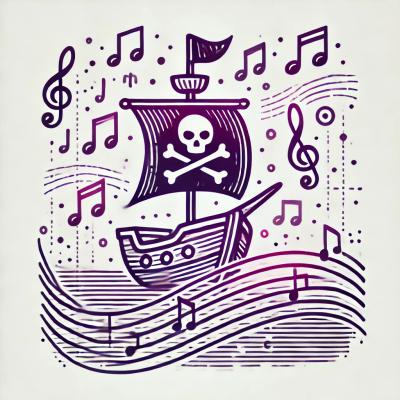
Research
Security News
Malicious PyPI Package Exploits Deezer API for Coordinated Music Piracy
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
@simpli/resource-collection
Advanced tools
A data-structure library to work with ResourceCollections (with Id and Tag) and PageCollections (search and order as well)
A data-structure library to work with ResourceCollections (with Id and Tag) and PageCollections (search and order as well)
npm i @simpli/resource-collection class-transformer
ResourceCollection is a Collection of items containing id and tag
import { ResourceCollection, IResource } from '@simpli/resource-collection'
Your class must implement IResource
with $id
and $tag
props or getters
class MyResource implements IResource {
get $id (){ return this.myId }
get $tag () { return this.myName }
myId: number
myName: string
constructor(myId: number, myName: string) {
this.myId = myId
this.myName = myName
}
}
You must informing the resource type and optionally an array of items
const subject = new ResourceCollection(MyResource, [
new MyResource(1, 'first'),
new MyResource(2, 'second'),
new MyResource(3, 'third'),
new MyResource(4, 'fourth')
])
subject.size() // returns 4
subject.isEmpty() // returns false
subject.first().$tag // returns 'first'
subject.last().$tag // returns 'fourth'
const allWPH = subject.allWithPlaceholder('hint')
allWPH[0].$tag // returns 'hint'
const allWPHnull = subject.allWithPlaceholder()
allWPHnull[0] // returns null
subject.getById(2).$tag // returns 'second'
const twoAnd3 = subject.getManyIds([2, 3])
twoAnd3[0].$tag // returns 'second'
twoAnd3[1].$tag // returns 'third'
const thebad = new MyResource(666, 'from hell')
const thegood = new MyResource(777, 'mighty one')
subject.add(thebad) // adds in the last position
subject.add(thegood, 0) // adds in the first position
subject.remove(thebad) // thebad was removed
subject.removeById(3) // removed item with id 3
subject.remove(new MyResource(123212, 'unexistent entry')) // not found, no item was removed
subject.removeById(123212) // not found, no item was removed
subject.clear() // remove all items
subject.addFilter({ abc: 123, ignoreMe: null, you: 'and me' })
subject.params // ignores null fields and returns { abc: 123, you: 'and me' }
subject.clearFilters() // remove all fields from filter
PageCollection is useful to work with paginated collections, it extends ResourceCollection
import { PageCollection, IResource } from '@simpli/resource-collection'
Differently of ResourceCollection, you must create a class for your collection
class MyPageCollection extends PageCollection<MyResource> {
constructor() {
super(MyResource)
}
async queryAsPage() {
// implement how to update itself with API calls
// send the PageCollection properties to the server
// { search, currentPage, perPage, orderBy, asc }
// and set other PageCollection properties on response
// this.total = <the amount of all items, as if it wasn't paginated>
// this.items = <the items on the current page>
// the class is prepared to be used with class-transformer library
// example using @simpli/serialized-request:
return await Request.get(`/mything`, {params: this.params})
.as(this)
.getResponse()
}
}
const subject = new MyPageCollection()
subject.setPerPage(20)
subject.lastPage
// if total is 90 the lastPage will be 4. It contains 5 pages, the last page with only 10 items
subject.setCurrentPage(4)
subject.isLastPage // returns true
await subject.queryCurrentPage(3)
// will change the page securely and call queryAsPage
await subject.queryPrevPage()
// will change the page securely and call queryAsPage
await subject.queryNextPage()
// will change the page securely and call queryAsPage
subject.noPagination() // to remove pagination properties
subject.currentPage // returns null
subject.perPage // returns null
await subject.querySearch()
// will call queryAsPage IF the search prop null, empty
// or >= PageCollection.defaultMinCharToSearch, which is 2 by default
// to avoid searching with insufficient caracters
await subject.queryOrderBy('column')
//sets the orderBy field, toggle asc and call queryAsPage
subject.orderBy // returns 'column'
subject.asc // returns true
await subject.queryOrderBy('column')
subject.asc // returns false
FAQs
A data-structure library to work with ResourceCollections (with Id and Tag) and PageCollections (search and order as well)
We found that @simpli/resource-collection demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.
Security News
Newly introduced telemetry in devenv 1.4 sparked a backlash over privacy concerns, leading to the removal of its AI-powered feature after strong community pushback.