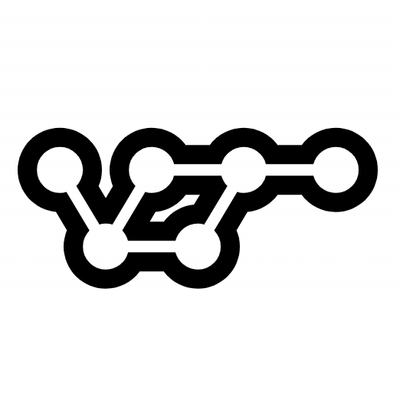
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@snowplow/react-native-tracker
Advanced tools
A library for tracking Snowplow events in React Native
This project is currently in alpha status.
Feedback and contributions are welcome - if you have identified a bug, please log an issue on this repo. For all other feedback, discussion or questions please open a thread on our discourse forum.
Due to pending design decisions, breaking changes in how the methods are called may be introduced before a beta/v1 release.
From the root of your react-js project:
$ npm install @snowplow/react-native-tracker --save
The tracker will be imported as a NativeModule. Initialise it then call the relevant tracking method:
import Tracker from '@snowplow/react-native-tracker';
Tracker.initialize('test-endpoint', 'post', 'https', 'namespace', 'my-app-id', {
setPlatformContext: true,
setBase64Encoded: true,
setApplicationContext:true,
setLifecycleEvents: true,
setScreenContext: true,
setSessionContext: true,
foregroundTimeout: 600, // 10 minutes
backgroundTimeout: 300, // 5 minutes
checkInterval: 15,
setInstallEvent: true
});
Tracker.trackSelfDescribingEvent({'schema': 'iglu:com.acme/hello_world_event/jsonschema/1-0-0', 'data': {'message': 'hello world'}}, []);
cd ios && pod install && cd ..
Run the app with: react-native run-ios
from the root of the project.
react-native run-android
from the root of the project.
initialize('endpoint', // required, collector endpoint, string
'method', // required, http method, string enum ('get' or 'post')
'protocol', // required, http protocol, string enum ('http' or 'https')
'namespace', // required, tracker namespace, string
'my-app-id', // required, app id, string
{
// optional arguments passed as json. Defaults are values provided
autoScreenView: false,
setPlatformContext: false,
setBase64Encoded: false,
setApplicationContext: false,
setLifecycleEvents: false,
setScreenContext: false,
setSessionContext: false,
foregroundTimeout: 600, // 10 minutes
backgroundTimeout: 300, // 5 minutes
checkInterval: 15,
setInstallEvent: false
});
Setting custom subject data is optional, can be called any time, and can be called again to update the subject. Once set, the specified parameters are set for all subsequent events. (For example, a userid may be set after login, and once set all subsequent events will contain the userid).
setSubjectData({ // All parameters optional
userId: 'my-userId', // user id, string
screenWidth: 123, // screen width, integer
screenHeight: 456, // screen height, integer
colorDepth: 20, // colour depth, integer
timezone: 'Europe/London', // timezone, string enum
language: 'en', // language, string enum
ipAddress: '123.45.67.89', // IP address, string
useragent: '[some-user-agent-string]', // user agent, string
networkUserId: '5d79770b-015b-4af8-8c91-b2ed6faf4b1e', // network user id, UUID4 string
domainUserId: '5d79770b-015b-4af8-8c91-b2ed6faf4b1e',// domain user id, UUID4 string
viewportWidth: 123, // viewport width, integer
viewportHeight: 456 // viewport height, integer
});
trackSelfDescribingEvent({ // Self-describing JSON for the custom event
'schema': 'iglu:com.acme/event/jsonschema/1-0-0',
'data': {'message': 'hello world'}
},
[]); // array of self-describing JSONs for custom contexts, or an empty array if none are to be attached
trackStructuredEvent('my-category', // required, category, string
'my-action', // required, action, string
'my-label', // optional - set to null if not needed, label, string
'my-property', // optional - set to null if not needed, property, string
50.00, // optional - set to null if not needed, value, number
[]); // array of self-describing JSONs for custom contexts, or an empty array if none are to be attached
Note - for the track Screen View method, if previous screen values aren't set, they should be automatically set by the tracker.
trackScreenViewEvent('my-screen-name', // required, screen name, string
'63ddebea-a948-4e0c-b458-96467d46f230', // optional - set to null if not needed (recommended), screen id, /uuid4 string
'my-screen-type' // optional - set to null if not needed (recommended), screen type, string
'my-previous-screen', // optional - set to null if not needed (recommended), previous screen name, string
'my-previous-screen-type', // optional - set to null if not needed (recommended), previous screen type, string
'e4711a72-c721-4dfa-b51a-a2e201dcec09', // optional - set to null if not needed (recommended), previous screen id, UUID4 string
'my-transition-type', // optional - set to null if not needed (recommended), transition type, string
[]); // array of self-describing JSONs for custom contexts, or an empty array if none are to be attached
trackPageViewEvent('my-page-url.com', // required, page url, string
'my page title', // optional - set to null if not needed, page title, string
'my-page-referrer.com', // optional - set to null if not needed, referrer url, string
[]); // array of self-describing JSONs for custom contexts, or an empty array if none are to be attached
FAQs
React Native tracker for Snowplow
The npm package @snowplow/react-native-tracker receives a total of 10,261 weekly downloads. As such, @snowplow/react-native-tracker popularity was classified as popular.
We found that @snowplow/react-native-tracker demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.