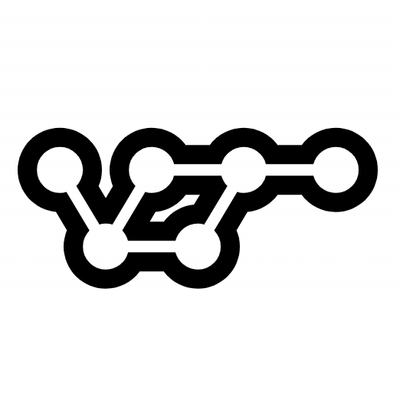
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Bloomy is an unofficial Ruby library for interacting with the Bloom Growth API. It provides convenient methods for getting user details, todos, rocks, meetings, measurables, and issues.
Add this line to your application's Gemfile:
bundle add bloomy
Or install it to your system:
gem install bloomy
You can find the full docs here but here's a quick overview to get you started.
First, you need to initialize the configuration and set up the API key.
require 'bloomy'
config = Bloomy::Configuration.new
You can configure the API key using your username and password. Optionally, you can store the API key locally under ~/.bloomy/config.yaml
for future use.
config.configure_api_key("your_username", "your_password", store_key: true)
You can also set an BG_API_KEY
environment variable and it will be loaded automatically for you once you initialize a client. A configuration is useful if you plan to use a fixed API key for your operations. However, you can also pass an API key when initializing a client without doing any configuration.
Once the configuration is set up, you can initialize the client. The client provides access to various features such as managing users, todos, rocks, meetings, measurables, and issues.
[!NOTE] Passing an API key is entirely optional and only useful if you plan to use different API keys to manage different organizations. This will bypass the regular configuration process.
client = Bloomy::Client.new(api_key: "abc...")
To interact with user-related features:
# Fetch current user details
current_user_details = client.user.details
# Search for users
search_results = client.user.search("John Doe")
To interact with meeting-related features:
# List all meetings for the current user
meetings = client.meeting.list
# Get details of a specific meeting
meeting_details = client.meeting.details(meeting_id)
To interact with todo-related features:
# List all todos for the current user
todos = client.todo.list
# Create a new todo
new_todo = client.todo.create(meeting_id: 1, title: "New Task", due_date: "2024-06-15")
To interact with goal-related features:
# List all goals for the current user
goals = client.goal.list
# Create a new goal
new_goal = client.goal.create(meeting_id: 1, title: "New Goal")
To interact with measurable-related features:
# Get user scorecard
user_scorecard = client.scorecard.list(user_id: 1)
# Get a meeting scorecard
meeting_scorecard = client.scorecard.list(meeting_id: 1)
To interact with issue-related features:
# Get details of a specific issue
issue_details = client.issue.details(issue_id)
# Create a new issue
new_issue = client.issue.create(meeting_id: 1, title: "New Issue")
FAQs
Unknown package
We found that bloomy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.