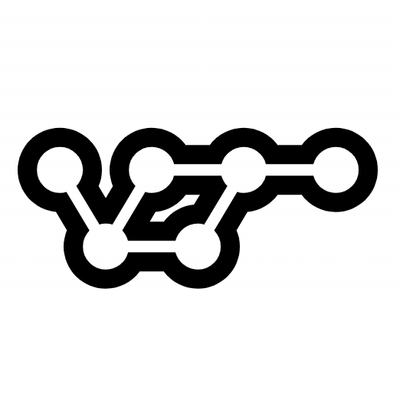
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@carbonaut/popover-dropdown
Advanced tools
This is a web-component built with Stencil that presents a custom popover that can receive a any callback functions that will be called on click.
npm i @carbonaut/popover-dropdown --save
To get our component up and running on your app, follow the steps for your framework (or Vanilla JS):
Vanilla JS:
script
tag inside your head
tag in your index.html
file:<script type='module' src='https://unpkg.com/@carbonaut/popover-dropdown@0.0.2/dist/popover-dropdown/popover-dropdown.esm.js'></script>
</head>
HTML
<popover-dropdown></popover-dropdown>
JavaScript
const popoverDropdown = document.querySelector('popover-dropdown');
popoverDropdown.currentOption = 'Deutsch';
popoverDropdown.options = [
{ label: 'Deutsch', callback: () => {} },
{ label: 'English', callback: () => {} },
{ label: 'Español', callback: () => {} },
];
Angular
defineCustomElements
function to your main.ts
file:import { defineCustomElements } from '@carbonaut/popover-dropdown/loader';
defineCustomElements(window);
module.ts
file you're going to use the component add CUSTOM_ELEMENTS_SCHEMA
to your schema configurationimport { CUSTOM_ELEMENTS_SCHEMA } from '@angular/core';
@NgModule({
imports: [
...
],
declarations: [...],
schemas: [CUSTOM_ELEMENTS_SCHEMA],
})
.ts
file;your-page.page.html
<popover-dropdown [options]="options" [currentOption]="firstOption"></popover-dropdown>
your-page.page.ts
export class ExamplePage {
options = [
{ label: 'Deutsch', callback: () => {} },
{ label: 'English', callback: () => {} },
{ label: 'Español', callback: () => {} },
];
firstOption: string = 'Deutsch';
option: {
label: string;
callback: () => {};
}
currentOption: string;
Property | Description |
---|---|
options | Option[] Array of objects that contains a label property of type string and a callback property that can be any function you want to be triggered when the option is selected. |
current-option | string The option you want to have displayed when you first open the component, this property must match with a label from the options property, otherwise it will be replaced by the first label on the options array. |
Stencil is a compiler for building fast web apps using Web Components.
Stencil combines the best concepts of the most popular frontend frameworks into a compile-time rather than run-time tool. Stencil takes TypeScript, JSX, a tiny virtual DOM layer, efficient one-way data binding, an asynchronous rendering pipeline (similar to React Fiber), and lazy-loading out of the box, and generates 100% standards-based Web Components that run in any browser supporting the Custom Elements v1 spec.
Stencil components are just Web Components, so they work in any major framework or with no framework at all.
FAQs
Generic popover dropdown menu made by Carbonaut.
The npm package @carbonaut/popover-dropdown receives a total of 53 weekly downloads. As such, @carbonaut/popover-dropdown popularity was classified as not popular.
We found that @carbonaut/popover-dropdown demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.