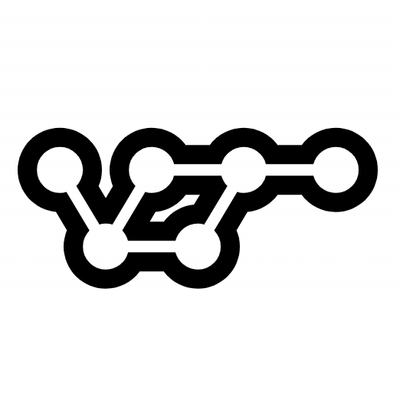
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
A simple to work with Excel files. Supported formats: xls
Add this line to your application's Gemfile:
gem 'simple-excel'
And then execute:
$ bundle
Or install it yourself as:
$ gem install simple-excel
Create instance of SimpleExcel:
excel = SimpleExcel.new('path/to/file', ['ID', 'Value'])
The second argument passed to the array with the headers that will be used to check the headers contained in the file.
The first argument can also provide a link to the file:
excel = SimpleExcel.new('http://link_to_file.com', ['ID', 'Value'])
To obtain data sufficient to call method each
:
excel.each do |row|
puts row
end
The variable row
contains a hash containing the headers and values:
{
'ID' => 1,
'Value' => 'Test',
'index' => 1 # The row number
}
By default, each
method compares the titles passed when creating an instance SimpleExcel with headers that are present in the file. If the headers do not match, an exception is thrown. This action can be undone, passed as the first argument to false
:
excel.each(false) do |row|
puts row
end
Also by default, the each
method checks filling the fields and if at least one field is not filled in, an exception is thrown. To undo this action, pass false
as the second argument:
excel.each(false, false) do |row|
puts row
end
If you want to check out not all fields, the third argument can be passed to the array with the headers that should be excluded from the check:
excel.each(true, true, ['ID']) do |row|
puts row
end
To changing the existing data or write new data, may be used such a construction:
excel.each(true, true, ['ID']) do |row|
# excel.worksheet.sheet.row(row['index'])[column number] = something
# For example:
excel.worksheet.sheet.row(row['index'])[0] = 7 # ID
excel.worksheet.sheet.row(row['index'])[1] = 'Angel' # Value
end
To save a file, you must call the method save_to_output
, which will save the file as a stream:
io = excel.save_to_output('path/to/file')
io.read # Print the contents of the file
git checkout -b my-new-feature
)git commit -am 'Add some feature'
)git push origin my-new-feature
)FAQs
Unknown package
We found that simple-excel demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.