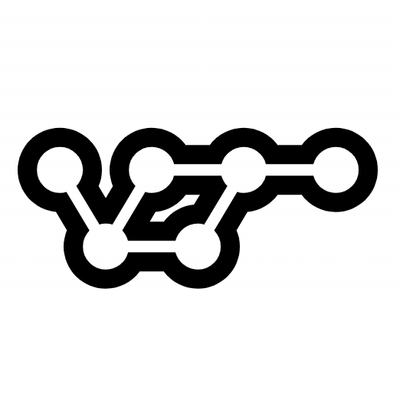
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
barcode-scan-js
Advanced tools
JavaScript browser-based scanner keyboard input library for seamless integration of barcode and QR code scanning into web applications
A JavaScript library for integrating barcode and QR code scanning into web applications using a scanner-like keyboard input. This library provides a customizable element, barcode-scan
, that can be easily integrated into your web projects.
npm install barcode-scan-js
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Barcode Scan Demo</title>
</head>
<body>
<!-- Add the barcode-scan element -->
<barcode-scan></barcode-scan>
<script src="main.js"></script>
</body>
</html>
<barcode-scan>
element in your HTML:<barcode-scan config='{"minLength": 4, "maxLength": 12, "toUpper": true}'></barcode-scan>
// Import the library
import 'barcode-scan-js';
// Get a reference to the barcode-scan element
const barcodeScanner = document.querySelector('barcode-scan');
// Add an event listener to capture scan results
barcodeScanner.addEventListener('scan', (event) => {
const scanResult = event.detail;
// Handle the scan result here
console.log('Scanned Barcode:', scanResult.code);
console.log('Is Valid:', scanResult.isValid);
console.log('Time Taken (ms):', scanResult.timeTakenMs);
console.log('Cleaned Code:', scanResult.cleanedCode);
});
The <barcode-scan>
element supports the following configuration options:
minLength
: Minimum length of the scanned barcode (default: 1).maxLength
: Maximum length of the scanned barcode (default: 14).codeStartsWith
: Start of the barcode string (default: empty string).codeEndsWith
: End of the barcode string (default: empty string).scanTimeoutMs
: Timeout (in milliseconds) to consider the input as a scan (default: 3000ms).ignoreOverElements
: An array of HTML tag names to ignore when scanning (default: ['INPUT']
).toUpper
: Convert scanned text to uppercase (default: false).This project is licensed under the MIT License - see the LICENSE.md file for details.
Feel free to modify and expand this README to suit your project's specific needs.
FAQs
JavaScript browser-based scanner keyboard input library for seamless integration of barcode and QR code scanning into web applications
We found that barcode-scan-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.