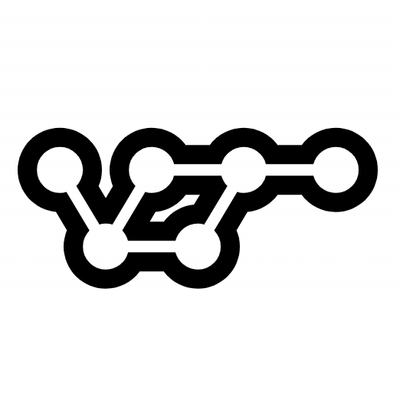
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@gnosis.pm/cow-sdk
Advanced tools
Install the SDK:
yarn add @gnosis.pm/cow-sdk
Instantiate the SDK:
import { CowSdk } from 'cow-sdk'
const chainId = 4 // Rinkeby
const cowSdk = new CowSdk(chainId)
The SDK will expose the CoW API operations (cowSdk.cowApi
)
// i.e. Get last 5 orders for a given trader
const trades = await cowSdk.cowApi.getOrders({
owner: '0x00000000005ef87f8ca7014309ece7260bbcdaeb', // Trader
limit: 5,
offset: 0
})
console.log(trades)
The SDK has also some convenient methods that will facilitate signing orders (cowSdk.signOrder
).
Let's see a full example on how to submit an order to CowSwap:
Before this example, the protocol requires you to approve the sell token before the order can be considered. For more details see https://docs.cow.fi/tutorials/how-to-submit-orders-via-the-api/1.-set-allowance-for-the-sell-token
import { Wallet } from 'ethers'
import { CowSdk, OrderKind } from 'cow-sdk'
const mnemonic = 'fall dirt bread cactus...'
const wallet = Wallet.fromMnemonic(mnemonic)
const cowSdk = new CowSdk(4, { signer: wallet })
// We will get a price quote for selling 1 WETH for USDC
const quoteResponse = await cowSdk.cowApi.getQuote({
kind: OrderKind.SELL,
amount: '1000000000000000000',
sellToken: '0xc778417e063141139fce010982780140aa0cd5ab', // WETH
buyToken: '0x4dbcdf9b62e891a7cec5a2568c3f4faf9e8abe2b', // USDC
userAddress: '0x1811be0994930fe9480eaede25165608b093ad7a',
validTo: 2524608000,
})
const order = {
kind: OrderKind.SELL,
sellToken: quoteResponse.quote.sellToken,
buyToken: quoteResponse.quote.buyToken,
validTo: quoteResponse.quote.validTo,
buyAmount: quoteResponse.quote.buyAmount,
sellAmount: quoteResponse.quote.sellAmount,
receiver: quoteResponse.quote.receiver,
partiallyFillable: false,
feeAmount: quoteResponse.quote.feeAmount,
}
const signedOrder = await cowSdk.signOrder(order)
const orderId = await cowSdk.cowApi.sendOrder({
order: { ...order, ...signedOrder },
owner: '0x1811be0994930fe9480eaede25165608b093ad7a',
})
// We can inspect the Order details in the CoW Protocol Explorer
console.log(`https://explorer.cow.fi/rinkeby/orders/${orderId}`)
yarn
yarn build
yarn start
yarn lint
yarn format
yarn test
FAQs
Unknown package
The npm package @gnosis.pm/cow-sdk receives a total of 3 weekly downloads. As such, @gnosis.pm/cow-sdk popularity was classified as not popular.
We found that @gnosis.pm/cow-sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 18 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.