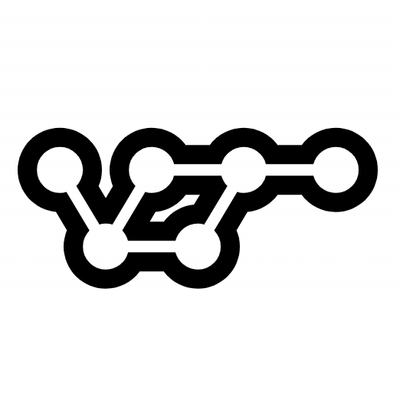
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@prezzee/ts-v2-sdks
Advanced tools
This SDK supports Prezzee's V2 Business APIs enabling quicker development. It allows the developers to:
This SDK supports Prezzee's V2 Business APIs enabling quicker development. It allows the developers to:
npm install @prezzee/ts-v2-sdks
Install dependencies as well:
npm install axios
To use the functions, you need a Business API token as part of your config. Add an environment variable TOKEN={business API token you must have received}. Example token: sJ0uOIDRYexgfL2M7BgLiPjb6xkPAi
const prezzeeClient = createPrezzeeClient({ token: process.env.TOKEN, env: "sandbox" });
Once initialised, you can use it as per following examples:
const products = await prezzeeClient.listProducts();
Returns a list of products currently available for purchase.
const styles = await prezzeeClient.listStyles();
Returns a list of gift styles for use with the product creation endpoint.
const product = products.results[0];
const style = styles.results[0];
const order = await prezzeeClient.createOrder({
reference: generateUUID(),
payment_method: "POSTPAID_CREDIT",
wait_for_stock: false,
items: [
{
reference: generateUUID(),
product_code: product.code,
product_theme_code: product.themes[0].code,
amount: product.denominations[0].amount,
currency: product.denominations[0].currency,
delivery_method: "LINK",
delivery_details: {
style_code: style.code,
message: "Hello Prezzee SDK",
recipient_name: "Prezzee SDK Recipient",
recipient_email: "dhanushka.krishnaith@prezzee.com",
sender_name: "Prezzee SDK Sender",
},
},
],
});
This will return order_uuid in response which can be used in the following example to retrieve the order details.
await prezzeeClient.retrieveOrder({ orderUuid: order.uuid, });
FAQs
This SDK supports Prezzee's V2 Business APIs enabling quicker development. It allows the developers to:
The npm package @prezzee/ts-v2-sdks receives a total of 1 weekly downloads. As such, @prezzee/ts-v2-sdks popularity was classified as not popular.
We found that @prezzee/ts-v2-sdks demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 61 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.