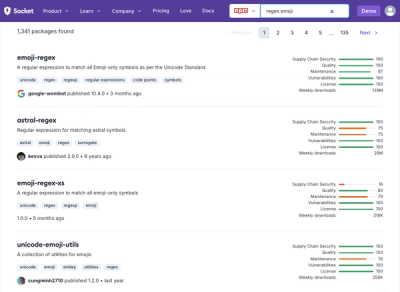
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
The ts-mixer package is a TypeScript library that provides utilities for creating mixins and multiple inheritance in TypeScript. It allows developers to combine multiple classes into one, enabling more flexible and reusable code structures.
Basic Mixin
This feature allows you to create a new class that combines the methods and properties of multiple classes. In this example, class C inherits methods from both class A and class B.
const { Mixin } = require('ts-mixer');
class A {
methodA() {
console.log('Method A');
}
}
class B {
methodB() {
console.log('Method B');
}
}
class C extends Mixin(A, B) {}
const c = new C();
c.methodA(); // Method A
c.methodB(); // Method B
Advanced Mixin with Constructor
This feature demonstrates how to handle constructors when mixing classes. The new class C can initialize properties from both class A and class B.
const { Mixin } = require('ts-mixer');
class A {
constructor(public name: string) {}
methodA() {
console.log(`Method A: ${this.name}`);
}
}
class B {
constructor(public age: number) {}
methodB() {
console.log(`Method B: ${this.age}`);
}
}
class C extends Mixin(A, B) {
constructor(name: string, age: number) {
super(name, age);
}
}
const c = new C('John', 30);
c.methodA(); // Method A: John
c.methodB(); // Method B: 30
Mixin with Interfaces
This feature shows how to use mixins with interfaces. Class C implements both IA and IB interfaces and inherits methods from class A and class B.
const { Mixin } = require('ts-mixer');
interface IA {
methodA(): void;
}
interface IB {
methodB(): void;
}
class A implements IA {
methodA() {
console.log('Method A');
}
}
class B implements IB {
methodB() {
console.log('Method B');
}
}
class C extends Mixin(A, B) implements IA, IB {}
const c = new C();
c.methodA(); // Method A
c.methodB(); // Method B
The mixwith package provides a similar functionality for creating mixins in JavaScript and TypeScript. It offers a more flexible and modern approach to mixins, but ts-mixer is more TypeScript-centric and provides better type safety.
The mixin-deep package allows deep merging of objects and mixins. While it is more focused on object merging rather than class-based mixins, it can be used to achieve similar results in a different way.
Lodash is a utility library that provides a wide range of functions, including mixin capabilities. However, it is more general-purpose and not specifically designed for TypeScript or class-based mixins.
It seems that no one has been able to provide an acceptable way to gracefully implement the mixin pattern with TypeScript. Mixins as described by the TypeScript docs are far less than ideal. Countless online threads feature half-working snippets. Some are elegant, but fail to work properly with static properties. Others solve static properties, but they don't work well with generics. Some are memory-optimized, but force you to write the mixins in an awkward, cumbersome format.
My fruitless search has led me to believe that there is no perfect solution with the current state of TypeScript. Instead, I present a "tolerable" solution that attempts to take the best from the many different implementations while mitigating their flaws as much as possible.
Mixin<A & B>(A, B)
in
order for the types to work correctly. ts-mixer is able to infer these types, so you can
just do Mixin(A, B)
... except when generics are involved. See
Dealing with Generics.Mixin
function is used as the model for the signature.instanceof
support; Because this library is intended for use with TypeScript, running
an instanceof
check is generally not needed. Additionally, adding support can have
negative effects on performance. See the
MDN documentation
for more information.npm i --save ts-mixer
class Person {
protected name: string;
constructor(name: string) {
this.name = name;
}
}
class RunnerMixin {
protected runSpeed: number = 10;
public run(){
return 'They are running at ' + this.runSpeed + ' ft/sec';
}
}
class JumperMixin {
protected jumpHeight: number = 3;
public jump(){
return 'They are jumping ' + this.jumpHeight + ' ft in the air';
}
}
class LongJumper extends Mixin(Person, RunnerMixin, JumperMixin) {
protected stateDistance() {
return 'They landed ' + this.runSpeed * this.jumpHeight + ' ft from the start!';
}
public longJump() {
let msg = "";
msg += this.run() + '\n';
msg += this.jump() + '\n';
msg += this.stateDistance() + '\n';
return msg;
}
}
Consider the following scenario:
class Person {
public static TOTAL: number = 0;
constructor() {
(<typeof Person>this.constructor).TOTAL ++;
}
}
class StudentMixin {
public study() { console.log('I am studying so hard') }
}
class CollegeStudent extends Mixin(Person, StudentMixin) {}
It would be expected that class CollegeStudent
should have the property TOTAL
since
CollegeStudent
inherits from Person
. The Mixin
function properly sets up the
inheritance of this static property, so that modifying it on the CollegeStudent
class
will also affect the Person
class:
let p1 = new Person();
let cs1 = new CollegeStudent();
// Person.TOTAL === CollegeStudent.TOTAL === 2
The only issue is that due to the impossibility of specifying properties on a constructor type, you must use some type assertions to keep the TypeScript compiler from complaining:
CollegeStudent.TOTAL ++; // error
(<any>CollegeStudent).TOTAL ++; // ok
(<typeof Person><unknown>CollegeStudent).TOTAL++; // ugly, but better
Normally, the Mixin
function is able to figure out the class types and produce an
appropriately typed result. However, when generics are involved, you should pass in
type parameters to the Mixin
function like so:
class GenClassA<T> {}
class GenClassB<T> {}
class Mixed<T1, T2> extends Mixin<GenClassA<T1>, GenClassB<T2>>(GenClassA, GenClassB) {}
While this is a bit of an inconvenience, it only affects generic classes.
All contributions are welcome, just please run npm run lint
and npm run test
before
submitting an MR. If you add a new feature, please make sure it's covered by a test case.
Tanner Nielsen tannerntannern@gmail.com
FAQs
A very small TypeScript library that provides tolerable Mixin functionality.
The npm package ts-mixer receives a total of 476,569 weekly downloads. As such, ts-mixer popularity was classified as popular.
We found that ts-mixer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.