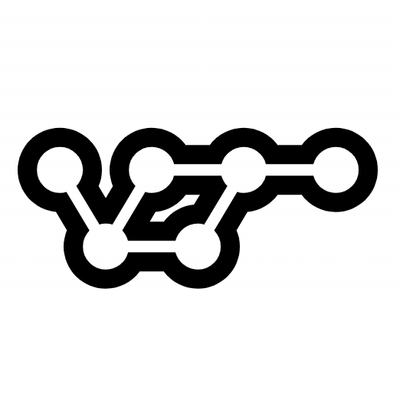
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
cdk-rest-api-integrations
Advanced tools
This CDK constructs library integrates AWS RestApi (a.k.a ApiGateway) with other AWS Services
This CDK constructs library integrates AWS RestApi (a.k.a ApiGateway) with other AWS Services directly without the need for a lambda function.
npm install cdk-rest-api-integrations --save
Add the integration to your stack:
// add the RestApi and Topic to be integrated with each other
const restApi = new apigateway.RestApi(this, 'myRestApi')
const myResource = restApi.root.addResource('myResource');
const topic = new sns.Topic(this, "myTopic")
// add the integration
new SnsRestApiIntegration(this, 'mySnsIntegration', {
topic: topic,
restApiResource: myResource
})
After deploying your CDK stack you can publish messages to the SNS Topic via HTTP:
curl -X POST https://xxxxxxxx.execute-api.eu-central-1.amazonaws.com/prod/myResource \
--header 'Content-Type: application/json' \
--data-raw '{"cdk":"rocks"}'
Add the integration to your stack:
// add the RestApi and Queue to be integrated with each other
const restApi = new apigateway.RestApi(this, 'myRestApi')
const myResource = restApi.root.addResource('myResource');
const queue = new sqs.Queue(this, "myQueue")
// add the integration
new SqsRestApiIntegration(this, 'mySqsIntegration', {
queue: queue,
restApiResource: myResource
})
After deploying your CDK stack you can publish messages to the SQS Queue via HTTP:
curl -X POST https://xxxxxxxx.execute-api.eu-central-1.amazonaws.com/prod/myResource \
--header 'Content-Type: application/json' \
--data-raw '{"cdk":"rocks"}'
FAQs
This CDK constructs library integrates AWS RestApi (a.k.a ApiGateway) with other AWS Services
We found that cdk-rest-api-integrations demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.