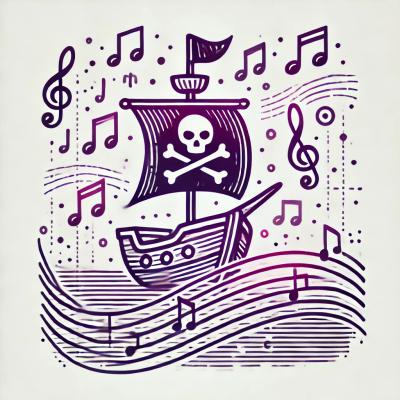
Research
Security News
Malicious PyPI Package Exploits Deezer API for Coordinated Music Piracy
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
component-bind
Advanced tools
The component-bind npm package is a utility for binding functions to a specific context, ensuring that the 'this' keyword inside the function refers to the desired object. This is particularly useful in event handling and callback scenarios where the context can be lost.
Basic Function Binding
This feature allows you to bind a function to a specific context. In this example, the 'getValue' function is bound to the 'obj' object, ensuring that 'this' inside 'getValue' refers to 'obj'.
const bind = require('component-bind');
const obj = {
value: 42,
getValue: function() {
return this.value;
}
};
const boundGetValue = bind(obj, obj.getValue);
console.log(boundGetValue()); // 42
Event Handling
This feature is useful for event handling where the context can be lost. By binding 'handleClick' to 'obj', you ensure that 'this' inside 'handleClick' refers to 'obj' when the button is clicked.
const bind = require('component-bind');
const obj = {
value: 42,
handleClick: function() {
console.log(this.value);
}
};
const button = document.createElement('button');
button.addEventListener('click', bind(obj, obj.handleClick));
document.body.appendChild(button);
Lodash is a popular utility library that provides a 'bind' function similar to component-bind. Lodash's 'bind' function offers more features, such as partial application of arguments, making it more versatile for complex use cases.
Underscore is another utility library that includes a 'bind' function. While it is similar to component-bind, Underscore's 'bind' function is part of a larger suite of functional programming utilities, making it a more comprehensive solution for developers who need additional functionality.
The 'function-bind' package is a polyfill for the native Function.prototype.bind method. It provides similar functionality to component-bind but is designed to work in environments where the native 'bind' method is not available.
Function binding utility.
$ component install component/bind
should bind the function to the given object.
var tobi = { name: 'tobi' };
function name() {
return this.name;
}
var fn = bind(tobi, name);
fn().should.equal('tobi');
should curry the remaining arguments.
function add(a, b) {
return a + b;
}
bind(null, add)(1, 2).should.equal(3);
bind(null, add, 1)(2).should.equal(3);
bind(null, add, 1, 2)().should.equal(3);
should bind the method of the given name.
var tobi = { name: 'tobi' };
tobi.getName = function() {
return this.name;
};
var fn = bind(tobi, 'getName');
fn().should.equal('tobi');
MIT
FAQs
function binding utility
The npm package component-bind receives a total of 1,179,287 weekly downloads. As such, component-bind popularity was classified as popular.
We found that component-bind demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.
Security News
Newly introduced telemetry in devenv 1.4 sparked a backlash over privacy concerns, leading to the removal of its AI-powered feature after strong community pushback.