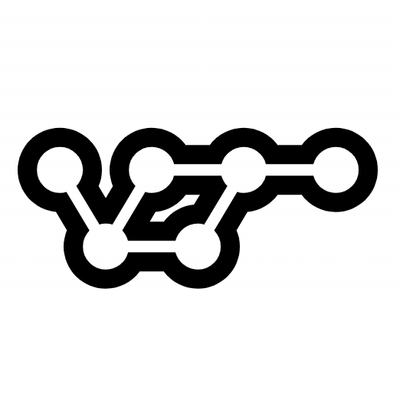
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@mayanfinance/swap-sdk
Advanced tools
A minimal package for sending cross-chain swap transactions
npm install --save @mayanfinance/swap-sdk
import the necessary functions and models:
import { fetchQuote, swapFromEvm, swapFromSolana, Quote } from '@mayanfinance/swap-sdk'
Then we will need to get a quote:
const quote = await getQuote({
amountIn: 250,
fromToken: fromToken.contract,
toToken: toToken.contract,
fromChain: "bsc",
toChain: "solana",
slippage: 3,
});
You can get the list of available tokens using Tokens API
Slippage is in percentage, so 3 means "up to three percent slippage".
After we get the quote we can send the swap transaction:
swapTrx = await swapFromSolana(quote, originWalletAddress, destinationWalletAddress, deadlineInSeconds, signSolanaTransaction)
swapTrx = await swapFromEvm(quote, destinationWalletAddress, deadlineInSeconds, provider, signer)
To track the progress of a swap, we can use Mayan Explorer API
FAQs
A SDK to swap with Mayan
The npm package @mayanfinance/swap-sdk receives a total of 5,206 weekly downloads. As such, @mayanfinance/swap-sdk popularity was classified as popular.
We found that @mayanfinance/swap-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.