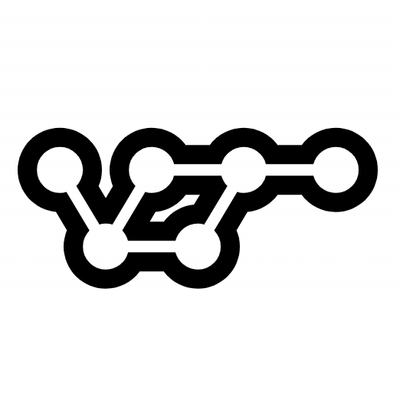
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@gasket/core
Advanced tools
npm install @gasket/core
Add a gasket.mjs
file to the root of your project.
This can be a .js
extension if your package.json has the type
field set to module
.
It is also possible to use with a .ts
extension if you have TypeScript configured.
// gasket.mjs
import { makeGasket } from '@gasket/core';
import LoggerPlugin from '@gasket/plugin-logger';
import MyPlugin from './my-plugin';
export default makeGasket({
plugins: [
LoggerPlugin,
MyPlugin
]
});
You can now import the Gasket instance from your gasket.mjs
file into your
application code.
With a Gasket, you can fire actions that will trigger lifecycles hooked
by plugins which encapsulate functionality allowing reuse across many applications.
A plugin is a module that exports a name
and hooks
object
(See Plugins Guide).
In your gasket.mjs
file, you can import plugins and add them to the plugins
array of the Gasket configuration.
When a new Gasket is created, there are three lifecycles executed in the following order:
The init
lifecycle allows the earliest entry to setting up a Gasket instance.
It can be used for setting up an initial state.
// gasket-plugin-example.mjs
export const name = 'gasket-plugin-example';
let _initializedTime;
export const hooks = {
init(gasket) {
_initializedTime = Date.now();
}
};
While it is possible to attach properties to the gasket
instance, it is not
recommended.
If a plugin needs to make properties available to other plugins, it should
register an action that can be executed to retrieve the value.
// gasket-plugin-example.mjs
export const name = 'gasket-plugin-example';
let _initializedTime;
export const hooks = {
init(gasket) {
- gasket.initializedTime = Date.now();
+ _initializedTime = Date.now();
},
+ actions() {
+ return {
+ getInitializedTime() {
+ return _initializedTime;
+ }
+ }
+ },
configure(gasket) {
- const time = gasket.initializedTime;
+ const time = gasket.actions.getInitializedTime();
}
};
The actions
lifecycle is the second lifecycle executed when a Gasket is created.
This will let plugins register actions that can be fired by the application code
where the Gasket is imported, or in other plugins.
// gasket-plugin-example.mjs
export const name = 'gasket-plugin-example';
export const hooks = {
actions(gasket) {
return {
async getDoodads() {
if(gasket.config.example) {
const dodaads = await gasket.exec('dodaads');
return dodaads.flat()
}
}
}
}
};
The configure
lifecycle is the first lifecycle executed when a Gasket is
instantiated.
This allows any registered plugins to adjust the configuration before further
lifecycles are executed.
// gasket-plugin-example.mjs
export const name = 'gasket-plugin-example';
export const hooks = {
configure(gasket, gasketConfig) {
// Modify the configuration
return {
...gasketConfig,
example: true
};
}
};
In this example, we register an action getDoodads
that will only execute if the
example
configuration is set to true
.
It will then execute the doodads
lifecycle, allowing any registered plugin to
provide doodads.
FAQs
Entry point to setting up Gasket instances
The npm package @gasket/core receives a total of 17 weekly downloads. As such, @gasket/core popularity was classified as not popular.
We found that @gasket/core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.