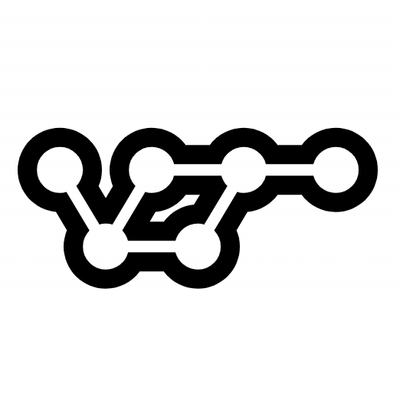
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
agent-protocol
Advanced tools
This SDK implements the Agent Communication Protocol in JavaScript/TypeScript and allows you to easily wrap your agent in a webserver compatible with the protocol - you only need to define an agent task handler.
npm install agent-protocol
Then add the following code to your agent:
import Agent, {
type StepHandler,
type StepInput,
type StepResult,
type TaskInput,
} from "agent-protocol";
async function taskHandler(taskInput: TaskInput | null): Promise<StepHandler> {
console.log(`task: ${taskInput}`);
async function stepHandler(stepInput: StepInput | null): Promise<StepResult> {
console.log(`step: ${stepInput}`);
return {
output: stepInput,
};
}
return stepHandler;
}
Agent.handleTask(taskHandler).start();
import Agent from "agent-protocol";
async function taskHandler(taskInput) {
console.log(`task: ${taskInput}`);
async function stepHandler(stepInput) {
console.log(`step: ${stepInput}`);
return {
output: stepInput,
};
}
return stepHandler;
}
Agent.handleTask(taskHandler).start();
To start the server run the file where you added the code above:
# Typescript
$ ts-node file/where/you/added/code.ts
# Javascript
$ node file/where/you/added/code.ts
and then you can call the API using the following terminal commands:
To create a task run:
curl --request POST \
--url http://localhost:8000/agent/tasks \
--header 'Content-Type: application/json' \
--data '{
"input": "task-input-to-your-agent"
}'
You will get a response like this:
{
"input": "task-input-to-your-agent",
"task_id": "e6d768bb-4c50-4007-9853-aeffb46c77be",
"artifacts": []
}
Then to execute one step of the task copy the task_id
you got from the
previous request and run:
curl --request POST \
--url http://localhost:8000/agent/tasks/<task-id>/steps
To get a response like this:
{
"output": "output-from-the-agent",
"artifacts": [],
"is_last": false,
"input": null,
"task_id": "e6d768bb-4c50-4007-9853-aeffb46c77be",
"step_id": "8ff8ba39-2c3e-4246-8086-fbd2a897240b"
}
FAQs
API for interacting with Agent
We found that agent-protocol demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.