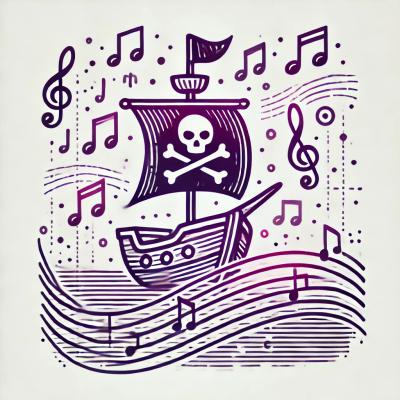
Research
Security News
Malicious PyPI Package Exploits Deezer API for Coordinated Music Piracy
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
@mediocre/walmart-marketplace
Advanced tools
[](https://github.com/mediocre/walmart-marketplace/actions/workflows/continuousIntegration.yaml) [;
const walmartMarketplace = new WalmartMarketplace({
clientId: 'your_api_key',
clientSecret: 'your_api_secret',
url: 'https://marketplace.walmartapis.com'
});
Get access token by providing Client ID and Client Secret.
https://developer.walmart.com/api/us/mp/auth
Promise Example
const accessToken = await walmartMarketplace.authentication.getAccessToken();
console.log(accessToken);
Callback Example
walmartMarketplace.authentication.getAccessToken(function(err, accessToken) {
console.log(accessToken);
});
Options
{
'WM_QOS.CORRELATION_ID': '00000000-0000-0000-0000-000000000000' // A unique ID which identifies each API call and used to track and debug issues. Defaults to a random UUID.
}
Returns
{
"access_token": "eyJraWQiOiI1MWY3MjM0Ny0wYWY5LTRhZ.....",
"token_type": "Bearer",
"expires_in": 900
}
Use this API for initial item setup and maintenance.
https://developer.walmart.com/api/us/mp/items#operation/itemBulkUploads
Promise Example
const mpItemMatch = {
MPItemFeedHeader: {
locale: 'en',
sellingChannel: 'mpsetupbymatch',
version: '4.2'
},
MPItem: [{
Item: {
condition: 'New',
price: 123,
productIdentifiers: {
productId: '123456789012',
productIdType: 'UPC'
},
ShippingWeight: 1,
sku: '123abc'
}
}]
};
const response = await walmartMarketplace.items.bulkItemSetup('MP_ITEM_MATCH', mpItemMatch);
console.log(response);
Callback Example
const mpItemMatch = {
MPItemFeedHeader: {
locale: 'en',
sellingChannel: 'mpsetupbymatch',
version: '4.2'
},
MPItem: [{
Item: {
condition: 'New',
price: 123,
productIdentifiers: {
productId: '123456789012',
productIdType: 'UPC'
},
ShippingWeight: 1,
sku: '123abc'
}
}]
};
walmartMarketplace.items.bulkItemSetup('MP_ITEM_MATCH', mpItemMatch, function(err, response) {
console.log(response);
});
Options
{
'WM_QOS.CORRELATION_ID': '00000000-0000-0000-0000-000000000000' // A unique ID which identifies each API call and used to track and debug issues. Defaults to a random UUID.
}
Returns
{
"feedId": "F129C19240844B97A3C6AD8F1A2C4997@AU8BAQA"
}
Retrieves an item and displays the item details.
https://developer.walmart.com/api/us/mp/items#operation/getAnItem
Promise Example
const itemDetails = await walmartMarketplace.items.getAnItem('97964_KFTest');
console.log(itemDetails);
Callback Example
walmartMarketplace.items.getAnItem('97964_KFTest', function(err, itemDetails) {
console.log(itemDetails);
});
Options
{
condition: 'New', // The value of product condition, (e.g. Restored). Enum: "New" "New without box" "New without tags" "Restored Premium" "Restored" "Remanufactured" "Open Box" "Pre-Owned: Like New" "Pre-Owned: Good" "Pre-Owned: Fair" "New with defects"
productIdType: 'SKU', // Item code type specifier allows to filter by specific code type, (e.g. GTIN). Enum: "GTIN" "UPC" "ISBN" "EAN" "SKU" "ITEM_ID"
'WM_QOS.CORRELATION_ID': '00000000-0000-0000-0000-000000000000' // A unique ID which identifies each API call and used to track and debug issues. Defaults to a random UUID.
}
Returns
{
"ItemResponse": [
{
"mart": "WALMART_US",
"sku": "30348_KFTest",
"condition": "New",
"wpid": "0RCPILAXM0C1",
"upc": "",
"gtin": "06932096330348",
"productName": "Kidsform Adjustable Infant Baby Carrier Sling Wrap Rider Carrier Backpack Front/Back Pack Khaki, Blue, Pink 4 Carrying Position Modes With Storage Bag",
"shelf": "[\"Home Page\",\"Baby\",\"Baby Activities & Gear\",\"Baby Carriers\"]",
"productType": "Baby Carriers",
"price": {
"currency": "USD",
"amount": 3
},
"publishedStatus": "PUBLISHED",
"lifecycleStatus": "ACTIVE"
}
],
"totalItems": 1
}
Completely deactivates and un-publishes an item from the site.
https://developer.walmart.com/api/us/mp/items#operation/retireAnItem
Promise Example
const response = await walmartMarketplace.items.retireAnItem('97964_KFTest');
console.log(response);
Callback Example
walmartMarketplace.items.retireAnItem('97964_KFTest', function(err, response) {
console.log(response);
});
Options
{
'WM_QOS.CORRELATION_ID': '00000000-0000-0000-0000-000000000000' // A unique ID which identifies each API call and used to track and debug issues. Defaults to a random UUID.
}
Returns
{
"sku": "97964_KFTest",
"message": "Thank you. Your item has been submitted for retirement from Walmart Catalog. Please note that it can take up to 48 hours for items to be retired from our catalog.",
"additionalAttributes": null,
"errors": null
}
Updates the regular price for a given item.
https://developer.walmart.com/api/us/mp/price#operation/updatePrice
Promise Example
const price = {
pricing: [
{
currentPrice: {
amount: 12.34,
currency: 'USD'
},
currentPriceType: 'BASE'
}
],
sku: '97964_KFTest'
};
const response = await walmartMarketplace.prices.updatePrice(price);
console.log(response);
Callback Example
const price = {
pricing: [
{
currentPrice: {
amount: 12.34,
currency: 'USD'
},
currentPriceType: 'BASE'
}
],
sku: '97964_KFTest'
};
walmartMarketplace.prices.updatePrice(price, function(err, response) {
console.log(response);
});
Options
{
'WM_QOS.CORRELATION_ID': '00000000-0000-0000-0000-000000000000' // A unique ID which identifies each API call and used to track and debug issues. Defaults to a random UUID.
}
Returns
{
"ItemPriceResponse": {
"mart": "WALMART_US",
"message": "Thank you. Your price has been updated. Please allow up to five minutes for this change to be reflected on the site.",
"sku": "97964_KFTest"
}
}
FAQs
[](https://github.com/mediocre/walmart-marketplace/actions/workflows/continuousIntegration.yaml) [![Coverage Status](https:/
The npm package @mediocre/walmart-marketplace receives a total of 94 weekly downloads. As such, @mediocre/walmart-marketplace popularity was classified as not popular.
We found that @mediocre/walmart-marketplace demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.
Security News
Newly introduced telemetry in devenv 1.4 sparked a backlash over privacy concerns, leading to the removal of its AI-powered feature after strong community pushback.