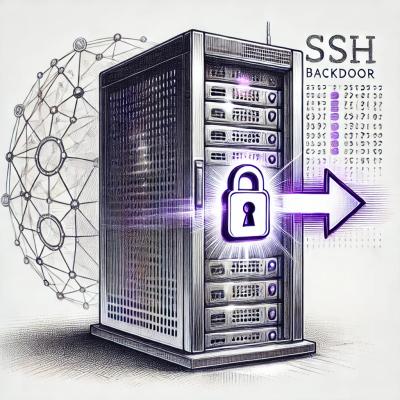
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
A module for cryptography built from scratch, offering a robust suite of classes and functions for both symmetric and asymmetric encryption, as well as hashing functionalities
The cryptosystems
package offers a robust suite of classes and functions for both symmetric and asymmetric encryption, as well as hashing functionalities. Designed for seamless encryption, decryption, and cryptographic operations, this package is lightweight and efficient, relying solely on Python’s built-in libraries: os
and hashlib
. With all cryptographic logic implemented from scratch, cryptosystems provides a streamlined, dependency-free solution, ensuring consistency and reliability across different environments as well as Python versions.
To install the package, simply clone the repository and install the dependencies:
git clone https://github.com/ishan-surana/cryptosystems.git
cd cryptosystems
pip install -r requirements.txt
from cryptosystems import AdditiveCipher
cipher = AdditiveCipher(3)
ciphertext = cipher.encrypt("Hello World")
print(ciphertext) # Output: 'Khoor Zruog'
plaintext = cipher.decrypt(ciphertext)
print(plaintext) # Output: 'Hello World'
from cryptosystems import MultiplicativeCipher
cipher = MultiplicativeCipher(5)
ciphertext = cipher.encrypt("Hello World")
print(ciphertext) # Output: 'Czggj Rjmgy'
plaintext = cipher.decrypt(ciphertext)
print(plaintext) # Output: 'Hello World'
from cryptosystems import AffineCipher
cipher = AffineCipher(5, 8)
ciphertext = cipher.encrypt("Hello World")
print(ciphertext) # Output: 'Rclla Oaplx'
plaintext = cipher.decrypt(ciphertext)
print(plaintext) # Output: 'Hello World'
from cryptosystems import HillCipher
cipher = HillCipher([[3, 3], [2, 5]])
ciphertext = cipher.encrypt("HelloWorld")
print(ciphertext) # Output: 'HiozeIpjql'
plaintext = cipher.decrypt(ciphertext)
print(plaintext) # Output: 'HelloWorld'
from cryptosystems import VigenereCipher
cipher = VigenereCipher("key")
ciphertext = cipher.encrypt("Hello World")
print(ciphertext) # Output: 'Rijvs Uyvjk'
plaintext = cipher.decrypt(ciphertext)
print(plaintext) # Output: 'Hello World'
from cryptosystems import PlayfairCipher
cipher = PlayfairCipher("key")
ciphertext = cipher.encrypt("Hello World")
print(ciphertext) # Output: 'Dahak Ldskn'
plaintext = cipher.decrypt(ciphertext)
print(plaintext) # Output: 'Hello World'
from cryptosystems import AutoKeyCipher
cipher = AutoKeyCipher("key")
ciphertext = cipher.encrypt("Hello World")
print(ciphertext) # Output: 'Rijss Hzfhr'
plaintext = cipher.decrypt(ciphertext)
print(plaintext) # Output: 'Hello World'
from cryptosystems import DES
cipher = DES("password")
ciphertext = cipher.encrypt("Hello World")
print(ciphertext) # Output: b'\xf4\\V\x1a\xc7S\xb7\xdeZ\xc1\xe9\x14\n\x15Y\xe8'
plaintext = cipher.decrypt(ciphertext)
print(plaintext) # Output: 'Hello World'
from cryptosystems import AES
cipher = AES("passwordpassword")
ciphertext = cipher.encrypt("Hello World")
print(ciphertext) # Output: b'\x9cHS\xc2\x00\x0c\xba\x82Bj\x90\xc3t|4}'
plaintext = cipher.decrypt(ciphertext)
print(plaintext) # Output: 'Hello World'
from cryptosystems import RSA
rsa = RSA(1024)
ciphertext = rsa.encrypt(123)
print(ciphertext) # Output: 1234567890
plaintext = rsa.decrypt(ciphertext)
print(plaintext) # Output: 123
from cryptosystems import ElGamal
elgamal = ElGamal(1024)
ciphertext = elgamal.encrypt(123)
print(ciphertext) # Output: (123, 123)
plaintext = elgamal.decrypt(ciphertext)
print(plaintext) # Output: 123
from cryptosystems import Rabin
rabin = Rabin(1024)
ciphertext = rabin.encrypt(123)
print(ciphertext) # Output: 1234567890
plaintext = rabin.decrypt(ciphertext)
print(plaintext) # Output: 123
from cryptosystems import Paillier
paillier = Paillier(1024)
ciphertext = paillier.encrypt(123)
print(ciphertext) # Output: 1234567890
plaintext = paillier.decrypt(ciphertext)
print(plaintext) # Output: 123
from cryptosystems import DiffieHellman
diffiehellman = DiffieHellman()
shared_secret = diffiehellman.getSharedSecret()
print(shared_secret) # Output: 1234567890
from cryptosystems import MD5
md5 = MD5()
hash_value = md5.hash("Hello World")
print(hash_value) # Output: 'b10a8db164e0754105b7a99be72e3fe5'
from cryptosystems import SHA256
sha256 = SHA256()
hash_value = sha256.hash("Hello World")
print(hash_value) # Output: 'a591a6d40bf420404a011733cfb7b190d62c65bf0bcda32b57b277d9ad9f146e'
from cryptosystems import getRandomInteger
random_int = getRandomInteger(1024)
print(random_int)
from cryptosystems import getRandomRange
random_range = getRandomRange(1, 100)
print(random_range)
from cryptosystems import isPrime
prime_check = isPrime(17)
print(prime_check) # Output: True
from cryptosystems import getPrime
prime_number = getPrime(1024)
print(prime_number)
This project is licensed under the Apache License - see the LICENSE file for details.
FAQs
A module for cryptography built from scratch, offering a robust suite of classes and functions for both symmetric and asymmetric encryption, as well as hashing functionalities
We found that cryptosystems demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.