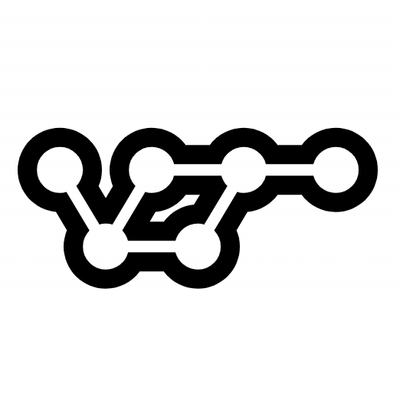
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
amazon-buddy
Advanced tools
Amazon Scraper. You can scrape products from amazon search result and you can also scrape reviews from a specific product
Useful tool to scrape product information from amazon
Product List
Review List
Note:
Possible errors
Install from NPM
$ npm i -g amazon-buddy
Install from YARN
$ yarn global add amazon-buddy
Terminal
$ amazon-buddy --help
Usage: amazon-buddy <command> [options]
Commands:
amazon-buddy products scrape products from the provided key word
amazon-buddy reviews scrape reviews from a product
Options:
--help, -h help [boolean]
--version Show version number [boolean]
--keyword, -k Amazon search keyword ex. 'Xbox one' [string] [default: ""]
--asin, -a To scrape reviews you need to provide product ASIN(amazon
product id) [string] [default: ""]
--number, -n Number of products to scrape. Maximum 100 products or 300 reviews [default: 10]
--save, -s Save to a CSV file? [boolean] [default: true]
--sort If searching for a products then list will be sorted by a higher
score(reviews*rating). If searching for a reviews then they will
be sorted by rating. [boolean] [default: false]
--discount, -d Scrape only products with the discount
[boolean] [default: false]
--sponsored Scrape only sponsored products [boolean] [default: false]
--min-rating Minimum allowed rating [default: 1]
--max-rating Maximum allowed rating [default: 5]
--host, -H The custom amazon host (can be www.amazon.fr, www.amazon.de, etc.)
[string] [default: "www.amazon.com"]
Examples:
amazon-buddy products -k 'Xbox one'
amazon-buddy products -k 'Xbox one' -h 'www.amazon.fr'
amazon-buddy reviews -a B01GW3H3U8
Example 1
Scrape 40 producs from the "vacume cleaner" keyword and save everything to a CSV file
$ amazon-buddy products -k 'vacume cleaner' -n 40
The file will be saved in a folder from which you run the script: 1552945544582_products.csv
Example 2
Scrape 100 reviews from a product by using ASIN. NOTE: ASIN is a uniq amazon product ID, it can be found in product URL or if you have scraped product list with our tool you will find it in a CSV file
$ amazon-buddy reviews -a B01GW3H3U8 -n 100
The file will be saved in a folder from which you run the script: 1552945544582_B01GW3H3U8_reviews.csv
Example 3
Scrape 300 producs from the "xbox one" keyword with rating minimum rating 3 and maximum rating 4 and save everything to a CSV file
$ amazon-buddy products -k 'xbox one' -n 300 --min-rating 3 --max-rating 4
The file will be saved in a folder from which you run the script: 1552945544582_products.csv
const amazonScraper = require('amazon-buddy');
(async() => {
try{
// Collect 50 products from a keyword 'xbox one'
let products = await amazonScraper.products({keyword: 'Xbox One', number: 50, save: true });
// Collect 50 reviews from a product ID B01GW3H3U8
let reviews = await amazonScraper.rewviews({asin: 'B01GW3H3U8', number: 50, save: true });
// Collect 50 products from a keyword 'xbox one' with rating between 3-5 stars
let products_rank = await amazonScraper.products({keyword: 'Xbox One', number: 50, rating:[3,5] });
// Collect 50 reviews from a product ID B01GW3H3U8 with rating between 1-2 stars
let reviews_rank = await amazonScraper.rewviews({asin: 'B01GW3H3U8', number: 50, rating: [1,2] });
}catch(error){
console.log(error);
}
})()
const amazonScraper = require('amazon-buddy');
let products = amazonScraper.products({
keyword: 'xbox',
number: 50,
event: true,
});
products.on('error message', error => {
console.log(error);
});
products.on('item', item => {
console.log(item);
});
products.on('completed', () => {
console.log('completed');
});
products._startScraper();
JSON/CSV output(products):
[{
asin: 'B01N6HLV9L',
discounted: false, // is true if product is with the discount
sponsored: false, // is true if product is sponsored
price: '$32.99',
before_discount: '$42.99', // displayed only if price is discounted
title:'product title',
url:'long amazon url'
}...]
JSON/CSV output(reviews):
[{
id: 'R335O5YFEWQUNE',
review_data: '6-Apr-17',
name: 'Bob',
title: 'Happy Gamer',
rating: 5,
review: 'blah blah blah'
}...]
Options
let options = {
//Search keyword: {string default: ""}
keyword: "",
//Number of products to scrape: {int default: 10}
number: 10,
// Enable/disabled EventEmitter: {boolean default: false}
// If enabled then you won't be able to use promises
event: false,
//Save to a CSV file: {boolean default: false}
save: false,
//Set proxy: {string default: ""}
proxy: "",
//Sort by rating. [minRating, maxRating]: {array default: [1,5]}
rating:[1,5],
//Sorting. If searching for a products then list will be sorted by a higher score(number of reviews*rating). If searching for a reviews then they will be sorted by rating.: {boolean default: false}
sort: false,
//Scrape only products with the discount: {boolean default: false}
discount: false,
//Scrape only sponsored products: {boolean default: false}
sponsored: false,
//Search on custom amazon host to list products in specific language
host: "www.amazon.de"
};
MIT
Free Software
FAQs
Amazon Scraper. You can scrape products from amazon search result and you can also scrape reviews from a specific product
The npm package amazon-buddy receives a total of 26 weekly downloads. As such, amazon-buddy popularity was classified as not popular.
We found that amazon-buddy demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.