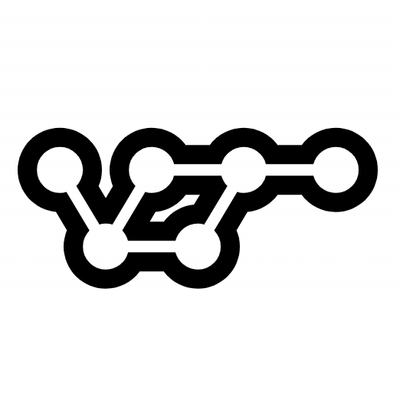
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
github.com/kuthumipepple/stocks-api
This API provides endpoints for managing stocks
To run this application as-is:
go mod download
command to download all the dependencies.stocks
. The columns should align with the Stock
model in the models package with the stockid
column set as SERIAL PRIMARY KEY
go run main.go
command to start the applicationEndpoint | Method | Description |
---|---|---|
/api/stocks | POST | Insert a new stock |
/api/stocks | GET | Get all stocks |
/api/stocks/{id} | GET | Get stock by ID |
/api/stocks/{id} | PUT | Update an existing stock |
/api/stocks/{id} | DELETE | Delete a stock |
Use any http client program to make HTTP requests to the running server. Here are some Request examples:
POST /api/stocks
{
"symbol": "AB",
"price": 19,
"company": "Company1"
}
201 Created
{
"id": 1,
"message": "stock created successfully"
}
GET /api/stocks
200 OK
[
{
"stockid": 1,
"symbol": "AB",
"price": 19,
"company": "Company1"
},
{
"stockid": 2,
"symbol": "CDE",
"price": 152,
"company": "Company2"
},
...
...
]
GET /api/stocks/1
200 OK
{
"stockid": 1,
"symbol": "AB",
"price": 19,
"company": "Company1"
}
PUT /api/stocks/1
{
"price": 25
}
200 OK
{
"id": 1,
"message": "stock updated successfully. Total records affected: 1"
}
DELETE /api/stocks/2
200 OK
{
"id": 2,
"message": "Stock deleted. Total records affected: 1"
}
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.