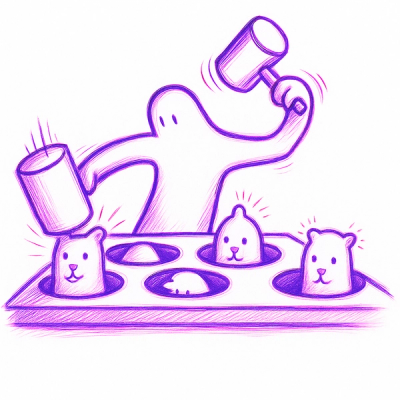
Research
/Security News
Contagious Interview Campaign Escalates With 67 Malicious npm Packages and New Malware Loader
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.
A python library for Gaussian Process Regression.
Create and activate virtualenv (for python2) or venv (for python3)
# for python3
python3 -m venv .env
# or for python2
python2 -m virtualenv .env
source .env/bin/activate
Upgrade pip
python -m pip install --upgrade pip
Install GPlib package
python -m pip install gplib
Matplotlib requires to install a backend to work interactively (See https://matplotlib.org/faq/virtualenv_faq.html). The easiest solution is to install the Tk framework, which can be found as python-tk (or python3-tk) on certain Linux distributions.
Import GPlib to use it in your python script.
import gplib
Initialize the GP with the desired modules.
gp = gplib.GP(
mean_function=gplib.mea.Fixed(),
covariance_function=gplib.ker.SquaredExponential()
)
Plot the GP.
gplib.plot.gp_1d(gp, n_samples=10)
Generate some random data.
import numpy as np
data = {
'X': np.arange(3, 8, 1.0)[:, None],
'Y': np.random.uniform(0, 2, 5)[:, None]
}
Get the posterior GP given the data.
posterior_gp = gp.get_posterior(data)
Finally plot the posterior GP.
gplib.plot.gp_1d(posterior_gp, data, n_samples=10)
There are more examples in examples/ directory. Check them out!
Download the repository using git
git clone https://gitlab.com/ibaidev/gplib.git
Update API documentation
source ./.env/bin/activate
pip install Sphinx
cd docs/
sphinx-apidoc -f -o ./ ../gplib
FAQs
Python library for Gaussian Process Regression.
We found that gplib demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.