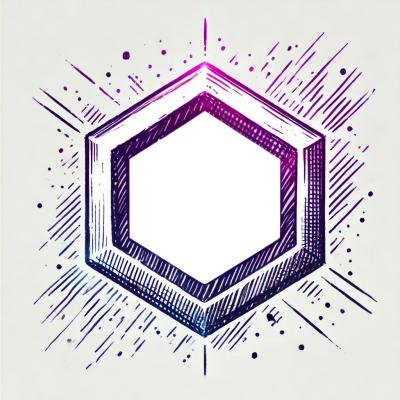
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
transformation-matrix
Advanced tools
2d transformation matrix functions written in ES6 syntax. Tree shaking ready!
The 'transformation-matrix' npm package provides a set of utilities for working with 2D affine transformations. It allows you to create, manipulate, and apply transformation matrices for operations such as translation, rotation, scaling, and skewing.
Translation
This feature allows you to create a translation matrix that moves points by a specified distance along the x and y axes.
const { translate } = require('transformation-matrix');
const translationMatrix = translate(10, 20);
console.log(translationMatrix);
Rotation
This feature allows you to create a rotation matrix that rotates points by a specified angle in radians.
const { rotate } = require('transformation-matrix');
const rotationMatrix = rotate(Math.PI / 4);
console.log(rotationMatrix);
Scaling
This feature allows you to create a scaling matrix that scales points by specified factors along the x and y axes.
const { scale } = require('transformation-matrix');
const scalingMatrix = scale(2, 3);
console.log(scalingMatrix);
Skewing
This feature allows you to create a skewing matrix that skews points by specified angles along the x and y axes.
const { skew } = require('transformation-matrix');
const skewingMatrix = skew(0.5, 0.3);
console.log(skewingMatrix);
Combining Transformations
This feature allows you to combine multiple transformation matrices into a single matrix.
const { compose, translate, rotate, scale } = require('transformation-matrix');
const combinedMatrix = compose(translate(10, 20), rotate(Math.PI / 4), scale(2, 3));
console.log(combinedMatrix);
The 'gl-matrix' package is a high-performance matrix and vector library for WebGL. It provides similar functionalities for 2D and 3D transformations, but is more focused on performance and is widely used in graphics programming.
The 'transformation-matrix-js' package offers similar functionalities for 2D transformations, including translation, rotation, scaling, and skewing. It is a lightweight alternative with a focus on simplicity.
The 'matrix-js' package provides general-purpose matrix operations, including those for 2D transformations. It is more versatile but less specialized compared to 'transformation-matrix'.
Javascript isomorphic 2D affine transformations written in ES6 syntax. Manipulate transformation matrices with this totally tested library!
Transformations, i.e. linear invertible automorphisms, are used to map a picture into another one with different size, position and orientation. Given a basis, transformations are represented by means of squared invertible matrices, called transformation matrices. A geometric transformation is defined as a one-to-one mapping of a point space to itself, which preservers some geometric relations of figures. - Geometric Programming for Computer Aided Design
This library allows us to:
import {scale, rotate, translate, compose, applyToPoint} from 'transformation-matrix';
let {scale, rotate, translate, compose, applyToPoint} = window.TransformationMatrix;
let {scale, rotate, translate, compose, applyToPoint} = require('transformation-matrix')
let matrix = compose(
translate(40,40),
rotate(Math.PI/2),
scale(2, 4)
);
applyToPoint(matrix, {x: 42, y: 42});
// { x: -128, y: 124.00000000000001 }
applyToPoint(matrix, [16, 24]);
// [ -56, 72 ]
npm install transformation-matrix
# or
yarn add transformation-matrix
<script src="https://unpkg.com/transformation-matrix@1"></script>
A Transformation Matrix is defined as an Object
with 6 keys: a
, b
, c
, d
, e
and f
.
const matrix = { a: 1, c: 0, e: 0,
b: 0, d: 1, f: 0 }
A Point can be defined in two different ways:
Object
, with inside two keys: x
and y
const point = { x: 24, y: 42 }
Array
, with two items in the form [x, y]
const point = [ 24, 42 ]
available at http://chrvadala.github.io/transformation-matrix/
Object
| Array
Calculate a point transformed with an affine matrix
array
Calculate an array of points transformed with an affine matrix
Object
Extract an affine matrix from an object that contains a,b,c,d,e,f keys Each value could be a float or a string that contains a float
Object
Parse a string matrix formatted as matrix(a,b,c,d,e,f)
Object
Parser for SVG Trasform Attribute http://www.w3.org/TR/SVG/coords.html#TransformAttribute
Warning: This should be considered BETA until it is released a stable version of pegjs.
Object
Returns a matrix that transforms a triangle t1 into another triangle t2, or throws an exception if it is impossible.
Object
Identity matrix
Object
Calculate a matrix that is the inverse of the provided matrix
boolean
Check if the object contain an affine matrix
Object
Calculate a rotation matrix
Object
Calculate a rotation matrix with a DEG angle
Object
Calculate a scaling matrix
Object
Calculate a shear matrix
Object
Calculate a skew matrix
Object
Calculate a skew matrix using DEG angles
Object
Rounds all elements of the given matrix using the given precision
string
Serialize the matrix to a string that can be used with CSS or SVG
string
Serialize the matrix to a string that can be used with CSS or SVG
string
Serialize the matrix to a string that can be used with CSS or SVG
Object
Merge multiple matrices into one
Object
Merge multiple matrices into one (alias of transform
)
Object
Calculate a translate matrix
translate(tx)
, scale(sx)
, rotate(angle, cx, cy)
fromTransformAttribute
, Discontinues node 4 supportskew(ax, ay)
, Upgrades deps, Improves fromTransformAttribute
compose
function, Upgrades deps, Exposes skew operation #37Array
in the form [x, y]
#38fromTriangle
and smoothMatrix
functions #41Object
| Array
Calculate a point transformed with an affine matrix
Kind: global function
Returns: Object
| Array
- Point
Param | Description |
---|---|
matrix | Affine matrix |
point | Point |
array
Calculate an array of points transformed with an affine matrix
Kind: global function
Returns: array
- Array of points
Param | Description |
---|---|
matrix | Affine matrix |
points | Array of points |
Object
Extract an affine matrix from an object that contains a,b,c,d,e,f keys Each value could be a float or a string that contains a float
Kind: global function
Returns: Object
- }
Param |
---|
object |
Object
Parse a string matrix formatted as matrix(a,b,c,d,e,f)
Kind: global function
Returns: Object
- Affine matrix
Param | Description |
---|---|
string | String with a matrix |
Object
Parser for SVG Trasform Attribute http://www.w3.org/TR/SVG/coords.html#TransformAttribute
Warning: This should be considered BETA until it is released a stable version of pegjs.
Kind: global function
Returns: Object
- Parsed matrices
Param | Description |
---|---|
transformString | string |
Object
Returns a matrix that transforms a triangle t1 into another triangle t2, or throws an exception if it is impossible.
Kind: global function
Returns: Object
- Affine matrix which transforms t1 to t2
Throws:
Param | Type | Description |
---|---|---|
t1 | Array.<{x: number, y: number}> | Array.<Array.<number>> | an array of points containing the three points for the first triangle |
t2 | Array.<{x: number, y: number}> | Array.<Array.<number>> | an array of points containing the three points for the second triangle |
Object
Identity matrix
Kind: global function
Returns: Object
- Affine matrix
Object
Calculate a matrix that is the inverse of the provided matrix
Kind: global function
Returns: Object
- Affine matrix
Param | Description |
---|---|
matrix | Affine matrix |
boolean
Check if the object contain an affine matrix
Kind: global function
Param |
---|
object |
Object
Calculate a rotation matrix
Kind: global function
Returns: Object
- Affine matrix *
Param | Description |
---|---|
angle | Angle in radians |
[cx] | If (cx,cy) are supplied the rotate is about this point |
[cy] | If (cx,cy) are supplied the rotate is about this point |
Object
Calculate a rotation matrix with a DEG angle
Kind: global function
Returns: Object
- Affine matrix
Param | Description |
---|---|
angle | Angle in degree |
[cx] | If (cx,cy) are supplied the rotate is about this point |
[cy] | If (cx,cy) are supplied the rotate is about this point |
Object
Calculate a scaling matrix
Kind: global function
Returns: Object
- Affine matrix
Param | Default | Description |
---|---|---|
sx | Scaling on axis x | |
[sy] | sx | Scaling on axis y (default sx) |
Object
Calculate a shear matrix
Kind: global function
Returns: Object
- Affine matrix
Param | Description |
---|---|
shx | Shear on axis x |
shy | Shear on axis y |
Object
Calculate a skew matrix
Kind: global function
Returns: Object
- Affine matrix
Param | Description |
---|---|
ax | Skew on axis x |
ay | Skew on axis y |
Object
Calculate a skew matrix using DEG angles
Kind: global function
Returns: Object
- Affine matrix
Param | Description |
---|---|
ax | Skew on axis x |
ay | Skew on axis y |
Object
Rounds all elements of the given matrix using the given precision
Kind: global function
Returns: Object
- the rounded matrix
Param | Type | Description |
---|---|---|
m | Object | a matrix to round |
[precision] | a precision to use for Math.round. Defaults to 10000000000 (meaning which rounds to the 10th digit after the comma). |
string
Serialize the matrix to a string that can be used with CSS or SVG
Kind: global function
Returns: string
- String that contains a matrix formatted as matrix(a,b,c,d,e,f)
Param | Description |
---|---|
matrix | Affine matrix |
string
Serialize the matrix to a string that can be used with CSS or SVG
Kind: global function
Returns: string
- String that contains a matrix formatted as matrix(a,b,c,d,e,f)
Param | Description |
---|---|
matrix | Affine matrix |
string
Serialize the matrix to a string that can be used with CSS or SVG
Kind: global function
Returns: string
- String that contains a matrix formatted as matrix(a,b,c,d,e,f)
Param | Description |
---|---|
matrix | Affine matrix |
Object
Merge multiple matrices into one
Kind: global function
Returns: Object
- Affine matrix
Param | Type | Description |
---|---|---|
...matrices | object | list of matrices |
Object
Merge multiple matrices into one (alias of transform
)
Kind: global function
Returns: Object
- Affine matrix
Param | Type | Description |
---|---|---|
...matrices | object | list of matrices |
Object
Calculate a translate matrix
Kind: global function
Returns: Object
- Affine matrix
Param | Default | Description |
---|---|---|
tx | Translation on axis x | |
[ty] | 0 | Translation on axis y |
FAQs
2d transformation matrix functions written in ES6 syntax. Tree shaking ready!
The npm package transformation-matrix receives a total of 32,366 weekly downloads. As such, transformation-matrix popularity was classified as popular.
We found that transformation-matrix demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.