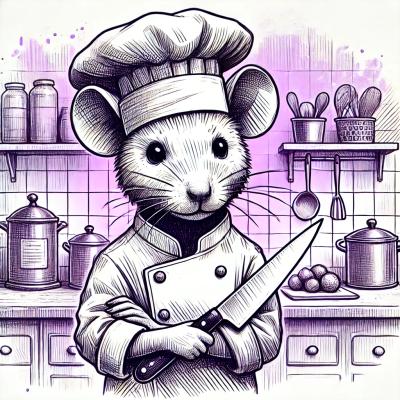
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
vega-parser
Advanced tools
The vega-parser npm package is a library for parsing Vega specifications into a format that can be used to generate visualizations. It is part of the Vega ecosystem, which is a set of tools for creating, sharing, and exploring data visualizations. The parser takes a JSON specification and converts it into a dataflow graph that can be rendered by Vega's runtime.
Parsing Vega Specifications
This feature allows you to parse a Vega specification JSON into a dataflow graph. The parsed specification can then be used to generate visualizations.
const vega = require('vega-parser');
const spec = { /* Vega specification JSON */ };
const parsedSpec = vega.parse(spec);
console.log(parsedSpec);
Customizing Parsing Options
This feature allows you to customize the parsing process by providing additional options. These options can control various aspects of the parsing, such as logging and error handling.
const vega = require('vega-parser');
const spec = { /* Vega specification JSON */ };
const options = { /* Custom parsing options */ };
const parsedSpec = vega.parse(spec, options);
console.log(parsedSpec);
Vega-Lite is a high-level grammar of interactive graphics. It provides a concise JSON syntax for rapidly generating visualizations to support analysis. Compared to vega-parser, Vega-Lite is more user-friendly and easier to use for creating standard visualizations, but it is less flexible for complex custom visualizations.
D3.js is a JavaScript library for producing dynamic, interactive data visualizations in web browsers. It uses HTML, SVG, and CSS. While D3 offers more control and flexibility compared to vega-parser, it requires more effort and expertise to create visualizations from scratch.
Plotly.js is a high-level, declarative charting library. It supports a wide range of chart types and is built on top of D3 and stack.gl. Compared to vega-parser, Plotly.js is more focused on providing ready-to-use chart types and is easier to use for quick visualizations, but it may not offer the same level of customization.
Parse Vega specifications to runtime dataflow descriptions.
# vega.parse(specification[, config]) <>
Parses a Vega JSON specification as input and produces a reactive dataflow graph description for a visualization. The output description uses the format of the vega-runtime module. To create a visualization, use the runtime dataflow description as the input to a Vega View instance.
The optional config object provides visual encoding defaults for marks, scales, axes and legends. Different configuration settings can be used to change choices of layout, color, type faces, font sizes and more to realize different chart themes. For more, see the configuration documentation below or view the source code defining Vega's default configuration.
In addition to passing configuration options to this parse method, Vega JSON specifications may also include a top-level "config"
block specifying configuration properties. Configuration options defined within a Vega JSON file take precedence over those provided to the parse method.
The Vega parser accepts a configuration file that defines default settings for a variety of visual encoding choices. Different configuration files can be used to "theme" charts with a customized look and feel. A configuration file is simply a JavaScript object with a set of named properties, grouped by type.
For more information regarding the supported configuration options, please see the Vega config documentation.
FAQs
Parse Vega specifications to runtime dataflows.
The npm package vega-parser receives a total of 55,139 weekly downloads. As such, vega-parser popularity was classified as popular.
We found that vega-parser demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.