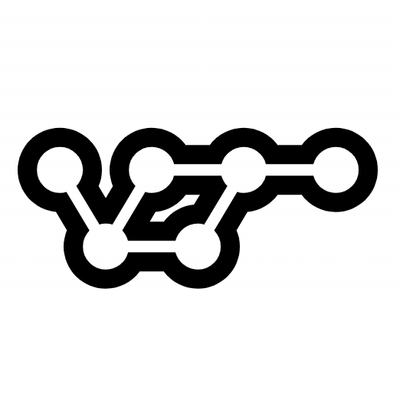
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Enables basic IMAP functionalities in Cypress. The point of the plugin is to make email content testing easy.
npm i -D cymap
Import cymap tasks in cypress.config.js. and put cymapTasks object in on task.
const { defineConfig } = require("cypress");
const cymapTasks = require("cymap/src/cymapTasks")
module.exports = defineConfig({
e2e: {
setupNodeEvents(on, config) {
on("task",{
...cymapTasks
})
// implement node event listeners here
},
},
});
Import cymap index in e2e.js
// Import commands.js using ES2015 syntax:
import './commands'
import "cymap/src/index"
cy.setConnectionConfig( {
password:"socadscjfa",
user:"some.gmail@gmail.com",
host:'imap.gmail.com',
port:993,
tls:true,
tlsOptions: { rejectUnauthorized: false }
})
Needs to be called in before each.
cy.createConnection()
Implicitly called inside the email functions.
cy.destroyConnection()
Implicitly called inside the email functions.
cy.getAllMail()
Fetches all mail from the INBOX
cy.deleteAllMail()
Deletes all mail from the INBOX. For gmail the mail is moved to Waste box
cy.getEmailByIndex(1)
Fetches email by index top down. Top email in the INBOX is under index 1.
cy.deleteEmailByIndex(1)
Deletes email by index top down. Top email in the INBOX is under index 1.
cy.pasteHtml(html)
Pastes HTML to the DOM
Email body example:
{
"attachments": [],
"headers": {},
"headerLines": [...],
"html": "<p>Hello World </p>",
"text": "Hello World",
"textAsHtml": "<p>Hello World</p>",
"subject": "Hello World",
"date": "2024-04-30T16:15:16.000Z",
"to": {
"value": [
{
"address": "some.gmail@gmail.com",
"name": ""
}
],
"html": "<span class=\"mp_address_group\"><a href=\"mailto:some.gmail@gmail.com\" class=\"mp_address_email\">some.gmail@gmail.com.com</a></span>",
"text": "some.gmail@gmail.com"
},
"from": {
"value": [
{
"address": "some.gmail@gmail.com",
"name": ""
}
],
"html": "<span class=\"mp_address_group\"><a href=\"mailto:some.gmail@gmail.com\" class=\"mp_address_email\">some.gmail@gmail.com</a></span>",
"text": "some.gmail@gmail.com"
},
"messageId": "<sdf@gmail.com>"
}
describe('tests getAllMail function', () => {
before("Set configs and add mail", ()=>{
cy.setConnectionConfig(config)
})
it('returns parsed body', ()=>{
cy.getAllMail().then(mail=>{
expect(mail.length).to.eq(4)
mail.forEach(email=>{
expect(email[0].subject).to.contain("Hello World")
})
})
})
})
before("Set configs and add mail", ()=>{
cy.setConnectionConfig(config)
})
it('returns parsed body', ()=>{
cy.getEmailByIndex().then(email=>{
cy.pasteHtml(email.html)
cy.contains("Hello World").should("be.visible")
})
})
before("Set configs and add mail", ()=>{
cy.setConnectionConfig(config)
})
it('returns parsed body', ()=>{
cy.deleteAllMail().then(isDeleted=>{
cy.expect(isDeleted).to.eq(true)
})
})
before("Set configs and add mail", ()=>{
cy.setConnectionConfig(config)
})
it('returns parsed body', ()=>{
cy.deleteEmailByIndex(1).then(isDeleted=>{
cy.expect(isDeleted).to.eq(true)
})
})
Filip Cica
waitForEmail()
FAQs
Plugin for E2E email testing with cypress
The npm package cymap receives a total of 206 weekly downloads. As such, cymap popularity was classified as not popular.
We found that cymap demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.