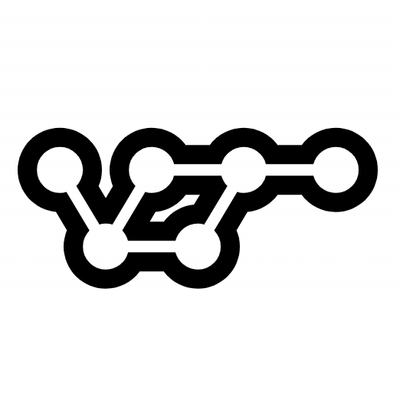
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
A Python tool for checking missing HTTP security headers for better web security.
A Python tool to check for missing HTTP security headers on websites. It can check for the presence of security headers like Strict-Transport-Security
, Content-Security-Policy
, X-Frame-Options
, X-Content-Type-Options
, Referrer-Policy
, and Permissions-Policy
.
To install the package, run:
pip install vulheader
You can use vulheader
both as a Python package and as a command-line tool.
You can use the check()
function to check for specific headers or all headers.
To check if a specific header is present or missing, use the following code:
import vulheader
url = "https://example.com"
result = vulheader.check(url, "Strict-Transport-Security")
if result == "missing":
print("Strict-Transport-Security: Missing")
else:
print("Strict-Transport-Security: Present")
You can replace "Strict-Transport-Security"
with any of the following headers to check for their presence:
Strict-Transport-Security
Content-Security-Policy
X-Frame-Options
X-Content-Type-Options
Referrer-Policy
Permissions-Policy
You can also check for all security headers at once:
import vulheader
url = "https://example.com"
header_status = vulheader.check(url)
for header, status in header_status.items():
print(f"{header}: {'Present' if status == 'present' else 'Missing'}")
Once installed, you can use vulheader
directly from the command line to check the headers of a website.
To check for all security headers:
vulheader --url https://example.com
To check for a specific header, use the -H
option followed by the header name:
vulheader --url https://example.com -H "Strict-Transport-Security"
Replace "Strict-Transport-Security"
with any of the following headers:
Strict-Transport-Security
Content-Security-Policy
X-Frame-Options
X-Content-Type-Options
Referrer-Policy
Permissions-Policy
Strict-Transport-Security: Missing
Content-Security-Policy: Present
X-Frame-Options: Missing
X-Content-Type-Options: Missing
Referrer-Policy: Missing
Permissions-Policy: Missing
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
A Python tool for checking missing HTTP security headers for better web security.
We found that vulheader demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.