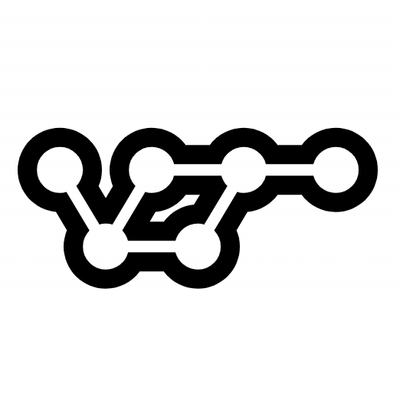
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@react-native-picker/picker
Advanced tools
React Native Picker for iOS, Android, macOS, and Windows
@react-native-picker/picker is a React Native package that provides a cross-platform Picker component for selecting values from a list. It supports both iOS and Android, offering a consistent user experience across platforms.
Basic Picker
This code demonstrates a basic Picker component where users can select between 'Java' and 'JavaScript'. The selected value is displayed above the Picker.
import React, { useState } from 'react';
import { View, Text } from 'react-native';
import { Picker } from '@react-native-picker/picker';
const BasicPicker = () => {
const [selectedValue, setSelectedValue] = useState('java');
return (
<View>
<Text>Selected: {selectedValue}</Text>
<Picker
selectedValue={selectedValue}
onValueChange={(itemValue, itemIndex) => setSelectedValue(itemValue)}
>
<Picker.Item label="Java" value="java" />
<Picker.Item label="JavaScript" value="js" />
</Picker>
</View>
);
};
export default BasicPicker;
Custom Picker Styles
This code demonstrates how to apply custom styles to the Picker component and its container. The Picker is styled to have a specific height and width, and the selected value is displayed with custom text styling.
import React, { useState } from 'react';
import { View, Text, StyleSheet } from 'react-native';
import { Picker } from '@react-native-picker/picker';
const CustomPicker = () => {
const [selectedValue, setSelectedValue] = useState('java');
return (
<View style={styles.container}>
<Text style={styles.text}>Selected: {selectedValue}</Text>
<Picker
selectedValue={selectedValue}
style={styles.picker}
onValueChange={(itemValue, itemIndex) => setSelectedValue(itemValue)}
>
<Picker.Item label="Java" value="java" />
<Picker.Item label="JavaScript" value="js" />
</Picker>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
text: {
fontSize: 18,
marginBottom: 10,
},
picker: {
height: 50,
width: 150,
},
});
export default CustomPicker;
Picker with Multiple Items
This code demonstrates a Picker component with multiple items. Users can select from 'Java', 'JavaScript', 'Python', and 'C++'. The selected value is displayed above the Picker.
import React, { useState } from 'react';
import { View, Text } from 'react-native';
import { Picker } from '@react-native-picker/picker';
const MultiItemPicker = () => {
const [selectedValue, setSelectedValue] = useState('java');
return (
<View>
<Text>Selected: {selectedValue}</Text>
<Picker
selectedValue={selectedValue}
onValueChange={(itemValue, itemIndex) => setSelectedValue(itemValue)}
>
<Picker.Item label="Java" value="java" />
<Picker.Item label="JavaScript" value="js" />
<Picker.Item label="Python" value="python" />
<Picker.Item label="C++" value="cpp" />
</Picker>
</View>
);
};
export default MultiItemPicker;
react-native-picker-select is another popular package for creating Picker components in React Native. It offers more customization options and supports placeholder text, custom icons, and styling. Compared to @react-native-picker/picker, it provides a more flexible and feature-rich API but may require more setup.
react-native-modal-selector provides a modal-based Picker component for React Native. It allows users to select values from a list displayed in a modal dialog. This package is useful for scenarios where a modal-based selection is preferred over a dropdown. It offers a different user experience compared to @react-native-picker/picker.
@react-native-picker/picker
Android | iOS | PickerIOS |
---|---|---|
![]() | ![]() | ![]() |
Windows | MacOS |
---|---|
![]() | ![]() |
@react-native-picker/picker | react-native | react-native-windows |
---|---|---|
>= 2.0.0 | 0.61+ | 0.64+ |
>= 1.16.0 | 0.61+ | 0.61+ |
>= 1.2.0 | 0.60+ or 0.59+ with Jetifier | N/A |
>= 1.0.0 | 0.57 | N/A |
$ npm install @react-native-picker/picker --save
or
$ yarn add @react-native-picker/picker
As react-native@0.60 and above supports autolinking there is no need to run the linking process.
Read more about autolinking here. This is supported by react-native-windows@0.64
and above.
CocoaPods on iOS needs this extra step:
npx pod-install
No additional step is required.
Usage in Windows without autolinking requires the following extra steps:
ReactNativePicker
project to your solution.D:\dev\RNTest\node_modules\@react-native-picker\picker\windows\ReactNativePicker\ReactNativePicker.vcxproj
Add a reference to ReactNativePicker
to your main application project. From Visual Studio 2019:
Right-click main application project > Add > Reference...
Check ReactNativePicker
from Solution Projects.
Add #include "winrt/ReactNativePicker.h"
to the headers included at the top of the file.
Add PackageProviders().Append(winrt::ReactNativePicker::ReactPackageProvider());
before InitializeComponent();
.
CocoaPods on MacOS needs this extra step (called from the MacOS directory)
pod install
The following steps are only necessary if you are working with a version of React Native lower than 0.60
$ react-native link @react-native-picker/picker
Libraries
➜ Add Files to [your project's name]
node_modules
➜ @react-native-picker/picker
and add RNCPicker.xcodeproj
libRNCPicker.a
to your project's Build Phases
➜ Link Binary With Libraries
Cmd+R
)<android/app/src/main/java/[...]/MainApplication.java
)import com.reactnativecommunity.picker.RNCPickerPackage;
to the imports at the top of the filenew RNCPickerPackage()
to the list returned by the getPackages()
methodandroid/settings.gradle
:
include ':@react-native-picker_picker'
project(':@react-native-picker_picker').projectDir = new File(rootProject.projectDir, '../node_modules/@react-native-picker/picker/android')
android/app/build.gradle
:
implementation project(path: ':@react-native-picker_picker')
Libraries
➜ Add Files to [your project's name]
node_modules
➜ @react-native-picker/picker
and add RNCPicker.xcodeproj
libRNCPicker.a
to your project's Build Phases
➜ Link Binary With Libraries
Cmd+R
)<you need to add android:supportsRtl="true"
to AndroidManifest.xml
<application
...
android:supportsRtl="true">
Import Picker from @react-native-picker/picker
:
import {Picker} from '@react-native-picker/picker';
Create state which will be used by the Picker
:
const [selectedLanguage, setSelectedLanguage] = useState();
Add Picker
like this:
<Picker
selectedValue={selectedLanguage}
onValueChange={(itemValue, itemIndex) =>
setSelectedLanguage(itemValue)
}>
<Picker.Item label="Java" value="java" />
<Picker.Item label="JavaScript" value="js" />
</Picker>
If you want to open/close picker programmatically on android (available from version 1.16.0+), pass ref to Picker
:
const pickerRef = useRef();
function open() {
pickerRef.current.focus();
}
function close() {
pickerRef.current.blur();
}
return <Picker
ref={pickerRef}
selectedValue={selectedLanguage}
onValueChange={(itemValue, itemIndex) =>
setSelectedLanguage(itemValue)
}>
<Picker.Item label="Java" value="java" />
<Picker.Item label="JavaScript" value="js" />
</Picker>
onValueChange
Callback for when an item is selected. This is called with the following parameters:
itemValue
: the value
prop of the item that was selecteditemPosition
: the index of the selected item in this pickerType | Required |
---|---|
function | No |
selectedValue
Value matching value of one of the items. Can be a string or an integer.
Type | Required |
---|---|
any | No |
style
Type | Required |
---|---|
pickerStyleType | No |
testID
Used to locate this view in end-to-end tests.
Type | Required |
---|---|
string | No |
enabled
If set to false, the picker will be disabled, i.e. the user will not be able to make a selection.
Type | Required | Platform |
---|---|---|
bool | No | Android, Web, Windows |
mode
On Android, specifies how to display the selection items when the user taps on the picker:
Type | Required | Platform |
---|---|---|
enum('dialog', 'dropdown') | No | Android |
dropdownIconColor
On Android, specifies color of dropdown triangle. Input value should be value that is accepted by react-native processColor
function.
Type | Required | Platform |
---|---|---|
ColorValue | No | Android |
dropdownIconRippleColor
On Android, specifies ripple color of dropdown triangle. Input value should be value that is accepted by react-native processColor
function.
Type | Required | Platform |
---|---|---|
ColorValue | No | Android |
prompt
Prompt string for this picker, used on Android in dialog mode as the title of the dialog.
Type | Required | Platform |
---|---|---|
string | No | Android |
itemStyle
Style to apply to each of the item labels.
Type | Required | Platform |
---|---|---|
text styles | No | iOS, Windows |
numberOfLines
On Android & iOS, used to truncate the text with an ellipsis after computing the text layout, including line wrapping, such that the total number of lines does not exceed this number. Default is '1'
Type | Required | Platform |
---|---|---|
number | No | Android, iOS |
onBlur
Type | Required | Platform |
---|---|---|
function | No | Android |
onFocus
Type | Required | Platform |
---|---|---|
function | No | Android |
selectionColor
Type | Required | Platform |
---|---|---|
ColorValue | No | iOS |
blur
(Android only, lib version 1.16.0+)Programmatically closes picker
focus
(Android only, lib version 1.16.0+)Programmatically opens picker
Props that can be applied to individual Picker.Item
label
Displayed value on the Picker Item
Type | Required |
---|---|
string | Yes |
value
Actual value on the Picker Item
Type | Required |
---|---|
number,string | Yes |
color
Displayed color on the Picker Item
Type | Required |
---|---|
ColorValue | No |
fontFamily
Displayed fontFamily on the Picker Item
Type | Required |
---|---|
string | No |
style
Style to apply to individual item labels.
Type | Required | Platform |
---|---|---|
ViewStyleProp | No | Android |
enabled
If set to false, the specific item will be disabled, i.e. the user will not be able to make a selection
@default: true
Type | Required | Platform |
---|---|---|
boolean | No | Android |
contentDescription
Sets the content description to the Picker Item
Type | Required | Platform |
---|---|---|
string | No | Android |
itemStyle
Type | Required |
---|---|
text styles | No |
onValueChange
Type | Required |
---|---|
function | No |
selectedValue
Type | Required |
---|---|
any | No |
selectionColor
Type | Required | Platform |
---|---|---|
ColorValue | No | iOS |
themeVariant
Type | Required |
---|---|
enum('light', 'dark') | No |
FAQs
React Native Picker for iOS, Android, macOS, and Windows
The npm package @react-native-picker/picker receives a total of 418,394 weekly downloads. As such, @react-native-picker/picker popularity was classified as popular.
We found that @react-native-picker/picker demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.