId: "<your_template_id>",
});
Parameters for sending a basic email:
to
(string): Recipient email addresssubject
(string): Email subject linename
(string): Recipient namemessage
(string): Email contentParameters for sending a template-based email:
to
(string): Recipient email addresstemplateId
(string): ID of the template to useParameters for sending a basic test email:
to
(string): Recipient email addresssubject
(string): Email subject linename
(string): Recipient namemessage
(string): Email contentParameters for sending a template-based testemail:
to
(string): Recipient email addresstemplateId
(string): ID of the template to useThe client includes built-in error handling. Both sendEmail
and sendEmailFromTemplate
methods will throw an error if the API request fails:
try {
await refire.sendEmail({
to: "recipient@example.com",
subject: "Hello",
name: "John Doe",
message: "Your email content here",
});
} catch (error) {
console.error("Failed to send email:", error);
}
MIT
For more information and detailed documentation, visit refire.email.
FAQs
Refire Email API Client
The npm package refire-client receives a total of 2 weekly downloads. As such, refire-client popularity was classified as not popular.
We found that refire-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.