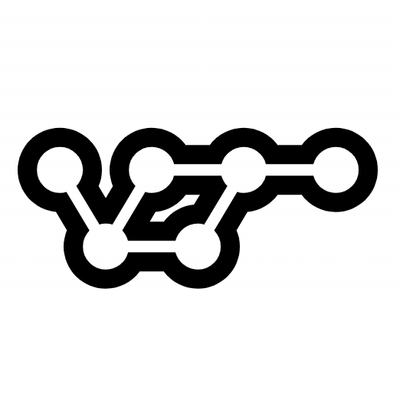
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Pyhopara is the Hopara Python SDK. It's main purpose is to ingest data from Python/Pandas.
pip install hopara
Before using Pyhopara you should setup your environment. This is achieved by installing the Hopara CLI and sign-in or sign-up:
npm install -g hopara
hopara signup
Basic usage:
import hopara as hp
hopara = hp.Hopara()
table = hp.Table('table_name')
table.add_column('column1', hp.ColumnType.INTEGER)
table.add_column('column2', hp.ColumnType.STRING)
hopara.create_table(table)
rows = [{'column1': 1, 'column2': 'a'},
{'column1': 2, 'column2': 'b'},
{'column1': 3, 'column2': 'c'}]
hopara.insert_rows(table, rows)
With pandas DataFrame:
import hopara as hp
import hopara.from_pandas as hpd
import pandas as pd
hopara = hp.Hopara()
df = pd.DataFrame({
'column1': [1, 2, 3],
'column2': ['a', 'b', 'c']
})
table = hpd.get_table('table_name', df)
hopara.create_table(table)
hopara.insert_rows(table, hpd.get_rows(df))
Please check the Hopara
and Table
sections for additional options.
FAQs
Hopara Python Library
We found that hopara demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.