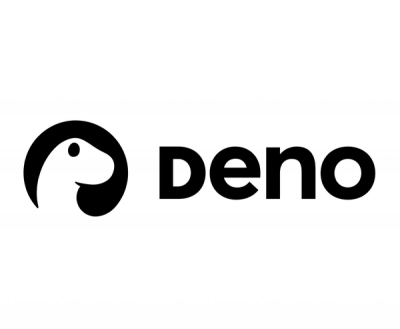
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@cognite/3d-viewer
Advanced tools
> Visualize Cognite's 3D models in a web browser with [WebGL](https://en.wikipedia.org/wiki/WebGL).
Visualize Cognite's 3D models in a web browser with WebGL.
$ npm install @cognite/3d-viewer
import { Cognite3DViewer } from '@cognite/3d-viewer';
const viewer = new Cognite3DViewer();
<script src="{path to this folder}/lib/cognite.bundle.js"></script> // Now you have 'cognite' as a global object
<script>
var viewer = new cognite.Cognite3DViewer();
</script>
const viewer = new Cognite3DViewer();
// The viewer will render to a canvas.
// Add this canvas to your DOM
document.body.appendChild(viewer.canvas);
// start rendering
viewer.start();
// At this point you will only see a black canvas.
// So let's add a 3D model:
const options = {
projectName, // Project name (tenant) in Cognite's Data Platform
modelId, // 3D model id (has to be under the same project)
revisionId, // The model's revision id
};
viewer.addModel(options).then(function(model) {
// Move camera to look at the model
viewer.fitCameraToModel(model, 0);
});
Note: you may need additional styling to the canvas to make it fit your use-case
canvas { width: 100%; height: 100%; }
function onProgress(progress) {
console.log(progress);
}
function onComplete() {
console.log('Model loaded');
}
const options = {
projectName,
modelId,
revisionId,
onProgress, // optional
onComplete, // optional
};
viewer.addModel(options)...
viewer.on('click', function(event) {
const { offsetX, offsetY } = event;
const nodeId = model.getNodeIdFromPixel(viewer.camera, viewer.renderer, offsetX, offsetY);
if (nodeId != null) {
// highlight the object
model.selectNode(nodeId);
// make the camera zoom to the object
const boundingBox = model.getBoundingBox(nodeId);
viewer.fitCameraToBoundingBox(boundingBox, 2000); // 2 sec
} else {
// Clicked outside the 3D model
}
});
Cognite3DViewer
is the root class of a Cognite 3D viewer. It controls all aspects of the 3D viewer.
options
Object (optional, default {}
)
const viewer = new Cognite3DViewer({
noBackground: true,
antialias: false,
});
Start viewer, or resume after calling stop().
viewer.start();
Stop the viewer. When stopped, the viewer will not do work, but it's state will be kept so it can restart immediately after start() is called.
viewer.stop();
Add event listener to the viewer call off to remove an event listener
type
"click"
func
function (event:MouseEvent) -- The callback functionconst onClick = event => { ... };
viewer.on('click', onClick);
Remove event listener from the viewer call on to add an event listener
viewer.off('click', onClick);
Add a new 3D model to the viewer. call fitCameraToModel to see the model after the model has loaded
options
Object
options.projectName
string -- The Cognite project the 3D model belongs tooptions.modelId
number -- The model's idoptions.revisionId
number -- The model's revision idoptions.sceneId
string -- Default to the latest scene (optional, default 'latest'
)options.onProgress
function (progress: Object)? -- Callback for progress eventsoptions.onComplete
function? -- Callback when the model is fully loadedconst options = {
projectName: 'COGNITE_TENANT_NAME',
modelId: 'COGNITE_3D_MODEL_ID',
revisionId: 'COGNITE_3D_REVISION_ID',
};
viewer.addModel(options).then(model => {
viewer.fitCameraToModel(model, 0);
});
Returns Promise<Cognite3DModel>
Move camera to a place where the content of a bounding box is visible to the camera
box
THREE.Box3 -- The bounding boxduration
number? -- The duration of the animation moving the camera. Set this to 0 (zero) to disable animation.radiusFactor
number? -- The the ratio of the distance from camera to center of box and radius of the box (optional, default 4
)Enables camera navigation with the keyboard.
Disables camera navigation with the keyboard.
Move camera to a place where the 3D model is visible. It uses the bounding box of the 3D model and calls fitCameraToBoundingBox
model
Cognite3DModel -- The 3D modelduration
number? -- Same as for fitCameraToBoundingBoxExtends THREE.Object3D
Cognite3DModel
is the class represeting a Cognite 3D model and it's state
Get bounding box of a node
nodeId
number -- The node's idbox
THREE.Box3? -- Optional target. Specify this to increase performance if the box can be reused.const box = model.getBoundingBox(nodeId);
const reusableBox = new THREE.Box3();
const box = model.getBoundingBox(nodeId, reusableBox);
// box === reusableBox
Returns THREE.Box3
Raycasting for nodes
camera
THREE.Camera -- The camera to project fromrenderer
THREE.WebGLRenderer -- A renderer. The raycaster will render an image to memory for efficiency.x
number -- X coordinate in pixelsy
number -- Y coordinate in pixelsconst box = model.getBoundingBox(nodeId);
const reusableBox = new THREE.Box3();
const box = model.getBoundingBox(nodeId, reusableBox);
// box === reusableBox
Returns (number | null) -- If there was a hit then returns the node id
Return the color on a node
nodeId
number -- The node's idReturns {r: number, g: number, b: number, a: number}
Set the color on a node
nodeId
number -- The node's idr
number -- The red color value (0-255)g
number -- The green color value (0-255)b
number -- The blue color value (0-255)a
number -- The alpha value (0-255) (optional, default 255
)Mark a node as selected
nodeId
number -- The node's idMark a node as deselected
nodeId
number -- The node's idMark all selected nodes as deselected
Get asset id assosiated with a node id in a Cognite 3D model.
projectName
string -- The Cognite project the 3D model belongs tomodelId
number -- The model's idrevisionId
number -- The model's revision idnodeId
number -- The node's idgetAssetInfoFromNodeId(projectName, modelId, revisionId, nodeId).then(result => {
if (result !== null) {
// result.assetId
// result.nodeId
}
});
Returns Promise<({nodeId: number, assetId: number} | null)>
Get all node id's assosiated with an asset id in a Cognite 3D model
projectName
string -- The Cognite project the 3D model belongs tomodelId
number -- The model's idrevisionId
number -- The model's revision idassetId
number -- The asset idgetNodeIdsFromAssetId(projectName, modelId, revisionId, assetId).then(nodeIds => {
nodeIds.forEach(nodeId => { ... });
});
Returns Promise<Array<number>> Array of nodeId's
Cognite3DModelLoader
is the class used to load a Cognite 3D model to Three.js.
options
Object? (optional, default {}
)
options.projectName
string -- The Cognite project the 3D model belongs tooptions.modelId
number -- The model's idoptions.revisionId
number -- The model's revision idoptions.sceneId
string -- Default to the latest scene (optional, default 'latest'
)options.onProgress
function (progress: Object)? -- Callback for progress eventsoptions.onComplete
function? -- Callback when the model is fully loadedconst options = {
projectName: 'COGNITE_TENANT_NAME',
modelId: 'COGNITE_3D_MODEL_ID',
revisionId: 'COGNITE_3D_REVISION_ID',
};
Cognite3DModelLoader.load(options).then(model => {
// if we have a THREE.Scene then:
scene.add(model);
});
Returns Promise<Cognite3DModel>
yarn
(npm install -g yarn
).yarn
to install packages.yarn run start
and go to http://localhost:3000
where the index.html file is hosted.Check out scripts
in package.json for more commands.
yarn publish
, make sure you have bumped the version first!FAQs
JavaScript viewer to visualize 3D models on Cognite Data Fusion
The npm package @cognite/3d-viewer receives a total of 109 weekly downloads. As such, @cognite/3d-viewer popularity was classified as not popular.
We found that @cognite/3d-viewer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 176 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.