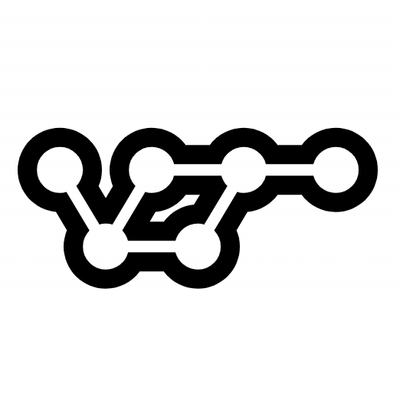
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
unipayconnect
Advanced tools
<!-- **unipayconnect/unipayconnect** is a ✨ _special_ ✨ repository because its `README.md` (this file) appears on your GitHub profile.
UnipayConnect is a unified payment library designed to make handling payments with Stripe, PayPal, and Razorpay simple and efficient. It abstracts away the complexities of using multiple payment APIs and offers a straightforward interface for initializing payments, capturing payments, and verifying webhook events.
Before getting started, ensure you have the following:
You can install the UnipayConnect package using npm:
npm install unipayconnect
Create a .env file and add your API keys and connection details:
STRIPE_SECRET_KEY=<your-stripe-secret-key>
STRIPE_WEBHOOK_SECRET=<your-stripe-webhook-secret>
PAYPAL_CLIENT_ID=<your-paypal-client-id>
PAYPAL_CLIENT_SECRET=<your-paypal-secret>
PAYPAL_WEBHOOK_ID=<your-paypal-webhook-id>
NODE_ENV='sandbox'||'production' for paypal
RAZORPAY_KEY_ID=<your-razorpay-key-id>
RAZORPAY_KEY_SECRET=<your-razorpay-secret>
RAZORPAY_WEBHOOK_SECRET=<your-razorpay-webhook-secret>
PORT=<your-port>
JWT_SECRET='<your-jwt-secret>'
REACT_APP_API_URL=http://localhost
REACT_APP_RAZORPAY_KEY_ID=<your-razorpay-key-id>
REACT_APP_RAZORPAY_KEY_SECRET=<your-razorpay-key-secret>
REACT_APP_STRIPE_SECRET_KEY=<your-stripe-webhook-secret>
REACT_APP_PAYPAL_CLIENT_ID=<your-paypal-client-id>
REACT_APP_PAYPAL_CLIENT_SECRET=<your-paypal-secret>
REACT_APP_RAZORPAY_URL='https://checkout.razorpay.com/v1/checkout.js'
const unipayconnect = require("unipayconnect");
// Import the payment providers
const StripeProvider = require("@unipayconnect/core/gateways/stripe");
const PayPalProvider = require("@unipayconnect/core/gateways/paypal");
const RazorPayProvider = require("@unipayconnect/core/gateways/razorpay");
// Register the providers with your credentials
const stripe = unipayconnect.register(
"stripe",
new StripeProvider(process.env.REACT_APP_STRIPE_SECRET_KEY)
);
const paypal = unipayconnect.register(
"paypal",
new PayPalProvider(process.env.REACT_APP_PAYPAL_CLIENT_SECRET)
);
const razorpay = unipayconnect.register(
"razorpay",
new RazorPayProvider({
key_id: process.env.REACT_APP_RAZORPAY_KEY_ID,
key_secret: process.env.REACT_APP_RAZORPAY_KEY_SECRET,
})
);
const session = await unipayconnect.createCheckoutSession({
price: 250, //total value of the cart
currency: "USD",
providers: ["razorpay", "stripe", "paypal"],
//Not Mandatory
name: "Avanish",
email: "avanishporwal01@gmail.com",
products: [
{
name: "Nike Tshirt",
price: 50,
quantity: 1,
},
{
name: "Puma shoes",
price: 100,
quantity: 2,
},
],
});
-Capture Payment After a successful checkout, capture the payment:
const paymentResult = await unipayconnect.capturePayment({
providerName: "razorpay", // Choose provider
paymentId: "pay_ABC123", // Payment ID from the provider
amount: 100, // Only required for Razorpay
});
providerName: Payment provider (required).
paymentId: The ID of the payment (from Stripe/PayPal/Razorpay).
amount: Total payment amount (required for Razorpay).
Verify Webhook Events Webhooks are crucial for receiving updates from payment providers. Here's how you verify the webhook data:
const isValid = unipayconnect.verifyWebhookPayload(
req.body, // Webhook payload received from the provider
req.headers["provider-name"] // Provider name ('stripe', 'paypal', 'razorpay')
// Signature from webhook headers
req.headers['paypal-transmission-sig']
|| req.headers['stripe-signature']
|| req.headers['X-Razorpay-Signature']
);
Available Methods Each provider has its own methods for interacting with their API. Here's a quick overview of the common methods available:
token: Here, refers to token recieved after creating checkout session.
Stripe: initPayment(token),getProvider(providerName), getAllProviders(), register(providerName,providerInstance), etc.
PayPal: initPayment(token),getProvider(providerName), getAllProviders(), register(providerName,providerInstance), etc.
Razorpay: initPayment(token),getProvider(providerName), getAllProviders(), register(providerName,providerInstance), etc.
Additional Configuration You can configure additional options such as successUrl, cancelUrl, and more depending on the gateway you're using. I motivate you to play with each provider for more detailed options.
app.post("/api/v1/payments/create-checkout-session", async (req, res) => {
const { price, currency, providers, products } = req.body;
const sessionData = await unipayconnect.createCheckoutSession({
providerName: providers[0],
price,
currency,
products,
});
res.status(200).json(sessionData);
});
app.post("/api/v1/payments/capture", async (req, res) => {
const { providerName, paymentId, amount } = req.body;
const result = await unipayconnect.capturePayment({
providerName,
paymentId,
amount,
});
res.status(200).json(result);
});
app.post("/api/v1/payments//verify-webhook", (req, res) => {
const providerName = req.headers["provider-name"];
const signature =
req.headers["stripe-signature"] ||
req.headers["paypal-transmission-sig"] ||
req.headers["X-Razorpay-Signature"];
const verified = unipayconnect.verifyWebhookPayload(
providerName,
req.body,
signature
);
res.status(verified ? 200 : 400).json({ verified });
});
Using ngrok (Recommended)
npm install -g ngrok
ngrok http 8080
Copy the Forwarding URL provided by ngrok (e.g., https://your-ngrok-subdomain.ngrok-free.app).
Once you have the publicly accessible URL, add it to your payment provider's webhook settings.
You're all set! Your local environment is now ready to receive webhooks.
To install and set up the unipayconnect/packages/client
on your local machine, follow these steps:
Node.js: Ensure you have Node.js installed on your machine. You can download it from nodejs.org.
npm: npm is included with Node.js. You can check if it's installed by running the following command in your terminal:
npm -v
First, clone the unipayconnect repository from GitHub:
git clone https://github.com/yourusername/unipayconnect.git
Change your working directory to the client package:
cd unipayconnect/packages/client
Install the required dependencies using npm:
npm install
Create a .env file in the packages/client directory to store your API keys and any other environment variables required for your application:
touch .env
Add your configuration details:
REACT_APP_API_URL=http://localhost
REACT_APP_RAZORPAY_KEY_ID=<your-razorpay-key-id>
REACT_APP_RAZORPAY_URL='https://checkout.razorpay.com/v1/checkout.js'
REACT_APP_RAZORPAY_KEY_SECRET=<your-razorpay-key-secret>
REACT_APP_STRIPE_SECRET_KEY=<your-stripe-webhook-secret>
REACT_APP_PAYPAL_CLIENT_ID=<your-paypal-client-id>
REACT_APP_PAYPAL_CLIENT_SECRET=<your-paypal-secret>
Start the development server to see your client application in action:
npm start
The application should open in your default browser at http://localhost:3000.
For production, you might want to build the application by running:
npm run build
UnipayConnect lets you build a custom checkout page like Stripe's. Here’s an example of a form using React Hook Form and Tailwind CSS:
import { useForm } from "react-hook-form";
const CheckoutForm = ({ products, totalAmount }) => {
const { register, handleSubmit } = useForm();
const onSubmit = async (data) => {
console.log(data);
// Add your submit logic here
};
return (
<form onSubmit={handleSubmit(onSubmit)} className="checkout-form">
<div className="col-left">
<input {...register("name")} placeholder="Name" className="input" />
<input {...register("email")} placeholder="Email" className="input" />
<input {...register("amount")} placeholder="Amount" className="input" />
{/* Add more fields as necessary */}
</div>
<div className="col-right">
<h2>Order Summary</h2>
{products.map((product, index) => (
<div key={index}>
{product.name} - ${product.price * product.quantity}
</div>
))}
<div>Total: ${totalAmount}</div>
</div>
<button type="submit" className="submit-button">
Pay Now
</button>
</form>
);
};
<div>
<label>
<input {...register("provider")} type="radio" value="stripe" /> Stripe
</label>
<label>
<input {...register("provider")} type="radio" value="paypal" /> PayPal
</label>
<label>
<input {...register("provider")} type="radio" value="razorpay" /> Razorpay
</label>
</div>
Use our Postman collection to test API endpoints: Postman
UnipayConnect simplifies payments across multiple providers. Whether it's creating checkout sessions, capturing payments, or verifying webhooks, it provides an easy way to integrate and manage multiple payment gateways without getting into their complex APIs.
FAQs
<!-- **unipayconnect/unipayconnect** is a ✨ _special_ ✨ repository because its `README.md` (this file) appears on your GitHub profile.
The npm package unipayconnect receives a total of 59 weekly downloads. As such, unipayconnect popularity was classified as not popular.
We found that unipayconnect demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.