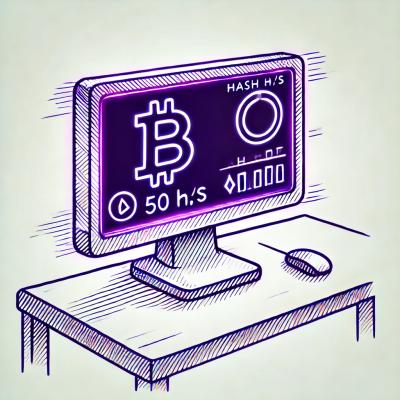
Security News
Research
Supply Chain Attack on Rspack npm Packages Injects Cryptojacking Malware
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
ds-jovo-platform-facebookmessenger
Advanced tools
To view this page on the Jovo website, visit https://www.jovo.tech/marketplace/jovo-platform-facebookmessenger
Learn more about the Facebook Messenger Platform and its platform-specific features that can be used with the Jovo Framework.
Install the module:
npm install jovo-platform-facebookmessenger --save
Import the installed module, initialize and add it to the app
object:
// @language=javascript
// src/app.js
const { FacebookMessenger } = require('jovo-platform-facebookmessenger');
app.use(new FacebookMessenger());
// @language=typescript
// src/app.ts
import { FacebookMessenger } from 'jovo-platform-facebookmessenger';
app.use(new FacebookMessenger());
Configure the module:
// @language=javascript
// src/app.js
module.exports = {
platform: {
FacebookMessenger: {
pageAccessToken: 'yourPageAccessToken',
verifyToken: 'yourVerifyToken',
locale: 'yourLocale'
}
}
};
// @language=typescript
// src/app.ts
export = {
platform: {
FacebookMessenger: {
pageAccessToken: 'yourPageAccessToken',
verifyToken: 'yourVerifyToken',
locale: 'yourLocale'
}
}
};
To set the greeting-text on startup you have to set the following configuration:
// @language=javascript
// src/config.js
module.exports = {
platform: {
FacebookMessenger: {
greeting: {
updateOnSetup: true,
data: [
{ locale: 'en-US', text: 'Get Started' },
{ locale: 'de-DE', text: 'Loslegen' }
]
}
}
}
};
// @language=typescript
// src/config.ts
export = {
platform: {
FacebookMessenger: {
greeting: {
updateOnSetup: true,
data: [
{ locale: 'en-US', text: 'Get Started' },
{ locale: 'de-DE', text: 'Loslegen' }
]
}
}
}
};
To set the launch-payload on startup you have to set the following configuration:
// @language=javascript
// src/config.js
module.exports = {
platform: {
FacebookMessenger: {
launch: {
updateOnSetup: true,
data: 'someLaunchPayload'
}
}
}
};
// @language=typescript
// src/config.ts
export = {
platform: {
FacebookMessenger: {
launch: {
updateOnSetup: true,
data: 'someLaunchPayload'
}
}
}
};
To set the persistent menu payload on startup you have to set the following configuration:
// @language=javascript
// src/config.js
module.exports = {
platform: {
FacebookMessenger: {
persistentMenu: {
updateOnSetup: true,
data: [{
locale:"default",
composer_input_disabled:false,
call_to_actions: [{
type:"postback",
title:"HelloWorld",
payload:"HelloWorldIntent"
}]
}]
}
}
}
};
// @language=typescript
// src/config.ts
export = {
platform: {
FacebookMessenger: {
persistentMenu: {
updateOnSetup: true,
data: [{
locale:"default",
composer_input_disabled:false,
call_to_actions: [{
type: PersistentMenuItemType.Postback,
title:"HelloWorld",
payload:"HelloWorldIntent"
}]
}]
}
}
}
};
By default, tell
and ask
generate a message that will be sent shortly before responding to the initial request.
As a consequence, this message will always be the last message sent.
If you do not like this behavior, you can disable it by setting the shouldIgnoreSynchronousResponse
-property to true
in the configuration:
// @language=javascript
// src/config.js
module.exports = {
platform: {
FacebookMessenger: {
shouldIgnoreSynchronousResponse: true
}
}
};
// @language=typescript
// src/config.ts
export = {
platform: {
FacebookMessenger: {
shouldIgnoreSynchronousResponse: true
}
}
};
You can access the messengerBot
object like this:
// @language=javascript
this.$messengerBot
// @language=typescript
this.$messengerBot!
The returned object will be an instance of MessengerBot
if the current request comes from Facebook Messenger. Otherwise undefined
will be returned.
These sections provide an overview of Facebook Messenger specific features for output. For the basic concept, take a look here: Docs: Basic Concepts > Output.
Facebook Messenger does not support reprompts. Any reprompt passed to ask
will be ignored.
Facebook Messenger allows sending multiple messages asynchronously.
All asynchronous methods for sending messages return a AxiosResponse
. It is recommended to wrap these call in a try-catch
-block to catch possible errors thrown by the API.
INFO: The message that results from calling
tell
orask
will be the last message sent! This behavior can be disabled, read more here.
You can read more about sending messages here.
The speech of tell
and ask
can be overwritten:
// @language=javascript
if (this.$messengerBot) this.$messengerBot.setText('someNewText');
// @language=typescript
this.$messengerBot?.setText('someNewText');
Additionally, quick-replies can be overwritten and added:
// @language=javascript
if (this.$messengerBot) {
this.$messengerBot.setQuickReplies([
new TextQuickReply('someQuickReplyText')
]);
this.$messengerBot.addQuickReply('test');
}
// @language=typescript
this.$messengerBot
?.setQuickReplies([new TextQuickReply('someQuickReplyText')])
.addQuickReply('test');
The following example shows how to send a text-message:
// @language=javascript
if (this.$messengerBot) await this.$messengerBot.showText({ text: 'text' });
// @language=typescript
await this.$messengerBot?.showText({ text: 'text' });
INFO: The message that was created by calling
tell
orask
will always be the last message to be displayed. Even if theshowText
-method was called after. Read more about disabling that behavior here.
When calling the text
-method an object with the following properties can be passed:
property | type | description |
---|---|---|
text | string | Required. The text that will be displayed |
quickReplies | Array<QuickReply | string> | Optional. Quick-Replies that will be shown below the text. |
messageType | MessageType | Optional. The type of the message. Defaults to MessageType.Response . |
Quick-replies can be sent by passing the quickReplies
property to the options of the showText
- or showAttachment
-method:
// @language=javascript
await this.$messengerBot.showText({
text: 'someText',
quickReplies: [
{ content_type: QuickReplyContentType.Text, title: 'someTitle' },
'Text-only-QuickReply'
]
});
// @language=typescript
await this.$messengerBot?.showText({
text: 'someText',
quickReplies: [
{ content_type: QuickReplyContentType.Text, title: 'someTitle' },
'Text-only-QuickReply'
]
});
Read more about quick-replies here.
In addition to manually creating a QuickReply
-object, the helper classes TextQuickReply
and BuiltInQuickReply
can be used.
INFO: When passing a string, a TextQuickReply will be created from that string.
TextQuickReply
can be used to create a QuickReply
-object that displays text.
// @language=javascript
new TextQuickReply('someTitle', 'somePayload', 'someImageUrl');
// @language=typescript
new TextQuickReply('someTitle', 'somePayload', 'someImageUrl');
BuiltInQuickReply
can be used to create QuickReply
-object that either displays the user's email or phone number and provides an easy to way for the user to reply with this information.
// @language=javascript
new BuiltInQuickReply(QuickReplyContentType.Email);
// @language=typescript
new BuiltInQuickReply(QuickReplyContentType.Email);
Read more about built-in quick-replies here and here.
Facebook Messenger allows sending attachments, which includes audio, videos, images and files.
// @language=javascript
if (this.$messengerBot)
await this.$messengerBot.showAttachment({
type: AttachmentType.File,
data: {
fileName: 'displayFileName',
path: 'localPathToFile'
}
});
// @language=typescript
await this.$messengerBot?.showAttachment({
type: AttachmentType.File,
data: {
fileName: 'displayFileName',
path: 'localPathToFile'
}
});
Read more about sending attachments here.
property | type | description |
---|---|---|
type | AttachmentType | Required. The type of the attachment. |
data | Buffer | string | number | Required. The data to be sent with the attachment. |
quickReplies | Array<QuickReply | string> | Optional. Quick-Replies that will be shown below the attachment. |
isReusable | boolean | Optional. Determines whether the resource is reusable. |
Actions allow controlling indicators for typing and read receipts.
The following actions are supported:
// @language=javascript
if (this.$messengerBot)
await this.$messengerBot.showAction(SenderActionType.TypingOn);
// @language=typescript
await this.$messengerBot?.showAction(SenderActionType.TypingOn);
There is also a helper method that sends the TypingOn and TypingOff action with a delay.
// @language=javascript
if (this.$messengerBot)
await this.$messengerBot.showTyping(5000); // Show typing bubble for 5 seconds.
// @language=typescript
await this.$messengerBot?.showTyping(SenderActionType.TypingOn); // Show typing bubble for 5 seconds.
Read more here.
Facebook Messenger allows sending templates.
Read more here.
The following example shows how to send a generic template:
// @language=javascript
if (this.$messengerBot)
await this.$messengerBot.showGenericTemplate({
elements: [
{
title: 'someTitle'
}
]
});
// @language=typescript
await this.$messengerBot?.showGenericTemplate({
elements: [
{
title: 'someTitle'
}
]
});
Read more here.
The following example shows how to send a button template:
// @language=javascript
if (this.$messengerBot)
await this.$messengerBot.showButtonTemplate({
text: 'someText',
buttons: [new PostbackButton('someTitle', 'somePayload')]
});
// @language=typescript
await this.$messengerBot?.showButtonTemplate({
text: 'someText',
buttons: [new PostbackButton('someTitle', 'somePayload')]
});
Read more here.
The following example shows how to send a media template:
// @language=javascript
if (this.$messengerBot)
await this.$messengerBot.showMediaTemplate({
elements: [
{
media_type: MediaType.Image,
url: 'someUrl',
buttons: []
}
]
});
// @language=typescript
await this.$messengerBot.showMediaTemplate({
elements: [
{
media_type: MediaType.Image,
url: 'someUrl',
buttons: []
}
]
});
Read more here.
The following example shows how to send a receipt template:
// @language=javascript
if (this.$messengerBot)
await this.$messengerBot.showReceiptTemplate({
recipient_name: 'someName',
order_number: 'someId',
currency: 'EUR',
payment_method: 'Visa',
summary: {
total_cost: 14.99
}
});
// @language=typescript
await this.$messengerBot?.showReceiptTemplate({
recipient_name: 'someName',
order_number: 'someId',
currency: 'EUR',
payment_method: 'Visa',
summary: {
total_cost: 14.99
}
});
Read more here.
The following example shows how to send an airline template:
// @language=javascript
if (this.$messengerBot)
await this.$messengerBot.showAirlineTemplate({
intro_message: 'someMessage',
locale: 'someLocale',
boarding_pass: [
{
passenger_name: 'someName',
pnr_number: 'somePassengerNumber',
logo_image_url: 'someUrl',
flight_info: {
flight_number: 'someFlightNumber',
departure_airport: {
terminal: 'someTerminal',
gate: 'someGate',
airport_code: 'someAirportCode',
city: 'someCity'
},
arrival_airport: {
airport_code: 'someAirportCode',
city: 'anotherCity'
},
flight_schedule: {
departure_time: 'someISOTimestamp'
}
}
}
]
});
// @language=typescript
await this.$messengerBot?.showAirlineTemplate({
intro_message: 'someMessage',
locale: 'someLocale',
boarding_pass: [
{
passenger_name: 'someName',
pnr_number: 'somePassengerNumber',
logo_image_url: 'someUrl',
flight_info: {
flight_number: 'someFlightNumber',
departure_airport: {
terminal: 'someTerminal',
gate: 'someGate',
airport_code: 'someAirportCode',
city: 'someCity'
},
arrival_airport: {
airport_code: 'someAirportCode',
city: 'anotherCity'
},
flight_schedule: {
departure_time: 'someISOTimestamp'
}
}
}
]
});
Read more here.
FAQs
Unknown package
The npm package ds-jovo-platform-facebookmessenger receives a total of 0 weekly downloads. As such, ds-jovo-platform-facebookmessenger popularity was classified as not popular.
We found that ds-jovo-platform-facebookmessenger demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.
Security News
Sonar’s acquisition of Tidelift highlights a growing industry shift toward sustainable open source funding, addressing maintainer burnout and critical software dependencies.