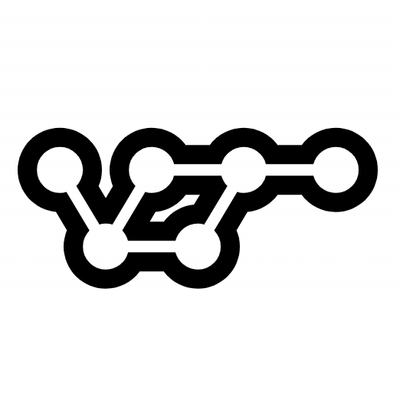
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
egg-template-helper
Advanced tools
install egg-init
globally
npm i egg-init -g
npx egg-init --type=ts projectName
cd projectName && npm i
npm run dev
egg-init --type simple projectName
cd projectName && npm i
npm run dev
For further details, please refer to https://github.com/eggjs/egg-init
npm install egg-template-helper -g
or
yarn global add egg-template-helper
$ eth -h
Usage: eth [commands] [options]
Options:
-v, --version output the version number
-h, --help output usage information
Commands:
new [name] [options] 新建egg文件
delete [name] [options] 删除egg文件
rename [name] [options] 重命名egg文件
eth new
create files by the [name] and a specified type.
name | type | description |
---|---|---|
category | string | new file category |
test | boolean | whether append test for controller / service / model |
Available category
default
: will create controller, service and model.controller
: will create a controller.service
: will create a service.model
: will create a model, the model depends on mongoose
. eth new user --category default
or
eth new user -c default
Actually the [name] parameter is a path relative to app/controller
/ app/service
/ app/model
In this example, it will create app/controller/user.ts
, app/model/user.ts
, app/service/user.ts
.
eth new permission/role --category default
or
eth new permission/role -c default
It will create app/controller/permission/role.ts
, app/model/permission/role.ts
, app/service/permission/role.ts
.
eth delete
delete files associated with the [name]
eth delete user
It will delete app/controller/user.ts
, app/model/user.ts
, app/service/user.ts
.
eth rename
name | type | description |
---|---|---|
newPath | string | new path |
rename files associated with the [name]
eth rename user -n masterUser
or
eth rename user -newPath masterUser
It will rename app/controller/user.ts
, app/model/user.ts
, app/service/user.ts
to app/controller/masterUser.ts
, app/model/masterUser.ts
, app/service/masterUser.ts
It will also change the file content!!!
Once user
renamed to masterUser
, the app/controller/masterUser.ts
content is changed.
import { Controller } from 'egg'
/**
* MasterUser Controller
*
* @export
* @class MasterUserController
* @extends {Controller}
*/
export default class MasterUserController extends Controller {
}
You can setup your own templates.
create an eth.config.json
file under the project root.
name | type | default | description |
---|---|---|---|
ext | string | ".ts" | file extenision, you can specify ext to ".js" if you use JavaScript to develop egg project |
templateDir | string | "" | custom template path, relative to the project root |
execEts | boolean | true | execute egg-ts-helper after command executed. It will take effect when ext is ".ts" |
renameContent | boolean | true | rewrite file content when executing rename command |
customData | object | {} | pass custom data to custom template when executing new command |
Let's customize a template and inject an author name by setting customData.
eth.config.json
{
"ext": ".ts",
"templateDir": "template",
"execEts": true,
"renameContent": false,
"customData": {
"Author": "Dada"
}
}
directory structure
egg-showcase
├── node_modules
└── app
├── controller
├── model
├── service
└── router.ts
├── template template directory
| ├── controller.test.tpl controller test template
| ├── controller.tpl controller template
| ├── model.tpl model template
| ├── service.test.tpl service testtemplate
└── └── service.tpl service template
import { Controller } from 'egg'
/**
* <%=pascalCaseName%> Controller
*
* @author <%=Author%>
* @export
* @class <%=pascalCaseName%>Controller
* @extends {Controller}
*/
export default class <%=pascalCaseName%>Controller extends Controller {
}
There are four built in variables: name
, pascalCaseName
, testName
, modelTestRootRelative
new
command eth new user -c default
The content in app/controller/user.ts
will like this:
import { Controller } from 'egg'
/**
* User Controller
*
* @author Dada
* @export
* @class UserController
* @extends {Controller}
*/
export default class UserController extends Controller {
}
FAQs
egg template helper
The npm package egg-template-helper receives a total of 0 weekly downloads. As such, egg-template-helper popularity was classified as not popular.
We found that egg-template-helper demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.