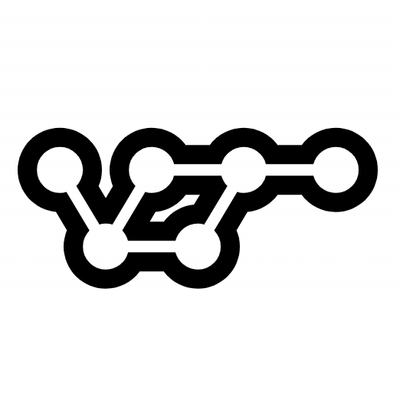
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Programmatically send out text messages via email.
|PyPI version|
Installation
``pip install mail_to_sms`` and import like any other Python module. Or,
``git clone https://github.com/naschorr/mail-to-sms`` locally as needed.
Make sure to install the requirements with
``pip install -r requirements.txt``
Arguments
~~~~~~~~~
- **number** {*string\|int*}: The destination phone number (ex.
``5551234567``).
- **carrier** {*string*}: The destination phone number's carrier (ex.
``"att"``). Current carriers are include: ``alltel``, ``att``,
``boost mobile``, ``cricket wireless``, ``metropcs``, ``project fi``,
``sprint``, ``tmobile``, ``us cellular``, ``verizon wireless``,
``virgin mobile``.
- **username** {*string*} [optional]: The username for accessing the
SMTP server (ex. ``"username"``). If omitted, it'll try to use the
username stored in the `.yagmail
file <https://github.com/kootenpv/yagmail#username-and-password>`__.
- **password** {*string*} [optional]: The password for accessing the
SMTP server (ex. ``"password"``). If using Gmail and 2FA, you may
want to use an app password. If omitted, it'll try to use `yagmail's
password <https://github.com/kootenpv/yagmail#username-and-password>`__
in the keyring, otherwise it'll prompt you for the password.
- **contents** {`*yagmail
contents* <https://github.com/kootenpv/yagmail#magical-contents>`__}
[optional]: A yagmail friendly contents argument (ex.
``"This is a message."``). If omitted, MailToSMS's ``send()`` method
can be called manually.
- keyworded args (for extra configuration):
- **quiet** {*boolean*}: Choose to disable printed statements. Defaults
to False. (ex. ``quiet=True``)
- **region** {*string*}: The region of the destination phone number.
Defaults to "US". (ex. ``region="US"``). This should only be
necessary when using a non international phone number that's not US
based. See the phonenumbers repo
`here <https://github.com/daviddrysdale/python-phonenumbers>`__.
- **mms** {*boolean*}: Choose to send a MMS message instead of a SMS
message, but will fallback to SMS if MMS isn't present. Defaults to
False. (ex. ``mms=True``)
- **subject** {*string*}: The subject of the email to send (ex.
``subject="This is a subject."``)
- **yagmail** {*list*}: A list of arguments to send to the
yagmail.SMTP() constructor. (ex.
``yagmail=["my.smtp.server.com", "12345"]``). As of 4/30/17, the args
and their defaults (after the username and password) are
``host='smtp.gmail.com'``, ``port='587'``, ``smtp_starttls=True``,
``smtp_set_debuglevel=0``, ``smtp_skip_login=False``,
``encoding="utf-8"``. This is unnecessary if you're planning on using
the basic Gmail interface, in which case you'll just need the
username and password. This may make more sense if you look at
yagmail's SMTP class
`here <https://github.com/kootenpv/yagmail/blob/master/yagmail/yagmail.py#L49>`__.
Examples
~~~~~~~~
::
from mail_to_sms import MailToSMS
::
MailToSMS(5551234567, "att", "username@gmail.com", "password", "this is a message")
::
MailToSMS("5551234567", "att", "username", "password", ["hello", "world"], subject="hey!")
::
MailToSMS(5551234567, "att", "username", "password", "hello world!", yagmail=["smtp.gmail.com", "587"])
::
MailToSMS("5551234567", "att", "username@gmail.com", "password", ["line one"], yagmail=["smtp.gmail.com"])
::
mail = MailToSMS(5551234567, "att", "username", "password")
mail.send("this is a string!")
Requirements
yagmail <https://github.com/kootenpv/yagmail>
__phonenumbers <https://github.com/daviddrysdale/python-phonenumbers>
__click <https://github.com/pallets/click>
__ (for the CLI)Note
I've only been able to test this on AT&T, so I can't guarantee that this
works for other carriers. Feedback is appreciated.
.. |PyPI version| image:: https://badge.fury.io/py/mail_to_sms.svg
:target: https://badge.fury.io/py/mail_to_sms
FAQs
Programmatically send out text messages via email.
We found that mail_to_sms demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.