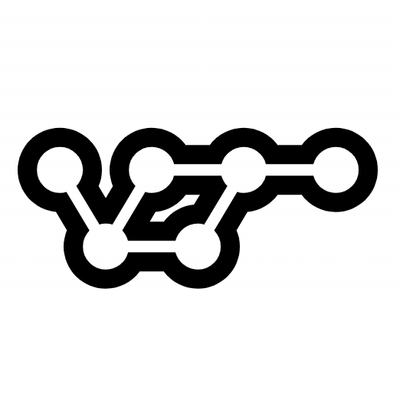
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
task-loader
Advanced tools
Class for loading assets
yarn add task-loader
npm i -S task-loader
import TaskLoader, {
LoadImageTask,
LoadVideoTask,
LoadHowlerAudioTask,
LoadJsonTask,
LoadScriptTask,
} from 'task-loader';
// Create the task loader instance
const taskLoader = new TaskLoader();
// Add events to the task
taskLoader.addEventListener(TaskLoaderEvent.START, () => console.log('Start'));
taskLoader.addEventListener(TaskLoaderEvent.UPDATE, ({ data }) => console.log('Update', data.progress));
taskLoader.addEventListener(TaskLoaderEvent.COMPLETE, () => console.log('Complete'));
taskLoader.addEventListener(TaskLoaderEvent.FAILURE, () => console.log('Failure during loading'));
// Load the tasks
taskLoader.loadTasks([
new LoadJsonTask({
// Array of strings or a single string with the path to the asset
assets: ['path/to/file.json'],
// The size of a batch, this is how many requests happen at the same time
batchSize: 1,
// The weight of the load task, the higher the number the more weight a task has on the
// total progress the lower the less. If none is provided all tasks have the same weight.
weight: 2,
// Flag to disable caching of assets, by default all
// assets are stored in an object for faster retrieval.
cached: true,
// When loading a lot of assets (for example an image sequence)
// you might want to group them so you can easily remove them when
// you no longer need them
cacheNameSpace: 'foo',
// Triggered when an asset is loaded, returns the original index + the asset
onAssetLoaded: ({index, asset}) => {}
}),
new LoadVideoTask({
assets: ['path/to/video.mp4'],
}),
new LoadHowlerAudioTask({
assets: ['path/to/audio.{format}}'],
// Define the formats of the file you've provided
formats: ['mp3', 'ogg'],
}),
new LoadImageTask({
assets: ['path/to/image-1.jpg', 'path/to/image-2.jpg'],
// Sometimes you might want change the image cross origin attribute, the default one is 'Use-Credentials'
crossOrigin: 'anonymous',
}),
new LoadScriptTask({
assets: ['path/to/file.js'],
}),
])
.then(() => {
console.log('All assets loaded')
});
.catch(() => {
console.log('Failure during loading')
});
import { LoadVideoTask } from 'task-loader';
// Create the task
const task = new LoadVideoTask({
assets: 'path/to/video-1.mp4',
});
// Add events to the task
task.addEventListener(TaskLoaderEvent.START, () => console.log('Start'));
task.addEventListener(TaskLoaderEvent.UPDATE, ({ data }) => console.log('Update', data.progress));
task.addEventListener(TaskLoaderEvent.COMPLETE, () => console.log('Complete'));
task.addEventListener(TaskLoaderEvent.FAILURE, () => console.log('Failure during loading'));
task.load()
.then(() => {
// Dispose of the load task when it's done
task.dispose();
console.log('All assets loaded');
});
.catch(() => {
console.log('Failure during loading');
});
import cacheManager from 'task-loader';
// Manually add an asset to the cacheManager
const asset = document.createElement('img');
cacheManager.add('image', asset, 'bar');
// Retrieve an asset from the cache manager once it's loaded
const videoBlob = cacheManager.get('path/to/video.jp4'));
// Retrieve images stored in a namespace
const images = cacheManager.get('path/to/image-1.jpg', 'foo');
// Remove assets from cache
cacheManager.remove('path/to/video.mp4');
// Remove assets within a namespace
cacheManager.remove('foo');
Keep in mind when tree shaking a module it will include all other dependencies for all tasks in your project (this means including Howler). If you do not want this, please include theme separately:
import TaskLoader from 'task-loader/lib/TaskLoader';
import cacheManager from 'task-loader/lib/CacheManager';
import LoadImageTask from 'task-loader/lib/task/LoadImageTask';
import LoadVideoTask from 'task-loader/lib/task/LoadVideoTask';
import LoadHowlerAudioTask from 'task-loader/lib/task/LoadHowlerAudioTask';
import LoadJsonTask from 'task-loader/lib/task/LoadJsonTask';
import LoadScriptTask from 'task-loader/lib/task/LoadScriptTask';
I've included an example setup where you can see the loader in action, to run the project follow these steps:
git clone https://github.com/larsvanbraam/task-loader.git
cd task-loader/example
yarn
yarn dev
localhost:8080
or click this link to preview online
View the generated documentation.
In order to build task-loader, ensure that you have Git and Node.js installed.
Clone a copy of the repo:
git clone https://github.com/larsvanbraam/task-loader.git
Change to the task-loader directory:
cd task-loader
Install dev dependencies:
yarn
Use one of the following main scripts:
yarn build # build this project
yarn dev # run compilers in watch mode, both for babel and typescript
yarn test # run the unit tests incl coverage
yarn test:dev # run the unit tests in watch mode
yarn lint # run eslint and tslint on this project
yarn doc # generate typedoc documentation
When installing this module, it adds a pre-commit hook, that runs lint and prettier commands before committing, so you can be sure that everything checks out.
View CONTRIBUTING.md
View CHANGELOG.md
View AUTHORS.md
MIT © Lars van Braam
FAQs
Load assets like a boss
We found that task-loader demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.