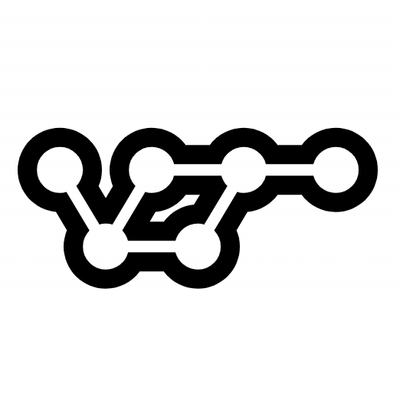
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@settlemint/sdk-hasura
Advanced tools
SettleMint SDK, integrate SettleMint into your application with ease.
✨ https://settlemint.com ✨
Integrate SettleMint into your application with ease.
To install the SettleMint SDK, you can use one of the following package managers:
# Using npm
npm install @settlemint/sdk
# Using yarn
yarn add @settlemint/sdk
# Using pnpm
pnpm add @settlemint/sdk
# Using Bun
bun add @settlemint/sdk
We recommend using Bun for faster installation and better performance.
To use the SettleMint SDK in your Node.js application, follow these steps:
import { createSettleMintClient } from '@settlemint/sdk';
const client = createSettleMintClient({
accessToken: 'your_access_token',
instance: 'https://console.settlemint.com'
});
// Example: List workspaces
const workspaces = await client.workspace.list();
console.log(workspaces);
The SettleMint SDK provides access to various resources. Here's an overview of the available methods:
workspace.list()
: List all workspaces and their applicationsworkspace.read(workspaceId)
: Read a specific workspace and its applicationsblockchainNetwork.list(applicationId)
: List blockchain networks for a given applicationblockchainNetwork.read(blockchainNetworkId)
: Read a specific blockchain networkblockchainNode.list(applicationId)
: List blockchain nodes for a given applicationblockchainNode.read(blockchainNodeId)
: Read a specific blockchain nodemiddleware.list(applicationId)
: List middlewares for a given applicationmiddleware.read(middlewareId)
: Read a specific middlewareintegrationTool.list(applicationId)
: List integration tools for a given applicationintegrationTool.read(integrationId)
: Read a specific integration toolstorage.list(applicationId)
: List storage items for a given applicationstorage.read(storageId)
: Read a specific storage itemprivateKey.list(applicationId)
: List private keys for a given applicationprivateKey.read(privateKeyId)
: Read a specific private keyinsights.list(applicationId)
: List insights for a given applicationinsights.read(insightsId)
: Read a specific insightHere are some examples of how to use the SettleMint SDK:
const client = createSettleMintClient({
accessToken: 'your_access_token',
instance: 'https://console.settlemint.com'
});
const workspaces = await client.workspace.list();
console.log(workspaces);
const client = createSettleMintClient({
accessToken: 'your_access_token',
instance: 'https://console.settlemint.com'
});
const networkId = 'your_network_id';
const network = await client.blockchainNetwork.read(networkId);
console.log(network);
We welcome contributions to the SettleMint SDK! If you'd like to contribute, please follow these steps:
Please ensure that your code follows the existing style and includes appropriate tests and documentation.
The SettleMint SDK is released under the FSL Software License. See the LICENSE file for more details.
FAQs
Hasura and PostgreSQL integration module for SettleMint SDK, enabling database operations and GraphQL queries
The npm package @settlemint/sdk-hasura receives a total of 9,301 weekly downloads. As such, @settlemint/sdk-hasura popularity was classified as popular.
We found that @settlemint/sdk-hasura demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.