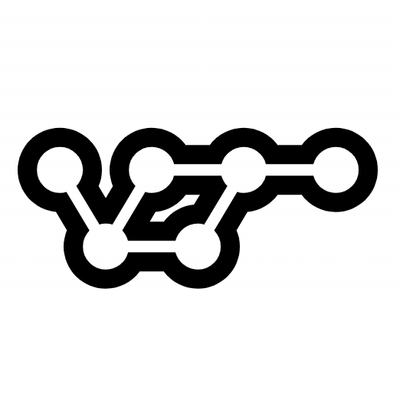
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@stacks/storage
Advanced tools
Store and fetch files with Gaia, the decentralized storage system.
npm install @stacks/storage
import { UserSession } from '@stacks/auth';
import { Storage } from '@stacks/storage';
const appConfig = new AppConfig();
const userSession = new UserSession({ appConfig });
const storage = new Storage({ userSession });
Note that you can also use an existing userSession
object created during the authentication process.
const myData = JSON.stringify({
hello: "world",
num: 1
});
storage.putFile('my_data.json', myData));
Store data at a different path
storage.putFile('path/my_data.json', myData));
Put file with options
const putFileOptions = {
// override the default content type
contentType: 'application/json',
// override encrypting data by default
// you can also set encrypt to a private key to specify a custom encryption key
encrypt: false,
// ignore automatic conflict prevention using etags
dangerouslyIgnoreEtag: true
}
storage.putFile('my_data.json', myData, putFileOptions));
storage.getFile('my_data.json').then((fileContent) => {
console.log(fileContent);
});
Get file with options
const getFileOptions = {
decrypt: false,
// by default files stored are signed and can be verified for authenticity
verify: false
}
storage.getFile('my_data.json', getFileOptions).then((fileContent) => {
console.log(fileContent);
});
storage.deleteFile('my_data.json');
Delete the file and the corresponding signature file if signed
storage.deleteFile('my_data.json', { wasSigned: true });
List all files in the user's Gaia hub
storage.listFiles((filename) => {
if (filename === 'my_data.json') {
return storage.getFile(filename).then((fileContent) => {
console.log(fileContent);
// return false to stop iterating through files
return false;
})
} else {
// return true to continue iterating
return true;
}
});
FAQs
Stacks storage library
The npm package @stacks/storage receives a total of 8,398 weekly downloads. As such, @stacks/storage popularity was classified as popular.
We found that @stacks/storage demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.