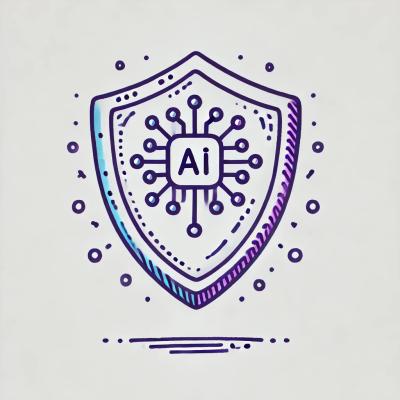
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
OpenAIHelperGem
is a Ruby module designed to simplify interactions with OpenAI's API. It provides functionality to upload files, send messages with structured outputs, generate speech from text, and automatically play the resulting audio on macOS.
file_id
for further use.Add the module to your project:
require_relative 'path_to_openai_helper_gem/openai_helper_gem'
Ensure you have the following environment variables set:
OPENAI_API_KEY
: Your OpenAI API key.GPT_MODEL
(optional): The OpenAI model you wish to use (defaults to gpt-3.5-turbo).OPENAI_AUDIO_PATH
(optional): Custom path to save generated audio (defaults to the user's home directory).Send a message and extract structured output:
require_relative 'openai_helper_gem'
messages = [{ role: 'user', content: 'Tell me a joke.' }]
schema = {
type: "object",
properties: {
joke: { type: "string" }
},
required: ["joke"]
}
response = OpenAIHelperGem::Client.perform_request(messages, schema)
puts response['joke']
Upload a file to OpenAI and retrieve the file_id
:
file_id = OpenAIHelperGem::Client.upload_file('path_to_your_file.pdf')
Generate speech from text and automatically play the audio:
OpenAIHelperGem::Client.generate_speech("This is a test message.")
Generate speech without playing it immediately:
OpenAIHelperGem::Client.generate_speech("This is a test message.", "output.mp3", autoplay: false)
Customize how errors and other notifications are handled:
Use terminal output:
OpenAIHelperGem::Client.notification_method = :puts
Disable notifications:
OpenAIHelperGem::Client.notification_method = :none
The module saves generated audio files to a default directory in the user's home folder:
OpenAIHelperGem::Client.default_audio_path = ENV['OPENAI_AUDIO_PATH'] || File.join(Dir.home, 'Local Resources', 'API Logs', 'OpenAI Whisper')
Here’s a more comprehensive example that combines file upload, schema validation, and text-to-speech generation:
require_relative 'openai_helper_gem'
# Step 1: Define messages and schema
messages = [
{ role: 'user', content: 'Please summarize the attached document.' }
]
schema = {
type: "object",
properties: {
summary: { type: "string" }
},
required: ["summary"]
}
# Step 2: Send message with the file and extract structured output
response = OpenAIHelperGem::Client.perform_request(messages, schema, 'path_to_your_document.pdf')
# Step 3: Output the summary
puts response['summary']
# Step 4: Convert the summary to speech and save it as an audio file
OpenAIHelperGem::Client.generate_speech(response['summary'], "document_summary.mp3")
This module is free to use and modify under the MIT License. See the LICENSE.txt
file for more details.
FAQs
Unknown package
We found that openai_helper_gem demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.