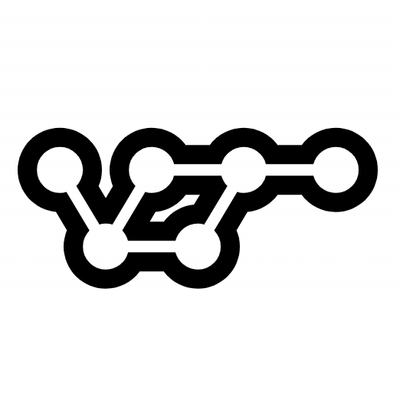
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
craco-alias
Advanced tools
A craco plugin for automatic aliases generation for Webpack and Jest.
:warning: The plugin does not fully support a module alises
Install craco
Install craco-alias
:
npm i -D craco-alias
Edit craco.config.js
:
const CracoAlias = require("craco-alias");
module.exports = {
plugins: [
{
plugin: CracoAlias,
options: {
// craco-alias options
// please see below
}
}
]
};
Go to Examples section
source
:
One of "options"
, "jsconfig"
, "tsconfig"
Defaults to "options"
aliases
:
An object with aliases names and paths
Defaults to {}
tsConfigPath
:
A path to tsconfig file
Only required when source
is set to "tsconfig"
Note: you don't need to add
/*
part for directories in this case
/* craco.config.js */
const CracoAlias = require("craco-alias");
module.exports = {
plugins: [
{
plugin: CracoAlias,
options: {
source: "options",
aliases: {
"@file": "src/file.js",
"@dir": "src/some/dir"
}
}
}
]
};
/* craco.config.js */
const CracoAlias = require("craco-alias");
module.exports = {
plugins: [
{
plugin: CracoAlias,
options: {
source: "jsconfig"
}
}
]
};
Note: your jsconfig should always have baseUrl and paths properties
/* jsconfig.json */
{
compilerOptions: {
baseUrl: "src",
paths: {
"@file": ["file.js"],
"@dir/*": ["dir/*", "dir"]
}
}
}
Go to project's root directory.
Create tsconfig.extend.json
.
Edit it as follows:
{
"compilerOptions": {
"baseUrl": "src",
"paths": {
"@file-alias": ["./your/file.tsx"],
"@folder-alias/*": ["./very/long/path/*", "./very/long/path/"]
}
}
}
Go to tsconfig.json
.
Extend tsconfig.json
from tsconfig.extend.json
:
{
+ "extends": "./tsconfig.extend.json",
"compilerOptions": {
...
},
...
}
Edit craco.config.js
:
const CracoAlias = require("craco-alias");
module.exports = {
plugins: [
{
plugin: CracoAlias,
options: {
source: "tsconfig",
// tsConfigPath should point to the file where "baseUrl" and "paths" are specified
tsConfigPath: "./tsconfig.extend.json"
}
}
]
};
FAQs
A craco plugin for automatic aliases generation
The npm package craco-alias receives a total of 19,473 weekly downloads. As such, craco-alias popularity was classified as popular.
We found that craco-alias demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.