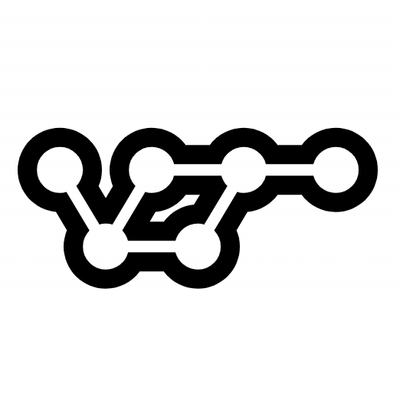
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@tokenex/tokenex-iframe
Advanced tools
The TokenEx Iframe repo is an open source library used for loading and interacting with the hosted [TokenEx Iframe](https://www.tokenex.com/hosted-iframe/)
The TokenEx Iframe repo is an open source library used for loading and interacting with the hosted TokenEx Iframe
Install the iframe npm library
npm install tokenex-iframe
Import the library into your front end application
import { TokenizeIframe } from "tokenex-iframe";
Configure the iframe
TokenizeIframe.configure({
auth: {
tokenExId: "TOKENEX_ID_GOES_HERE",
origin: window.location.origin,
tokenScheme: "TOKEN_SCHEME_GOES_HERE",
// Alternatively there is an optional clientSecret property that generates
// the hmac, but we recommend against exposing your clientSecret publicly
hmac: "BASE64_ENCODED_HMACSHA256_GOES_HERE",
timestamp: "UTC_TIMESTAMP_OF_GENERATED_HMAC_GOES_HERE_YYYYMMDDHHMMSS",
},
// "test" | "production" | "eu"
env: "test",
initialSettings: {
container: "ID_OF_THE_HTML_CONTAINER_TO_APPEND_IFRAME",
},
});
Load the iframe
TokenizeIframe.load();
Before you can use the iframe you must set the configuration object.
TokenizeIframe.configure({
auth: {
tokenExId: "TOKENEX_ID_GOES_HERE",
origin: window.location.origin,
tokenScheme: "TOKEN_SCHEME_GOES_HERE",
// Alternatively there is an optional clientSecret property that generates
// the hmac, but we recommend against exposing your clientSecret publicly
hmac: "BASE64_ENCODED_HMACSHA256_GOES_HERE",
timestamp: "UTC_TIMESTAMP_OF_GENERATED_HMAC_GOES_HERE_YYYYMMDDHHMMSS",
},
// "test" | "production" | "eu"
env: "test",
initialSettings: {
container: "ID_OF_THE_HTML_CONTAINER_TO_APPEND_IFRAME",
},
});
Authentication properties that must be set with a valid hmac
and timestamp
OR with a valid clientSecret
NOTE: It is recommended that your hmac
is generated in a secure backend environment and your clientSecret
isn't exposed to the front end.
auth
/** Valid TokenExID from the Customer Portal */
tokenExId: string;
/** Fully qualified origin for the domain the code is running on */
origin: string;
/** Either the name (case insensitive) of the Token Scheme value */
tokenScheme: number | string;
hmac
authenticationFor more information on generating an HMAC, see: https://docs.tokenex.com/docs/generating-the-authentication-key
/** Base64 encoded HMACSHA256(TokenExID|origin|timestamp|tokenScheme, clientSecret)*/
hmac: string;
/** The UTC timestamp of when the HMACSHA256 has was generated in yyyyMMddHHmmss format */
timestamp: string;
clientSecret
authenticationNOTE: It is recommended that you utilize an hmac that is generated in a secure backend environment and not your clientSecret so that it isn't exposed to the front end.
/** Client Secret found in the Portal */
clientSecret: string;
TokenEx environment from which to load the iframe
NOTE: This can be extended for local internal testing through a .env
file with values for VITE_APP_ENVIRONMENTS_LOCAL
, VITE_APP_ENVIRONMENTS_DEV
, and VITE_APP_ENVIRONMENTS_STAGING
/** TokenEx environment to load iframe
* @property test https://test-htp.tokenex.com
* @property production https://htp.tokenex.com
* @property eu https://eu1-htp.tokenex.com
*/
env: "test" | "production" | "eu";
These are settings that are sent in the GET
request to initialize the iframe.
/** If no object is provided for this field, the max length for any card brand will be set to the default max length, which is 19. */
cardMaxLengths?: CardMaxLengths;
/** Required - The div id in which you want to render the iFrame */
container: string;
/** Allows customization of the iframe data label. If none supplied, this defaults to "Data" */
customDataLabel?: string;
/** Allows customization of the CVV iframe data label. If none supplied, this defaults to "CVV" */
customCvvDataLabel?: string;
/** Specifies if a CVV iFrame should be rendered; defaults to false */
cvv?: boolean;
/** The div id in which you want to render the CVV iFrame when cvvOnly is false */
cvvContainer?: string;
/** Specifies if only the CVV iFrame should be rendered; defaults to false */
cvvOnly?: boolean;
/** Content of the title element used by the cvv iframe document */
cvvTitle?: string;
/** Indicates the system to enforce Luhn compliance */
enforceLuhnCompliance?: boolean;
/** The expiration time when the authentication key is going to expire after initial load.
* Valid range: 1 to 1200 Seconds NOTE: Default expiration time is set to 1200 seconds (20 Minutes).
* The expiration time will be reset to default, in case if user provides value which • Is less than or equal to 0 • Is greater than 1200 */
expiresInSeconds?: number;
/** Inlines the JavaScript on the Iframe, eliminating a network call */
inlineIframeJavaScript?: boolean;
/** Sets the maxLength property of the input. NOTE: This parameter only works with input type "text". */
inputMaxLength?: number;
/** Merchant Id for use with Kount Integration (https://docs.tokenex.com/reference/third-party-integrations) */
kountMerchantId?: string;
/** Specifies if the PCI version of the iFrame should be rendered; defaults to false */
pci?: boolean;
/** If true, then this will return nameOnCard and cardExp values whenever a
* user utilizes the browsers autocomplete functionality. NOTE: Autocomplete must be enabled */
returnAutoCompleteValues?: boolean;
/** True: returns the kHash value for a given credit card False: returns kHash property with empty value
* (https://docs.tokenex.com/reference/third-party-integrations) */
returnKHash?: boolean;
/** For use with Womply */
returnWHash?: boolean;
/** For use with 3DS (https://docs.tokenex.com/reference/third-party-integrations) */
threeDSMethodNotificationUrl?: string;
/** Content of the title element used by the iframe document */
title?: string;
/** If cvvOnly is set to true, the token the CVV is associated with must be provided */
token?: string;
/** For use with 3DS (https://docs.tokenex.com/reference/third-party-integrations) */
use3DS?: boolean;
/** If true, returns the first eight characters of the PAN in the Tokenize response.
* NOTE: Will not return firstEight in the Tokenize response if: • PCI is false • or PAN is fewer than 16 characters. */
useExtendedBin?: boolean;
/** For use with Kount Integration (https://docs.tokenex.com/reference/third-party-integrations) */
useKount?: boolean;
These are settings that are sent via postMessage
after the iframe is rendered.
/** If true, then unknown, or types other than 'masterCard', 'americanExpress',
* 'discover', 'visa', 'diners', or 'jcb' will tokenize; defaults to false
* NOTE: If this field is true while using Custom Data Types in PCI w/ CVV or CVV Only modes,
* the CVV text field's allowable input will be 4. Use the cvvValidRegex field
* to validate the length of the CVV input.
*/
allowUnknownCardTypes?: boolean;
/** If cvvOnly is set to true, a card type must be provided to validate the CVV length */
cardType?: string;
/** Custom validations appended to our standard brand detection (https://docs.tokenex.com/reference/using-custom-data-types) */
customDataTypes?: CustomDataType[];
/** If present, the RegEx expression provided will be used during validation events
* in addition to any internal validation. Please note that the
* backslash is an escape character - if used in your RegEx, it will need to be escaped */
customRegEx?: string;
/** Allowed values are "number", "tel", and "text"; if nothing is supplied, this will default to "text" */
cvvInputType?: "number" | "tel" | "text";
/** Optionally sets the placeholder attribute of the CVV input when cvvOnly is false */
cvvPlaceholder?: string;
/** If enabled, data will be output to the console to assist with debugging */
debug?: boolean;
/** If true, sets the aria-required and aria-invalid attributes to true.
* When Validate function is called aria-invalid will update according to the result of isValid */
enableAriaRequired?: boolean;
/** This will set the data element autocomplete attribute to: cc-number and the cvv field to cc-csc.
* For use with cvv only mode, the only data element is cc-cs https://docs.tokenex.com/docs/autocomplete */
enableAutoComplete?: boolean;
/** If pci is true, then enabling this property will auto format the credit card number as it appears on the physical card */
enablePrettyFormat?: boolean;
/** If true, then the Validate function will be called when the input loses focus; otherwise, Validate must be invoked manually
* NOTE: This functionality is only available for PCI and PCIwithCVV iFrame modes */
enableValidateOnBlur?: boolean;
/** If true, then the Validate function will be called after each keystroke in the CVV iframe; otherwise, Validate must be invoked manually
* NOTE: This functionality is only available for PCIwithCVV iFrame modes */
enableValidateOnCvvKeyUp?: boolean;
/** If true, then the Validate function will be called after each keystroke; otherwise, Validate must be invoked manually
* NOTE: This functionality is only available for PCI and PCIwithCVV iFrame modes */
enableValidateOnKeyUp?: boolean;
/** A google font to use in the iframe */
font?: string;
/** Settings for use with a Forter Integration (https://docs.tokenex.com/reference/third-party-integrations) */
forterSiteId?: string;
/** Settings for use with a Forter Integration (https://docs.tokenex.com/reference/third-party-integrations) */
forterUsername?: string;
/** Mode to be set on input field (https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/inputmode) */
inputMode?: string;
/** Allowed values are "number", "tel", and "text". If nothing is supplied, this will default to "text" */
inputType?: "number" | "tel" | "text";
/** Hides the input being typed by masking it with masking character */
maskInput?: boolean;
/** Optionally sets the placeholder attribute of the input */
placeholder?: string;
/** CSS to be applied to the PAN inputs (https://docs.tokenex.com/reference/styling-the-iframe) */
styles?: {
/** The base style applied to the input element within the iFrame */
base?: string;
/** Optional style applied when input element within the iFrame gains focus */
focus?: string;
/** Style applied to the input element if a validation failure occurs */
error?: string;
/** Allows for the styling of the input placeholder text */
placeholder?: string;
/** CSS to be applied to the CVV inputs (https://docs.tokenex.com/reference/styling-the-iframe) */
cvv?: {
/** The base style applied to the input element within the iFrame */
base?: string;
/** Optional style applied when input element within the iFrame gains focus */
focus?: string;
/** Style applied to the input element if a validation failure occurs */
error?: string;
/** Allows for the styling of the input placeholder text */
placeholder?: string;
}
};
configure(configuration)
Sets the configuration object settings for the iframe see the configuration section for more details.
TokenizeIframe.configure({
auth: {
tokenExId: "TOKENEX_ID_GOES_HERE",
origin: window.location.origin,
tokenScheme: "TOKEN_SCHEME_GOES_HERE",
// Alternatively there is an optional clientSecret property that generates
// the hmac, but we recommend against exposing your clientSecret publicly
hmac: "BASE64_ENCODED_HMACSHA256_GOES_HERE",
timestamp: "UTC_TIMESTAMP_OF_GENERATED_HMAC_GOES_HERE_YYYYMMDDHHMMSS",
},
// "test" | "production" | "eu"
env: "test",
initialSettings: {
container: "ID_OF_THE_HTML_CONTAINER_TO_APPEND_IFRAME",
},
});
validateConfig()
Validates the current configuration of the iframe and returns { isValid: boolean, details: string[] }
which can be useful for testing and troubleshooting your configuration object. This method is also ran on TokenizeIframe.load()
so the iframe won't load if the configuration is invalid.
const { isValid, details } = TokenizeIframe.validateConfig();
if (!isValid) {
console.log(details);
}
onEvent(messageEvent, callback)
Sets a listener that returns a callback whenever an event is triggered. See events section for more details.
TokenizeIframe.onEvent("load", () => {
// iframe successfully loaded
hideLoadingSpinner();
});
load()
Loads the iframe and appends the iframe to the container element provided in the configuration
TokenizeIframe.load();
remove()
Removes the iframe from the container element provided in the configuration
TokenizeIframe.remove();
setFraudServicesRequestDetails(data)
Sets the fraud services request with the specified details
TokenizeIframe.setFraudServicesRequestDetails(data);
tokenize()
Validates and tokenizes the contents of the iFrame (if validations succeed)
TokenizeIframe.tokenize();
validate()
Invokes validation routines on the contents of the iFrame
TokenizeIframe.validate();
reset()
Restore iFrame contents to initial state
TokenizeIframe.reset();
blur()
Remove focus from the input element within the iFrame
TokenizeIframe.blur();
cvvBlur()
Remove focus from the CVV input element within the iFrame
TokenizeIframe.cvvBlur();
focus()
Set the focus of the page to the input element within the iFrame
TokenizeIframe.focus();
cvvFocus()
Set the focus of the page to the CVV input element within the iFrame
TokenizeIframe.cvvFocus();
toggleMask()
Toggles the masking of the input element within the iframe
TokenizeIframe.toggleMask();
toggleCvvMask()
Toggles the masking of the cvv input element within the iframe
TokenizeIframe.toggleCvvMask();
binLookup()
Send a Bin Lookup request to the Iframe (subscribers only)
TokenizeIframe.toggleCvvMask();
sendCommandToIframe(command, data)
(Advanced use cases) Sends a post message command to the iframe
TokenizeIframe.sendCommandToIframe(command, data);
Each event object returned from the iFrame will contain properties relevant to the type of message or event. Wiring up one or more of these events to your instance of the iFrame is done on the iFrame object generated by the call to the on() function and supplying a callback function.
Event | Raise Condition(s) |
---|---|
load | The iFrame has loaded. |
focus | The input in the iFrame gains focus. |
blur | The input in the iFrame loses focus. |
change | The input value has changed. Raised only if the data has changed between the most recent focus and blur events. |
cardTypeChange | (PCI only) The possible card type entered by the user has changed. |
error | An error occurred during the invocation of a command. |
validate | The validate command was invoked, either directly by the user or as part of the Tokenize command (see Validate event fields below). |
tokenize | The tokenize command was invoked (see Tokenize event fields below). |
cvvFocus | The input in the cvv iFrame gains focus. |
cvvBlur | The input in the cvv iFrame loses focus. |
notice | Raised when the iFrame is loaded by providing an expiration date time stamp as yyyyMMddHHmmss in UTC (current time + 20 mins) and a second notice one minute prior to expiration." |
expired | Raised when the IFrame has expired. |
toggleMask | The toggleMask command was invoked (see ToggleMask and ToggleCvvMask event fields below) |
toggleCvvMask | The toggleCvvMask command was invoked (see ToggleMask and ToggleCvvMask event fields below) |
binLookupResponse | The Bin Lookup command has returned a response |
TokenizeIframe.onEvent("load", () => { console.log("CC iFrame Loaded") });
TokenizeIframe.onEvent("focus", () => { console.log("CC iFrame focus") });
TokenizeIframe.onEvent("blur", () => { console.log("CC iFrame blur") });
TokenizeIframe.onEvent("change", () => { console.log("CC iFrame Change:") });
TokenizeIframe.onEvent("validate", (data) => { console.log("CC iFrame validate:" + JSON.stringify(data)) });
TokenizeIframe.onEvent("cardTypeChange", (data) => { console.log("CC iFrame cardTypeChange:" + JSON.stringify(data)) });
TokenizeIframe.onEvent("tokenize", (data) => { console.log("CC iFrame Tokenize:" + JSON.stringify(data)) });
TokenizeIframe.onEvent("error", (data) => { console.log("CC iFrame error:" + JSON.stringify(data)) });
TokenizeIframe.onEvent("cvvFocus", () => { console.log("CVV iFrame focus") });
TokenizeIframe.onEvent("cvvBlur", () => { console.log("CVV iFrame blur") });
TokenizeIframe.onEvent("autoCompleteValues", (data) => { console.log("CC iFrame Autocomplete Values:" + JSON.stringify(data)) });
TokenizeIframe.onEvent(“notice”, function(data) { console.log(“CC iFrame notice:” + JSON.stringify(data) });
MIT License
Copyright (c) TokenEx
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
FAQs
The TokenEx Iframe repo is an open source library used for loading and interacting with the hosted [TokenEx Iframe](https://www.tokenex.com/hosted-iframe/)
The npm package @tokenex/tokenex-iframe receives a total of 70,695 weekly downloads. As such, @tokenex/tokenex-iframe popularity was classified as popular.
We found that @tokenex/tokenex-iframe demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.