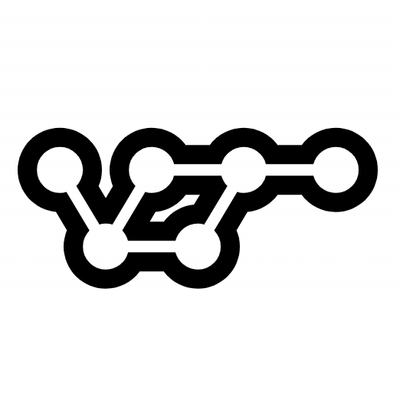
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@wordpress/interactivity
Advanced tools
Package that provides a standard and simple way to handle the frontend interactivity of Gutenberg blocks.
The Interactivity API, introduced in WordPress 6.5, provides a standard way for developers to add interactions to the front end of their blocks. The API is also used in many Core WordPress blocks, including Search, Query, Navigation, and File.
This standard makes it easier for developers to create rich, interactive user experiences, from simple counters or pop-ups to more complex features like instant page navigation, instant search, shopping carts, or checkouts.
Blocks can share data, actions, and callbacks between them. This makes communication between blocks simpler and less error-prone. For example, clicking on an “add to cart” block can seamlessly update a separate “cart” block.
For more information about the genesis of the Interactivity API, check out the original proposal. You can also review the merge announcement, the status update post, and the official Trac ticket.
Install the Interactivity API using the command:
npm install @wordpress/interactivity --save
This step is only required if you use the Interactivity API outside WordPress.
Within WordPress, the package is already bundled in Core. To ensure it's loaded, add @wordpress/interactivity
to the dependency array of the script module. This process is often done automatically with tools like wp-scripts
.
Furthermore, this package assumes your code will run in an ES2015+ environment. If you're using an environment with limited or no support for such language features and APIs, you should include the polyfill shipped in @wordpress/babel-preset-default
in your code.
This guide will help you build a basic block that demonstrates the Interactivity API in WordPress.
Start by ensuring you have Node.js and npm
installed on your computer. Review the Node.js development environment guide if not.
Next, use the @wordpress/create-block
package and the @wordpress/create-block-interactive-template
template to scaffold the complete “My First Interactive Block” plugin.
Choose the folder where you want to create the plugin, and then execute the following command in the terminal from within that folder:
npx @wordpress/create-block@latest my-first-interactive-block --template @wordpress/create-block-interactive-template
The slug provided (my-first-interactive-block
) defines the folder name for the scaffolded plugin and the internal block name.
With the plugin activated, you can explore how the block works. Use the following command to move into the newly created plugin folder and start the development process.
cd my-first-interactive-block && npm start
When create-block
scaffolds the block, it installs wp-scripts
and adds the most common scripts to the block’s package.json
file. Refer to the Get started with wp-scripts article for an introduction to this package.
The npm start
command will start a development server and watch for changes in the block’s code, rebuilding the block whenever modifications are made.
When you are finished making changes, run the npm run build
command. This optimizes the block code and makes it production-ready.
If you have a local WordPress installation already running, you can launch the commands above inside the plugins
folder of that installation. If not, you can use wp-now
to launch a WordPress site with the plugin installed by executing the following command from the plugin's folder (my-first-interactive-block
).
npx @wp-now/wp-now start
You should be able to insert the "My First Interactive Block" block into any post and see how it behaves in the front end when published.
Interactivity API is included in Core in WordPress 6.5. For versions below, you'll need Gutenberg 17.5 or higher installed and activated in your WordPress installation.
It’s also important to highlight that the block creation workflow doesn’t change, and all the prerequisites remain the same. These include:
You can start creating interactions once you set up a block development environment and are running WordPress 6.5+ (or Gutenberg 17.5+).
interactivity
support to block.json
To indicate that the block supports the Interactivity API features, add "interactivity": true
to the supports
attribute of the block's block.json
file.
"supports": {
"interactivity": true
},
wp-interactive
directive to a DOM elementTo "activate" the Interactivity API in a DOM element (and its children), add the wp-interactive
directive to the element in the block's render.php
or save.js
files.
<div data-wp-interactive="myPlugin">
<!-- Interactivity API zone -->
</div>
To take a deep dive into how the API works internally, the list of Directives, and how Store works, visit the API reference.
Here you have some more resources to learn/read more about the Interactivity API:
There's a Tracking Issue opened to ease the coordination of the work related to the Interactivity API Docs: Documentation for the Interactivity API - Tracking Issue #53296
Interactivity API proposal, as part of Gutenberg and the WordPress project is free software, and is released under the terms of the GNU General Public License version 2 or (at your option) any later version. See LICENSE.md for complete license.
FAQs
Package that provides a standard and simple way to handle the frontend interactivity of Gutenberg blocks.
The npm package @wordpress/interactivity receives a total of 11,396 weekly downloads. As such, @wordpress/interactivity popularity was classified as popular.
We found that @wordpress/interactivity demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.