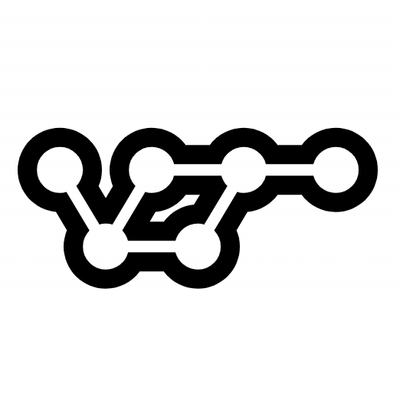
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
vue3-summernote-editor
Advanced tools
A VueJs 3 component for use Summernote WYSIWYG
// npm install
npm install vue3-summernote-editor --save
You must be have jQuery
at window.$
and install summernote by yourself.
// import SummernoteEditor
import SummernoteEditor from 'vue3-summernote-editor';
const vueApp = createApp({});
vueApp.component('SummernoteEditor', SummernoteEditor);
// import SummernoteEditor
import SummernoteEditor from 'vue3-summernote-editor';
<template>
<SummernoteEditor
v-model="myValue"
@update:modelValue="summernoteChange($event)"
@summernoteImageLinkInsert="summernoteImageLinkInsert"
/>
</template>
<script>
export default {
// declare SummernoteEditor
components: {SummernoteEditor},
data() {
return {
myValue: '',
}
},
methods: {
summernoteChange(newValue) {
console.log("summernoteChange", newValue);
},
summernoteImageLinkInsert(...args) {
console.log("summernoteImageLinkInsert()", args);
},
}
}
</script>
options
: option[]
window.SUMMERNOTE_DEFAULT_CONFIGS
update:modelValue
summernote bare events
"summernote.change": "summernoteChange"
"summernote.image.link.insert": "summernoteImageLinkInsert"
FAQs
A vuejs 3 component for Summernote library
We found that vue3-summernote-editor demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.