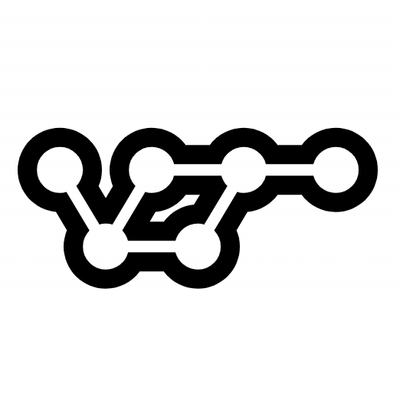
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@hmscore/cordova-plugin-hms-account
Advanced tools
Introduction
Installation Guide
Cordova SDK API Method Definition
Configure Description
Licensing and Terms
The Cordova SDK code encapsulates the Huawei Account Kit interface. It provides many APIs for your reference or use.
The Cordova SDK code package is described as follows:
src/main/java/com/huawei/hms/cordova/account: Core layer that exposes AccountSDK functionality to JS side.
www: Public interfaces for interacting AccountSDK through Cordova.
Download the Cordova Account SDK Plugin.
Add Platform To Project.
cordova platform add android
You can either install the plugin thorough npm or by downloading from downloads page, Cordova Account Plugin.
a. Run the following command in the root directory of your Cordova project to install it through npm.
cordova plugin add @hms-core/cordova-plugin-hms-account
b. Run the following command in the root directory of your Cordova project to install it manually after downloading the plugin.
cordova plugin add <CORDOVA_ACCOUNT_PLUGIN_PATH>
Check whether the Cordova Account SDK is successfully added to Plugin folder in the root directory of the Cordova project.
Add agconnect-services.json and jks file to root directory.
Add build.json file to your project's root.
Then run the Cordova app.
cordova run android
Download the Cordova Account Plugin through npm or by downloading from downloads page, Cordova Account Plugin.
a. Run the following command in the root directory of your Cordova project to install it through npm.
npm install @hmscore/cordova-plugin-hms-account
b. Run the following command in the root directory of your Cordova project to install it manually after downloading the plugin.
npm install <CORDOVA_ACCOUNT_PLUGIN_PATH>
Check whether the Cordova Account SDK is successfully added to the node_modules directory.
If you want full Ionic support with code completion etc, install"@ionic-native/core" in your project.
npm install @ionic-native/core --save
Copy the "ionic/dist/hms-account" folder from library to "node_modules/@ionic-native" folder under your Ionic project.
Compile ionic project.
a. ionic build
b. npx cap init [appName] [appId]
NOTE
where appName is the name of your app, and appId is package_name in your agconnect-services.json file (ex: com.example.app).
Add a native platform to your project.
ionic capacitor add android
Make sure your project has a build.gradle file with a maven repository address and Account service dependencies.
Add agconnect-services.json and jks file to the app directory in your Android project.
To update dependencies, and copy any web assets to your project, add following code.
npx capacitor sync
Then run the Ionic app.
ionic capacitor run android
Module | Definition |
---|---|
CommonTypes | A module for providing common data types and constants. |
HMSAccount | A module for interacting with the Huawei Sign In API. |
HMSHuaweiIdAuthManager | A module is used to entry point for the Huawei Sign In API. |
HMSHuaweiIdAuthTool | A module for obtaining and clearing authorization information. |
HMSNetworkTool | A module is used to construct a cookie and a url with specific parameters. |
HMSReadSMSManager | A module for Huawei ID SMS Service. |
HMSHuaweiIdAuthButton | A module is used to processing the the visual elements of the button for sign in. |
Return Type | Methods | Definition |
---|---|---|
Promise<AuthHuaweiId> | signIn | This API is called to sign in to the apps with HUAWEI IDs securely. |
Promise<void> | signOut | This API is called to sign out of a HUAWEI ID from an app. |
Promise<void> | cancelAuthorization | This API is called to cancel HUAWEI sign-in authorization. |
Promise<AuthHuaweiId> | silentSignIn | This API is called to silent authorization. The API will not display the related page. |
This API is called for HUAWEI sign-in.
Parameter | Type | Description |
---|---|---|
scope | ScopeConstants[] | ScopeConstants array to customize the authorization. |
param | HuaweiIdAuthParams | Authorization scope parameter to customize the authorization. |
scopeList | ScopeURI[] | ScopeURI array to customize the authorization. If this value not passed to API, an empty array will be send. |
Return | Description |
---|---|
Promise<AuthHuaweiId> | If the operation is successful, promise resolves to a AuthHuaweiId object. Otherwise it throws an error. |
This API is called to sign out of a HUAWEI ID from an app. After sign-out, the HMS SDK deletes the cached HUAWEI ID information.
Return | Description |
---|---|
Promise<void> | If the operation is successful, promise will resolve successfully. Otherwise it throws an error. |
This API is called to cancel HUAWEI sign-in authorization.
Return | Description |
---|---|
Promise<void> | If the operation is successful, promise will resolve successfully. Otherwise it throws an error. |
This API is called to return the information (or error information) about the HUAWEI ID used by a user who has signed in to an app. During this process, no user page will be displayed to the HUAWEI ID user.
Return | Description |
---|---|
Promise<AuthHuaweiId> | If the operation is successful, promise resolves to a AuthHuaweiId object. Otherwise it throws an error. |
Return Type | Methods | Definition |
---|---|---|
Promise<void> | addAuthScopes | This API is called to request the permission specified by scopeList from a HUAWEI ID. |
Promise<ContainScopesResult> | containScopes | This API is called to check whether the user with the designated HUAWEI ID has been assigned all permissions specified by scopeList. |
Promise<AuthHuaweiId> | getAuthResultWithScope | This API is called to obtain the AuthHuaweiId instance. |
Promise<AuthHuaweiId> | getAuthResult | This API is called to obtain the latest authorization information. |
This API is called to request the permission specified by scopeList from a HUAWEI ID.
Parameter | Type | Description |
---|---|---|
requestCode | Integer | Request Id. |
scopeList | ScopeURI[] | Authorization scope list. |
Return Type | Description |
---|---|
Promise<void> | If the operation is successful, promise will resolve successfully. Otherwise it throws an error. |
This API is called to checks whether the designated HUAWEI ID has been assigned all permissions specified by scopeList.
Parameter | Type | Description |
---|---|---|
authHuaweiId | AuthHuaweiIdBuilder | Authorized HUAWEI ID information. |
scopeList | ScopeURI[] | Authorization scope list. |
Return | Description |
---|---|
Promise<ContainScopesResult> | If the operation is successful, promise resolves to an object that contains boolean result, like {"containScopes": false }. Otherwise it throws an error. |
This API is called to obtain the AuthHuaweiId.
Parameter | Type | Description |
---|---|---|
scopeList | ScopeList[] | Authorization scope list. |
Return | Description |
---|---|
Promise<AuthHuaweiId> | If the operation is successful, promise resolves to a AuthHuaweiId object. Otherwise it throws an error. |
This API is called to obtain information about the HUAWEI ID used for the previous sign-in and authorization.
Return | Description |
---|---|
Promise<AuthHuaweiId> | If the operation is successful, promise resolves to a AuthHuaweiId object. Otherwise it throws an error. |
Return Type | Methods | Definition |
---|---|---|
Promise<String> | requestUnionId | This API is called to obtain a unionId. |
Promise<String> | requestAccessToken | This API is called to obtain a token. |
Promise<void> | deleteAuthInfo | This API is called to clear the local cache. |
Obtains a unionId.
Parameter | Type | Description |
---|---|---|
huaweiAccountName | String | HUAWEI ID Name. |
Return | Description |
---|---|
Promise<String> | If the operation is successful, promise resolves to a String that contains union id. Otherwise it throws an error. |
Obtains a token.
Parameter | Type | Description |
---|---|---|
account | Account | HUAWEI ID for which you need to obtain a token. It contains name and type properties. |
scopeList | ScopeURI[] | HUAWEI ID authorization scope. |
Return | Description |
---|---|
Promise<String> | If the operation is successful, promise resolves to a String that contains a access token. Otherwise it throws an error. |
Clears the local cache.
Parameter | Type | Description |
---|---|---|
accessToken | String | Token to be clear. |
Return | Description |
---|---|
Promise<void> | If the operation is successful, promise will resolve successfully. Otherwise it throws an error. |
Return Type | Methods | Definition |
---|---|---|
Promise<String> | buildNetworkCookie | This API is called to construct a cookie by combining input values. |
Promise<String> | buildNetworkURL | This API is called to cookie url based on the domain name and isUseHttps. |
Constructs a cookie by combining input values.
Parameter | Type | Description |
---|---|---|
cookie | Cookie | It is an object that contains cookieName, cookieValue, domain, path, isHttpOnly, isSecure, maxAge. |
Return | Description |
---|---|
Promise<String> | If the operation is successful, promise resolves to a String that contains a cookie. Otherwise it throws an error. |
Returns cookie url based on the domain name and isUseHttps.
Parameter | Type | Description |
---|---|---|
domainInfo | DomainInfo | The parameter that contains a domain name and isUseHttps info. |
Return | Description |
---|---|
Promise<String> | If the operation is successful, promise resolves to a String that contains a cookie url. Otherwise it throws an error. |
Return Type | Methods | Definition |
---|---|---|
Promise<String> | smsVerificationCode | This API is called to enable the service of reading SMS messages until the SMS messages that meet the rules (Timeout five minutes). |
Promise<String> | obtainHashCode | This API is called to obtain hash code. |
With this function,app can automatically retrieve SMS verification codes without requesting the permission of reading SMS messages. In case of get SMS Message, returned the SMS Message.
Return | Description |
---|---|
Promise<String> | If the operation is successful, promise resolves to a String that contains a content of SMS. Otherwise it throws an error. |
Obtains hash code which indicates the hash value generated by the HMS SDK based on app package name to uniquely identify app.
Return | Description |
---|---|
Promise<String> | If the operation is successful, promise resolves to a String that contains a hash value. Otherwise it throws an error. |
Return Type | Methods | Definition |
---|---|---|
void | getHuaweiIdAuthButton | This API is called to creating a specific button for login. |
Authenticates user identities. Note that this class only processes the the visual elements of the button.
Parameter | Type | Description |
---|---|---|
buttonId | String | Id of div that is used for HuaweiIdAuthButton. |
theme | Theme | Button theme |
colorPolicy | ColorPolicy | Values indicates button colors |
cornerRadius | CornerRadius | Values indicates button corner types |
Name | Definition |
---|---|
CommonTypes.ScopeConstants | Provides scopes to request a Huawei ID user. |
CommonTypes.HuaweiIdAuthParams | Provides default HuaweiID authorization parameters. |
CommonTypes.ScopeURI | Provides authorization scopes. |
CommonTypes.Gender | Provides gender information. |
HMSHuaweiIdAuthButton.Theme | Provides themes to set theme of the button. |
HMSHuaweiIdAuthButton.ColorPolicy | Provides colors to set color of the button. |
HMSHuaweiIdAuthButton.CornerRadius | Provides corner radiueses to set corner radius size of the button. |
Constant Fields | Value | Definition |
---|---|---|
SCOPE_PROFILE | "profile" | Requests a HUAWEI ID user to authorize profile information to an app. |
SCOPE_ID_TOKEN | "idToken" | Requests a HUAWEI ID user to authorize ID token to an app. |
SCOPE_ACCESS_TOKEN | "accessToken" | Requests a HUAWEI ID user to authorize access token to an app. |
SCOPE_MOBILE_NUMBER | "mobileNumber" | Requests a HUAWEI ID user to authorize mobile number to an app. |
SCOPE_EMAIL | "email" | Requests a HUAWEI ID user to authorize email address to an app. |
SCOPE_SHIPPING_ADDRESS | "shippingAddress" | Requests a HUAWEI ID user to authorize shipping address to an app. |
SCOPE_UID | "uid" | Requests a HUAWEI ID user to authorize uid token to an app. |
SCOPE_ID | "id" | Requests a HUAWEI ID user to authorize ID to an app. |
SCOPE_AUTHORIZATION_CODE | "authorizationCode" | Requests a HUAWEI ID user to authorize authorization code to an app. |
Constant Fields | Value | Definition |
---|---|---|
DEFAULT_AUTH_REQUEST_PARAM | "param" | Exposes HuaweiIdAuthParam.DEFAULT_AUTH_REQUEST_PARAM. Default authorization parameter of an AppTouch ID. |
DEFAULT_AUTH_REQUEST_PARAM_GAME | "game" | Exposes HuaweiIdAuthParam.DEFAULT_AUTH_REQUEST_PARAM_GAME. Default authorization parameter of a game. |
Constant Fields | Value | Definition |
---|---|---|
GAME | "https://www.huawei.com/auth/games" | Value to specify game scope. |
OPENID | "openid" | Value to specify openid scope. |
EMAIL | "email" | Value to specify email scope. |
PROFILE | "profile" | Value to specify profile scope. |
Constant Fields | Value | Definition |
---|---|---|
UNKNOWN | -1 | Unkown |
MALE | 0 | Male |
FEMALE | 1 | Female |
CONFIDENTIAL | 2 | Confidential |
Constant Fields | Value | Definition |
---|---|---|
THEME_NO_TITLE | 0 | Button without any title. |
THEME_FULL_TITLE | 1 | Button with an icon and a title. |
Constant Fields | Value | Definition |
---|---|---|
COLOR_POLICY_BLUE | 0 | Blue button. |
COLOR_POLICY_RED | 1 | Red button. |
COLOR_POLICY_WHITE | 2 | White button. |
COLOR_POLICY_WHITE_WITH_BORDER | 3 | White button with strokes. |
COLOR_POLICY_BLACK | 4 | Black button. |
COLOR_POLICY_GRAY | 5 | Gray button. |
Constant Fields | Value | Definition |
---|---|---|
CORNER_RADIUS_LARGE | -1 | Button with large rounded corners. |
CORNER_RADIUS_MEDIUM | -2 | Button with medium-sized rounded corners. |
CORNER_RADIUS_SMALL | -3 | Button with small rounded corners |
Field Name | Type | Description |
---|---|---|
openId | String | openId. |
uid | String | uid. |
displayName | String | Nickname. |
photoUrl | String | Obtains the picture url. |
accessToken | String | Access token. |
serviceCountryCode | String | Service country code. |
status | Integer | User status. 1: Normal; 2: Dbank suspended; 3: Deregistered, 4: All services are suspended. |
gender | Gender | User Gender. |
scopes | ScopeURI[] | Authorized scopes. |
serverAuthCode | String | serverAuthCode. |
unionId | String | unionId. |
countryCode | String | Registration country code. |
Field | Type | Description |
---|---|---|
accessToken | String | Access token. |
displayName | String | Nickname. |
String | Email adress. | |
familyName | String | Family name. |
givenName | String | Given name. |
idToken | String | ID Token |
unionId | String | unionId |
avatarUriString | String | Profile picture URI |
expressionTimeSecs | Number | expressionTimeSecs |
openId | String | openId |
uid | String | uid |
countryCode | String | Registration country code. |
serviceCountryCode | String | Service country code. |
status | Number | User status. 1: Normal; 2: Dbank suspended; 3: Deregistered, 4: All services are suspended. |
gender | Gender | User Gender. |
describeContentsInAuthHuaweiId | Number | describeContentsInAuthHuaweiId |
authorizedScopes | String[] | Authorized Scopes |
extensionScopes | String[] | extensionScopes |
authorizationCode | String | authorizationCode |
huaweiAccount | Account | huaweiAccount |
Field Name | Type | Description |
---|---|---|
type | String | Type of HUAWEI ID Account. |
name | String | Name of the HUAWEI ID Account. |
Field Name | Type | Description |
---|---|---|
cookieName | String | Cookie name. |
cookieValue | String | Cookie value. |
domain | String | Cookie domain name. |
path | String | Page path for accessing the cookie. |
isHttpOnly | Boolean | Value true indicates that the cookie information is contained only in the HTTP request header and cannot be accessed through document.cookie. |
isSecure | Boolean | Value true indicates that the cookie can be transmitted only through HTTPS, and value false indicates that the cookie can be transmitted through HTTP. |
maxAge | Long | Cookie lifetime, in seconds. |
Field Name | Type | Description |
---|---|---|
domain | String | Domain name. |
isUseHttps | Boolean | Value true indicates HTTPS, and value false indicates HTTP. |
Field Name | Type | Description |
---|---|---|
containScopes | Boolean | true: The HUAWEI ID has all the permissions specified by scopeArr. false: The HUAWEI ID does not have all the permissions specified by scopeArr. |
No.
Apache 2.0 license.
FAQs
Huawei Account Cordova Plugin
The npm package @hmscore/cordova-plugin-hms-account receives a total of 14 weekly downloads. As such, @hmscore/cordova-plugin-hms-account popularity was classified as not popular.
We found that @hmscore/cordova-plugin-hms-account demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.