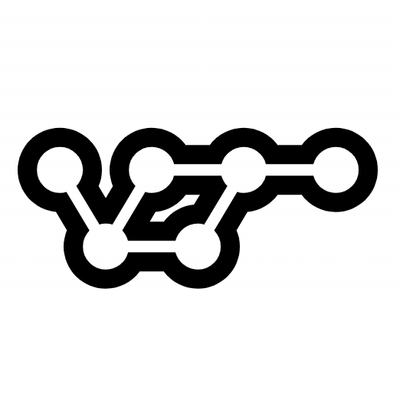
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@keyvaluesystems/react-scatter-graph
Advanced tools
A fully customizable ready to use scatter graph UI package for React. Try tweaking React Scatter Graph using this codesandbox link here
npm install @keyvaluesystems/react-scatter-graph
You’ll need to install React separately since it isn't included in the package.
React Scatter Graph can run in a very basic mode like this:
import React, { useState } from 'react';
import ReactScatterGraph from '@keyvaluesystems/react-scatter-graph';
function App() {
data = [
{ x: 450, y: 150 },
{ x: 360, y: 330 },
{ x: 650, y: 315 },
{ x: 270, y: 200 }
];
return <ScatterGraph data={data} graphHeight={500} />;
}
export default App;
The data
array is an array of objects with { x, y } cordinates.
Note: The graph width is responsive. So it can be adjusted by a parent wrapper. You need to provide the height.
Scatter graph is a useful tool for plotting date values. In order to do so, timestamps must be provided for the x-axis values.
import React, { useState } from 'react';
import ReactScatterGraph from '@keyvaluesystems/react-scatter-graph';
function App() {
data = [
// x given in milliseconds curresponding to the date
{ x: 1672876800000, y: 150 },
{ x: 1673568000000, y: 330 },
{ x: 1674086400000, y: 315 },
{ x: 1673222400000, y: 200}
];
return (
<ScatterGraph
data={data}
graphHeight={500}
/>
);
}
export default App;
Props that can be passed to the component are listed below:
Prop | Description | Default |
---|---|---|
data: object[] | An array of x-y cordinates to render. | undefined |
graphHeight: number | Height of graph in pixel | undefuned |
axisColor: string | Color for the x and y axes color which indicates the lines that are used to measure data | #9E9E9E |
originAxisColor: string | Color for the origin axis color | #9E9E9E |
renderYLabel?: (arg: number | string): string | Render function for customizing Y axis label | undefined |
renderXLabel?: (arg: number | string): string | Render function for customizing X axis label | undefined |
scatterPointColor?: (arg: { x: number, y: number }): string | Function for customizing scatter point color. Based on the args, we can customise the color. Return value should be the color hash / string. | undefined |
renderValuebox?: (x: number, y: number): ReactElement | Render function for customizing the value box shown on hover. | undefined |
FAQs
A fully customizable react scatter graph
The npm package @keyvaluesystems/react-scatter-graph receives a total of 1 weekly downloads. As such, @keyvaluesystems/react-scatter-graph popularity was classified as not popular.
We found that @keyvaluesystems/react-scatter-graph demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.