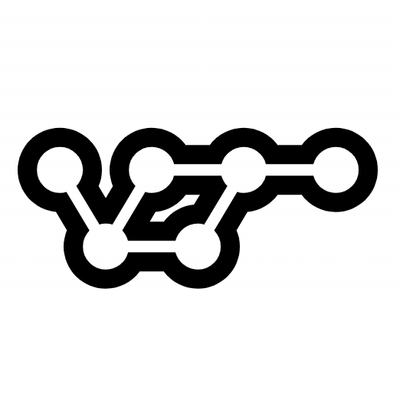
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@koopjs/cli
Advanced tools
An easy-to-use CLI tool to quickly build Koop applications and plugins
Use npm
npm install -g @koopjs/cli
Use yarn
yarn global add @koopjs/cli
Once installed the koop
command is available at the console.
Create a new Koop application with the name my-koop-app
# create a project folder and initialize it
koop new app my-koop-app
# cd in the folder
cd my-koop-app
Add a provider @koopjs/filesystem-s3 from npm
# install the provider and register it to the koop app
koop add provider @koopjs/filesystem-s3
Add a custom provider that connects to a local database
# add boilerplate provider files at src/providers/local-db and register it to
# the koop app (though you still need to implement the provider)
koop add provider providers/local-db --local
Test out your work
# run the koop server
koop serve
koop <command>
Commands:
koop new <type> <name> create a new koop project
koop add <type> <name> add a new plugin to the current app
koop test run tests in the current project
koop serve [path] run a Koop server for the current project
Options:
--quiet supress all console messages except errors
[boolean] [default: false]
The new
command creates a new Koop project from the template at the current location.
koop new <type> <name>
Positionals:
type project type
[string] [choices: "app", "provider", "auth", "output"]
name project name [string]
Options:
--config specify the project configuration in JSON [string]
--skip-install skip dependence installation [boolean] [default: false]
--skip-git do not initialize Git [boolean] [default: false]
--npm-client an executable that knows how to install npm package
dependencies[string] [choices: "npm", "yarn"] [default: "npm"]
For more details on the project templates, please take a look at the Koop specification and samples.
The add
command adds a Koop plugin to the current Koop app.
koop add <type> <name>
Positionals:
type plugin type [string] [choices: "output", "provider", "cache", "auth"]
name plugin name [string]
Provider Options:
--route-prefix add a prefix to all of a registered routes [string]
Options:
--config specify the plugin configuration in JSON [string]
--add-to-root add the given configuration to the app root configuration
[boolean] [default: false]
--skip-install skip plugin installation [boolean] [default: false]
The serve
command starts a test server for the current project.
For Koop apps, the command runs the app directly.
For Koop plugins, the command creates a Koop server that includes the plugin and a simple GeoJSON provider named dev-provider
(source). This GeoJSON provider is only registered if the current Koop project is not a provider project. It makes sure the Koop server has an output (geoservices by default) and a provider. When the GeoJSON provider is used, the data
file path is required to provide test data (must be *.geojson
).
If a more customized test server is needed, you can provide your test server file path to the command.
koop serve [path]
run a koop server for the current project
Positionals:
path server file path [string]
Options:
--port port number of the server [number]
--data path to a GeoJSON data file for testing Koop plugin [string]
--debug enable nodejs inspector for debugging [boolean]
--watch enable auto-restart on file change [boolean]
The test
command run tests in the current koop project.
koop test
This tool can be also used as a library.
const cli = require('@koopjs/cli')
// create a koop app project at /Documents
cli.new('/Documents', 'app', 'my-app', {
config: {
port: 8080
}
})
Create a Koop project at the given directory.
cwd
: current work directorytype
: project typename
: project nameReturn a promise.
Add a plugin to the given Koop app
cwd
: Koop app directorytype
: project typename
: Koop plugin nameReturn a promise.
FAQs
CLI tool to build Koop projects
The npm package @koopjs/cli receives a total of 80 weekly downloads. As such, @koopjs/cli popularity was classified as not popular.
We found that @koopjs/cli demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.