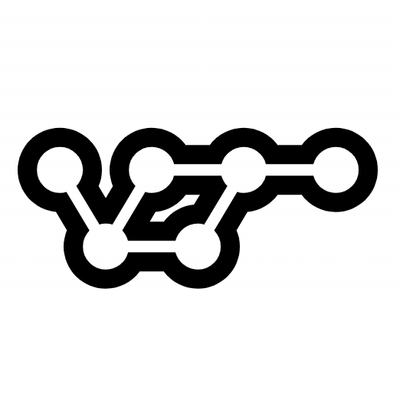
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@outlinerisk/auth0-tools
Advanced tools
Handles everything Auth0.
auth0-tools is a tool that wraps Auth0's ManagementClient, which is used to interact with the Management API. Its goal is to simplify the management of various Auth0 resources. To do this, auth0-tools provides:
Currently, we support:
// for the client package
npm i @outlinerisk/auth0-tools
// for the cli
npm i -g @outlinerisk/auth0-tools
// for the client package
yarn add @outlinerisk/auth0-tools
// for the cli
yarn global add @outlinerisk/auth0-tools
npm run build
You'll need to set a few values to secure a connection to Auth0's Management API:
Domain // The domain of your Auth0 tenant.
Client ID // The client ID of the Management API M2M app.
Client Secret // The client secret of the Management API M2M app.
Name Prefix // An optional prefix for the names of all your Auth0 resources.
You can pass these values in as optional arguments...
auth0-tools api deploy api_name api_audience --domain=domain --client-id=client_id --client-secret=client_secret
...but this can be tedious. Without these optional arguments, the CLI will default to the following environment variables:
process.env.AUTH0_DOMAIN
process.env.AUTH0_MANAGEMENT_CLIENT_ID
process.env.AUTH0_MANAGEMENT_CLIENT_SECRET
process.env.AUTH0_NAME_PREFIX
Check out the Auth0 documentation on actions to learn more.
To deploy actions, you'll need to provide JSON files that describes each action's properties.
# sample `action.json`
{
"code": "\nexports.onExecuteCredentialsExchange = async (event, api) => {\n const greeting = 'Hello World!'\n}",
"name": "My Action",
"runtime": "node16",
"supported_triggers": [
{
"id": "post-login",
"version": "v2"
}
]
}
To do this, create a .auth0-toolrc
configuration file. You must keep this file in your project root directory.
In the config file, create a actionsDir
variable and set it to the path of your actions directory, relative to the project's root directory.
# project
# ├---actions
# | | action1.json
# | | action2.json
# | ├---action3
# | | action3.json
# | └---action4
# | action4.json
# ├---folder1
# ...
# sample `.auth0-toolsrc`
actionsDir='./actions'
The CLI will recursively look within the actionsDir
for all JSON files. Note that if you have any non-action JSON files in the actionsDir
, the CLI will break.
It can be messy keeping code as a string. Instead of manually creating action.json
files, we recommend creating Action
objects, parsing them using a tool such as JSON.stringify()
, then writing the JSON string to a JSON file.
// create the action as an Action object
const action: Action = {
code: `
exports.onExecuteCredentialsExchange = async (event, api) => {
const greeting = 'Hello World!'
}`,
name: 'My Action',
runtime: 'node16',
supported_triggers: [
{
id: 'credentials-exchange',
version: 'v2',
},
],
}
// parse the action as a JSON string
const actionJSONString = JSON.stringify(action)
// write the JSON string to a JSON file
import fs from 'fs'
fs.writeFile('./action/action1/action1.json', actionJSONString, (err) => {
if (err) throw err
})
import { APIClient } from '@outlinerisk/auth0-tools'
const domain = process.env.AUTH0_DOMAIN
const clientId = process.env.AUTH0_MANAGEMENT_CLIENT_ID
const clientSecret = process.env.AUTH0_MANAGEMENT_CLIENT_SECRET
const apiClient = new APIClient(domain, clientId, clientSecret)
const apiName = 'My API'
const apiAudience = 'https://my-website.com/my/api'
await apiClient.deployAPI(apiName, apiAudience)
Passing a prefix to any client constructor will prepend all resources for that client with the prefix.
import { AppClient } from '@outlinerisk/auth0-tools'
const domain = process.env.AUTH0_DOMAIN
const clientId = process.env.AUTH0_MANAGEMENT_CLIENT_ID
const clientSecret = process.env.AUTH0_MANAGEMENT_CLIENT_SECRET
const prefix = 'dev'
const appClient = new AppClient(domain, clientId, clientSecret, prefix)
const appName = 'My App'
const clientSecret = 'TotallyLegitSecret'
await appClient.deployM2MApp(appName, clientSecret) // spins up a m2m app named 'dev-My App'
While names are not the unique identifiers for resources, they are the easiest property to identify resources with. As such, we believe that resources should have unique names to make the management of resources clearer. auth0-tools enforces this ideology by applying unique name constraints to all resources within a given type. That is, no API can share the same name, nor can any app, but an API and an app can share the same name, though we wouldn't recommend that either.
import { APIClient } from '@outlinerisk/auth0-tools'
const domain = process.env.AUTH0_DOMAIN
const clientId = process.env.AUTH0_MANAGEMENT_CLIENT_ID
const clientSecret = process.env.AUTH0_MANAGEMENT_CLIENT_SECRET
const apiClient = new APIClient(domain, clientId, clientSecret)
const apiName = 'My API'
const apiAudience = 'https://my-website.com/my/api'
await apiClient.deployAPI(apiName, apiAudience) // successfully deploys 'My API'
const apiAudience2 = 'https://my-website.com/my/api/2'
await apiClient.deployAPI(apiName, apiAudience2) // while valid within Auth0, auth0-tools throws an error
Note that while Auth0 does allow for resources to share names, there are complications. For instance, if you have multiple APIs deployed with the same name, you cannot manually delete the APIs with shared names in the web console--the delete button becomes grayed out. You'd have to change the names of the APIs until they are all unique, then delete them.
auth0-tools help
Usage: main [options] [command]
Pathpoint's CLI tool for managing Auth0 resources.
Options:
-V, --version output the version number
-h, --help display help for command
Commands:
action Run action commands.
api Run API commands.
app Run app commands.
help [command] display help for command
FAQs
Pathpoint's internal Auth0 tooling.
The npm package @outlinerisk/auth0-tools receives a total of 257 weekly downloads. As such, @outlinerisk/auth0-tools popularity was classified as not popular.
We found that @outlinerisk/auth0-tools demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.