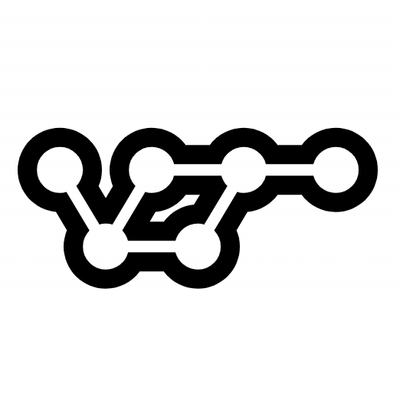
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Integrate Composio with llamaindex agents to allow them to interact seamlessly with external apps & data sources, enhancing their functionality and reach.
Ensure you have the necessary packages installed and connect your GitHub account to allow your agents to utilize GitHub functionalities.
# Install Composio llamaindex package
pip install composio-llamaindex
# Connect your GitHub account
composio-cli add github
# View available applications you can connect with
composio-cli show-apps
Prepare your environment by initializing necessary imports from llamaindex and setting up your agent.
from llama_index.llms.openai import OpenAI
from llama_index.core.llms import ChatMessage
from llama_index.core.agent import FunctionCallingAgentWorker
import dotenv
from llama_index.core.tools import FunctionTool
# Load environment variables from .env
dotenv.load_dotenv()
llm = OpenAI(model="gpt-4-turbo")
Access GitHub tools provided by Composio for llamaindex.
from composio_llamaindex import App, Action, ComposioToolSet
# Get All the tools
composio_toolset = ComposioToolSet()
tools = composio_toolset.get_actions(
actions=[Action.GITHUB_STAR_A_REPOSITORY_FOR_THE_AUTHENTICATED_USER]
)
print(tools)
Configure the agent to perform tasks such as starring a repository on GitHub.
prefix_messages = [
ChatMessage(
role="system",
content=(
"You are now a integration agent, and what ever you are requested, you will try to execute utilizing your toools."
),
)
]
agent = FunctionCallingAgentWorker(
tools=tools,
llm=llm,
prefix_messages=prefix_messages,
max_function_calls=10,
allow_parallel_tool_calls=False,
verbose=True,
).as_agent()
Validate the execution and response from the agent to ensure the task was completed successfully.
response = agent.chat("Hello! I would like to star a repo composiohq/composio on GitHub")
print("Response:", response)
FAQs
Use Composio to get an array of tools with your LlamaIndex agent.
We found that composio-llamaindex demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.