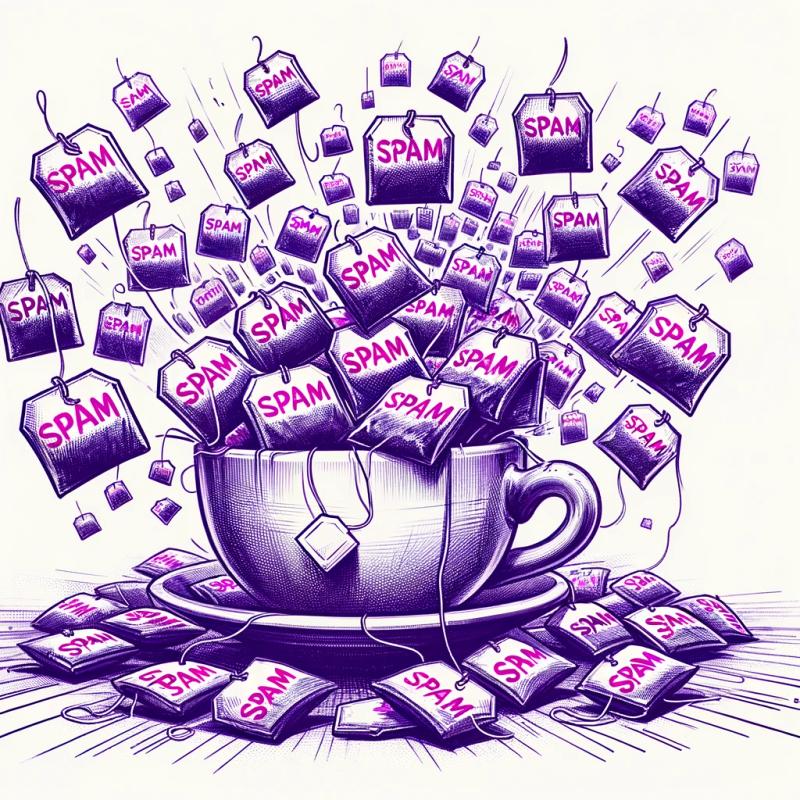
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Readme
Requires Python >= 3.10
TimeProfiler makes use of new features from Python's typing module.
pip install timer-module
import time
from timer_module import TimerModule
timer_module = TimerModule().start()
timer_module.pause()
time.sleep(0.2)
timer_module.start()
time.sleep(0.5)
seconds = timer_module.get_time()
print(seconds)
# Seconds
TimerModule().get_time() # float
# Milliseconds
TimerModule().get_time_ms() # float
# Nanoseconds
TimerModule().get_time_ns() # float
# Seconds
TimerModule().set_time(1.0)
# Milliseconds
TimerModule().set_time_ms(1.0)
# Nanoseconds
TimerModule().set_time_ns(1.0)
TimerModule().pause()
TimerModule().refresh()
TimerModule().reset()
TimeProfiler also includes a "function_profiler" and "async_function_profiler", in a class the asynchronous methods are handled automatically, but for functions you need to select the appropriate decorator.
import time
from timer_module import TimeProfiler
# You only need this line to profile the entire class
# The program is run normally, this decorator will wrap
# The class and its methods in order to track execution time
@TimeProfiler().class_profiler
class ExampleClass:
def __init__(self):
time.sleep(0.1)
def method_1(self):
time.sleep(0.5)
self.method_2()
def method_2(self):
time.sleep(1)
self.method_3()
def method_3(self):
time.sleep(1)
self.method_4()
def method_4(self):
time.sleep(0.1)
self.method_5()
def method_5(self):
time.sleep(0.1)
ec = ExampleClass()
ec.method_1()
FAQs
Timer Module with performance profiling features
We found that timer-module demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.